Mastering ParamConverters: JAX-RS Parameter Conversion Issues
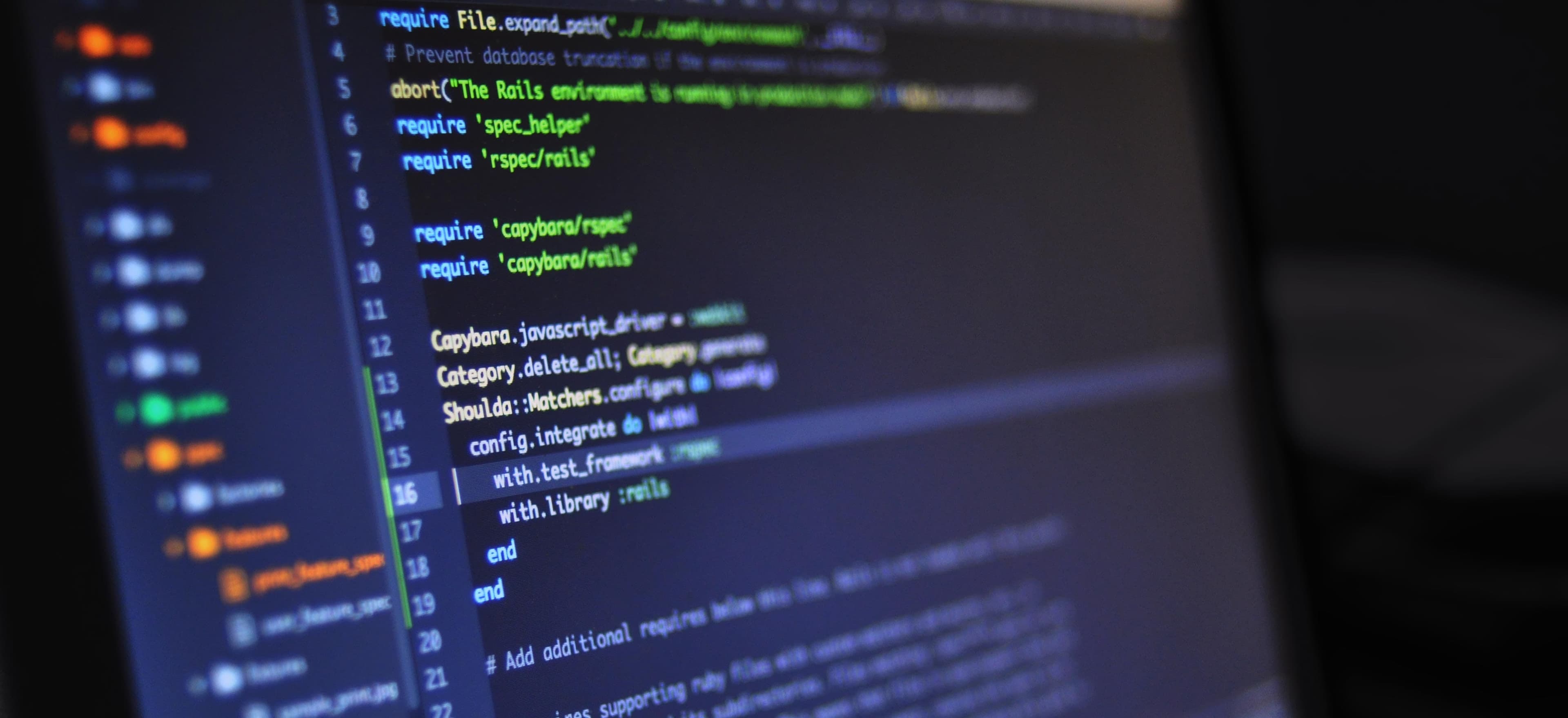
- Published on
Mastering ParamConverters: JAX-RS Parameter Conversion Issues
Java Application Programming Interfaces (APIs) have become an integral part of web development. In particular, Java EE's JAX-RS framework simplifies the creation of RESTful web services. With request parameters forming the backbone of any API, effective conversion of these parameters into appropriate Java data types is crucial. This is where ParamConverters come in, addressing common parameter conversion issues.
What is JAX-RS and ParamConverters?
JAX-RS (Java API for RESTful Web Services) is a set of APIs to create web services following REST architecture principles. It allows Java developers to build APIs that are easily consumable across various platforms.
