Common Pitfalls When Getting Started with JAX-WS
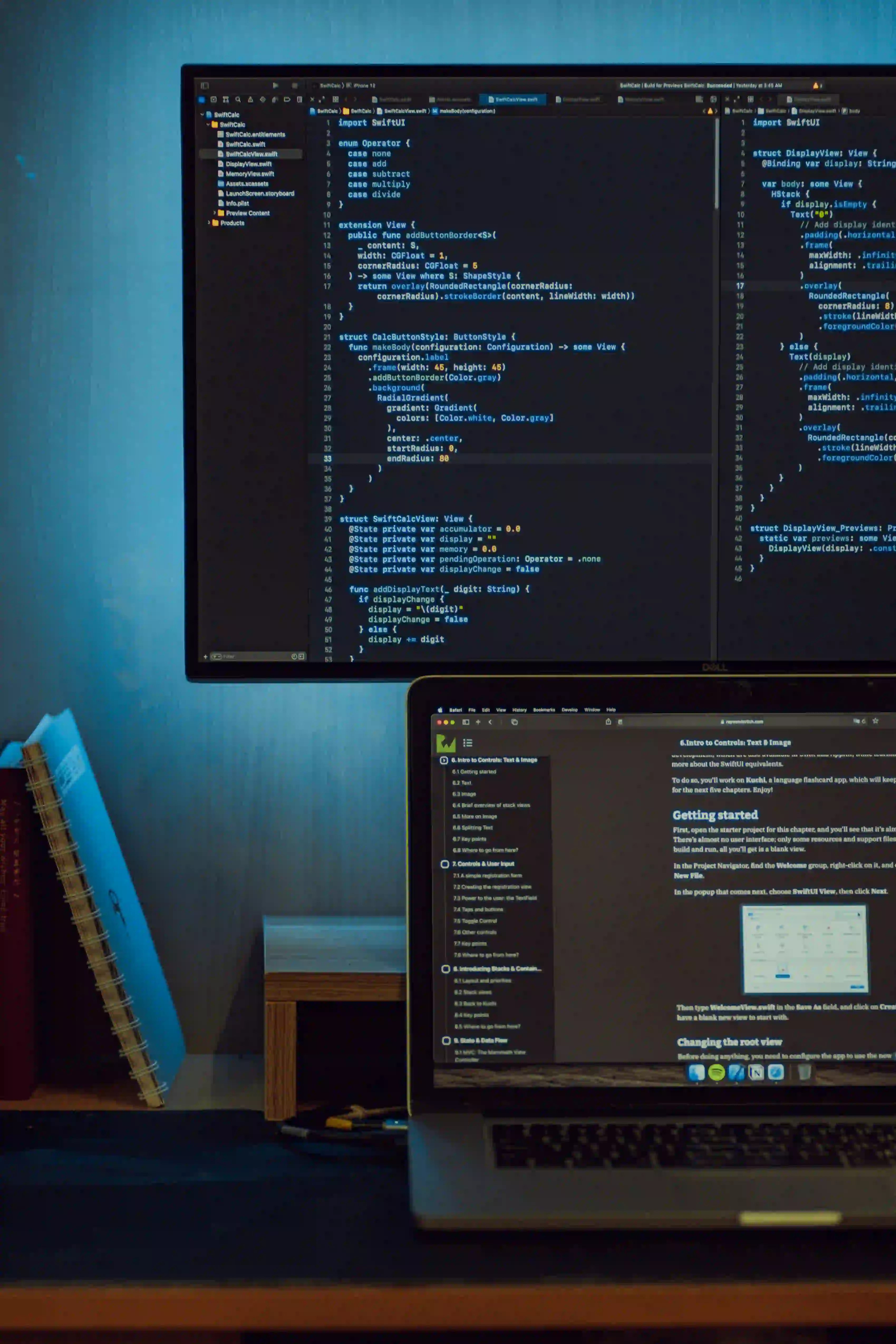
Common Pitfalls When Getting Started with JAX-WS
Java API for XML Web Services (JAX-WS) is a crucial part of Java EE that enables developers to build web services that communicate over the Internet via XML. While JAX-WS provides powerful tools, getting started can lead to several common pitfalls that can hinder development or lead to frustrating debugging sessions. In this blog post, we will delve into these pitfalls, offering insights and solutions to help you navigate the world of JAX-WS smoothly.
Understand the Basics of JAX-WS
Before diving into the pitfalls, it's essential to grasp the basics of JAX-WS. JAX-WS allows the creation of both SOAP and RESTful web services in Java. Understanding its architecture, including the role of service endpoints (SEI), service implementations, and the deployment descriptor, is crucial for effective use.
Why JAX-WS?
JAX-WS provides a standardized way to develop web services, which leads to increased interoperability across different systems. The API enables developers to expose business logic as web services and facilitates client-server communication with ease.
Common Pitfalls When Getting Started with JAX-WS
1. Misunderstanding Service Endpoints
A common confusion exists between Service Endpoint Interfaces (SEI) and service implementations. Many developers mistakenly think that the SEI is the implementation.
Example:
@WebService
public interface HelloWorld {
@WebMethod
String sayHello(String name);
}
In this snippet, HelloWorld
defines the SEI. The actual implementation should be in another class:
@WebService(endpointInterface = "com.example.HelloWorld")
public class HelloWorldImpl implements HelloWorld {
@Override
public String sayHello(String name) {
return "Hello, " + name + "!";
}
}
Why? It's vital to separate the interface from the implementation for better code organization and flexibility. This distinction aids in maintaining and scaling the application.
2. Ignoring Annotations
Annotations are at the core of JAX-WS, yet many new developers are unaware of their significance. Using the correct annotations, such as @WebService
, @WebMethod
, and @WebParam
, is essential for the service to function correctly.
Example:
@WebService
public class MathService {
@WebMethod
public int add(@WebParam(name = "num1") int num1, @WebParam(name = "num2") int num2) {
return num1 + num2;
}
}
Why? Annotations tell the JAX-WS runtime how to handle methods and classes as web service endpoints. Skipping or misusing them can lead to deployment errors or runtime exceptions.
3. Configuration Mistakes
JAX-WS services require proper deployment descriptors, particularly when hosted on application servers. Failing to configure these files accurately can result in issues that are challenging to diagnose.
Solution: Always double-check your web.xml
or sun-jaxws.xml
files for correct mapping. For example:
<servlet>
<servlet-name>HelloWorldService</servlet-name>
<servlet-class>com.sun.xml.ws.transport.http.servlet.WSServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>HelloWorldService</servlet-name>
<url-pattern>/HelloWorldService</url-pattern>
</servlet-mapping>
Why? Proper configuration aligns the server with your service, ensuring that requests are routed correctly.
4. Faulty WSDL Generation
WSDL (Web Services Description Language) is a core component of SOAP web services. Many developers overlook proper WSDL generation, leading to client incompatibility.
Solution:
Always verify the generated WSDL by accessing it via a browser at the endpoint. If the WSDL is malformed or missing, recheck your service definitions and annotations.
Why? The WSDL defines how clients can interact with your service. If it is incorrect, clients won’t be able to consume your web service.
5. Error Handling Oversights
Robust web service design includes how to handle errors gracefully. Many developers ignore SOAP faults and rely on generic error messages.
Example:
@WebService
public class UserService {
@WebMethod
public User getUser(int id) throws UserNotFoundException {
User user = userDatabase.findUserById(id);
if (user == null) {
throw new UserNotFoundException("User not found for id: " + id);
}
return user;
}
}
Why? Exposing meaningful errors to clients helps in debugging and improves the overall service experience. Ensure custom exceptions are properly annotated for SOAP fault generation.
6. Ignoring Security
When deploying web services, security should never be an afterthought. Many developers treat JAX-WS services as open endpoints, exposing sensitive data.
Solution: Implement WS-Security standards or consider HTTPS to secure communication.
Why? With the rise of cyber threats, ensuring that your web services are secured is paramount. Information should be protected during transit, and sensitive data must be encrypted.
7. Not Using Client Libraries
The JAX-WS API provides tools to generate client stubs from WSDL files. However, many new developers attempt to consume services without utilizing these resources.
Example:
Generating a client can be done using the following command:
wsimport -keep -s src -d bin http://localhost:8080/HelloWorldService?wsdl
Why? Using generated client stubs simplifies the API calls and handles the underlying complexity of the SOAP protocol. It leads to cleaner and fewer error-prone code.
8. Performance Issues
As with any technology, performance can degrade if not managed correctly. One common pitfall is failing to cache WSDL or improperly managing connection pools.
Solution: Configure your server for optimal performance and analyze your code for bottlenecks.
Why? Proper performance management allows your service to handle multiple requests efficiently, improving user experiences and reducing server load.
9. Lack of Testing
Many developers overlook automated testing for web services. Failing to establish tests can lead to unexpected behavior post-deployment.
Solution: Implement tools like JUnit and Mockito to create unit tests for your JAX-WS services.
Example:
import static org.mockito.Mockito.*;
import org.junit.Test;
public class HelloWorldServiceTest {
@Test
public void testSayHello() {
HelloWorldImpl helloWorld = new HelloWorldImpl();
String response = helloWorld.sayHello("World");
assertEquals("Hello, World!", response);
}
}
Why? Automated tests help catch issues early, ensuring your service behaves as expected across different scenarios.
Key Takeaways
JAX-WS offers powerful capabilities for building web services, yet many developers face pitfalls when starting. By understanding service endpoint structures, adhering to proper configuration, handling errors graciously, and ensuring security, you can navigate these anxieties with ease.
As the world leans towards a digital-first approach, mastering JAX-WS will empower you to provide efficient, reliable, and secure services. With careful attention to detail and a focus on best practices, you can avoid the common pitfalls that newcomers often face.
For further reading about JAX-WS, you can check out the official Oracle documentation.
Remember, the web service landscape is rapidly evolving—keeping yourself informed is vital for your success as a developer. Happy coding!