Understanding ParamConverters
ParamConverters in JAX-RS are components that handle the conversion of request parameters into Java types. Each time an endpoint is hit, JAX-RS extracts parameters from the HTTP request and attempts to convert them into the types required by the resource methods. This conversion process, while usually automatic, can run into complications—especially when dealing with custom data types or complex structures.
To grasp how ParamConverters can be utilized effectively, consider the following sections.
Common Parameter Conversion Issues
-
Primitive Types and Null Handling: JAX-RS automatically handles conversion for primitive types like
int
,boolean
, etc. However, developers may faceNullPointerException
when an expected parameter is not passed. -
Complex Data Types: Representing complex objects or arrays through the URL can be tricky. For instance, how do you convert a comma-separated string into a proper list?
-
Custom Data Types: For custom classes, which JAX-RS doesn't know how to handle, developers must implement their own converters.
-
Type Safety: Conversions that don't respect the Java type system may lead to
ClassCastException
.
Let’s explore how to handle these issues through custom ParamConverters.
Implementing Custom ParamConverters
Custom ParamConverters are powerful tools to manage parameter conversion in a seamless manner. Below, we'll implement a custom converter for a complex data type: a User
class.
Step 1: Define the User Class
First, let's create a simple User
class:
public class User {
private String name;
private int age;
public User(String name, int age) {
this.name = name;
this.age = age;
}
// Getters and toString() method
public String getName() {
return name;
}
public int getAge() {
return age;
}
@Override
public String toString() {
return "User{name='" + name + "', age=" + age + '}';
}
}
Step 2: Create the ParamConverter
Now, let’s create a custom ParamConverter
for the User
class. The ParamConverter
interface has two important methods: fromString
for converting request parameters into an object and toString
for converting an object back into a string.
import javax.ws.rs.ext.ParamConverter;
import javax.ws.rs.ext.Provider;
@Provider
public class UserParamConverter implements ParamConverter<User> {
@Override
public User fromString(String value) {
String[] parts = value.split(",");
if (parts.length != 2) {
throw new IllegalArgumentException("Invalid User format. Expected format: name,age");
}
String name = parts[0];
int age = Integer.parseInt(parts[1].trim());
return new User(name, age);
}
@Override
public String toString(User user) {
return user.getName() + "," + user.getAge();
}
}
Explanation
-
@Provider Annotation: This tells JAX-RS that this class provides a conversion mechanism.
-
fromString Method: This method converts a
String
representation of aUser
into aUser
object. It uses the comma to split the parameters. An error is thrown if the format is unexpected. -
toString Method: This method offers a way to convert
User
objects back into theirString
representation.
Step 3: Register the ParamConverter
To use this ParamConverter
, it must be registered with your JAX-RS application. This can usually be done in your Application
class:
import javax.ws.rs.ApplicationPath;
import javax.ws.rs.core.Application;
import java.util.HashSet;
import java.util.Set;
@ApplicationPath("/api")
public class MyApplication extends Application {
@Override
public Set<Class<?>> getClasses() {
final Set<Class<?>> classes = new HashSet<>();
classes.add(UserResource.class); // Your REST resource
return classes;
}
}
Step 4: Create the REST Endpoint
Create a REST endpoint to utilize the User
parameter.
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.QueryParam;
import javax.ws.rs.core.Response;
@Path("/users")
public class UserResource {
@GET
public Response getUser(@QueryParam("user") User user) {
return Response.ok("Received user: " + user.toString()).build();
}
}
Explanation
- The
@QueryParam("user")
annotation tells JAX-RS to look for a query parameter calleduser
. - If the request is made like
/api/users?user=John Doe,30
, the custom ParamConverter will seamlessly convert this string into aUser
object.
Testing the Setup
After deploying your application, test the endpoint using a tool like Postman or directly via the browser:
http://localhost:8080/api/users?user=John Doe,30
If everything is correctly configured, you should receive a response confirming the received user data.
Closing Remarks
Mastering ParamConverters is critical for effective parameter handling when using JAX-RS. Custom ParamConverters not only simplify the conversion of complex data types but also significantly improve the robustness and maintainability of your code. Understanding the conversions happening behind the scenes can help developers avoid potential pitfalls with parameter handling.
For more information on JAX-RS and RESTful APIs, consider checking JAX-RS Official Documentation and exploring different JAX-RS capabilities.
As we continue to refine our knowledge of Java and its frameworks, keep experimenting with different ParamConverters and other JAX-RS features to enhance your applications further. Happy coding!
Checkout our other articles