Mastering Cache Eviction: Common Pitfalls in Spring Caching
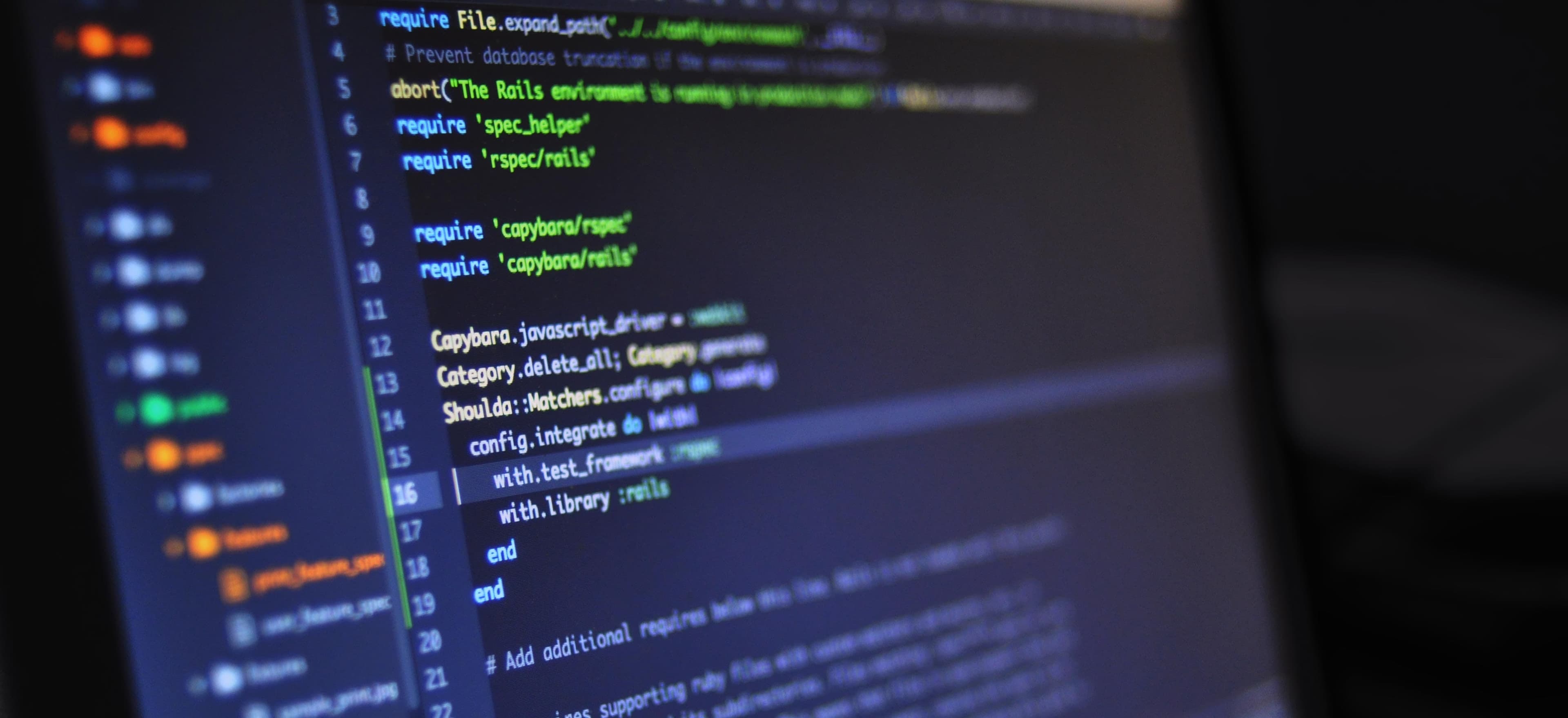
- Published on
Mastering Cache Eviction: Common Pitfalls in Spring Caching
Caching is an essential technique used in modern software development to improve performance and reduce latency. In Spring applications, caching can greatly enhance the responsiveness of your application by storing frequently accessed data in memory. However, cache eviction presents a myriad of challenges that developers must navigate to ensure smooth performance. In this blog post, we will delve into common pitfalls in Spring caching, focusing on cache eviction strategies and best practices.
Understanding Spring Caching
Before exploring cache eviction, it’s important to first understand what caching means in the context of Spring applications. Caching allows you to store the results of expensive method calls temporarily. When a method is called, Spring checks if the return value is already available in the cache. If it is, the cached value is returned instantly without executing the method again, thus saving time and CPU resources.
Spring provides several annotations to manage caching effectively:
@EnableCaching
: Enables caching in your Spring configuration.@Cacheable
: Indicates that the result of a method should be cached.@CachePut
: Updates an existing cache entry.@CacheEvict
: Removes one or more entries from the cache when certain conditions are met.
Quick Example
Let’s look at a simple example demonstrating how to use caching in a Spring service.
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Service;
@Service
public class MathService {
@Cacheable("squares")
public int square(int number) {
try {
Thread.sleep(3000); // Simulate an expensive operation
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return number * number;
}
}
In this example, the square
method simulates a resource-intensive calculation. The result of each call will be cached for future use.
Cache Eviction Strategies
Cache eviction is the process of removing cached entries based on specific criteria. This aspect of caching is critical, as stale data can lead to incorrect application behavior. Here are common pitfalls in cache eviction along with tips on avoiding them.
1. Not Using Cache Eviction Properly
Some developers mistakenly think they don’t need cache eviction. This is a dangerous assumption, especially when working with mutable data sources.
Example Pitfall
Imagine a scenario where your application's state changes based on user actions. If you don't evict outdated cache entries when data is modified, users may see stale information.
Solution
Always ensure you mark data as "stale" and evict it upon updates. Below is an updated version of our MathService
class using the @CacheEvict
annotation:
import org.springframework.cache.annotation.CacheEvict;
import org.springframework.stereotype.Service;
@Service
public class MathService {
@Cacheable("squares")
public int square(int number) {
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return number * number;
}
@CacheEvict(value = "squares", allEntries = true)
public void updateCache() {
// Logic to update the cache entries
}
}
In this case, whenever you want to update the cache, calling the updateCache
method evicts all cache entries associated with the "squares" cache.
2. Over-relying on Annotations
Spring’s annotations provide a quick way to manage caching, but over-relying on them without understanding their implications can lead to pitfalls.
Example Pitfall
Using allEntries = true
on the @CacheEvict
annotation means you will remove everything from the cache. If your cache contains other relevant entries, this can lead to performance degradation.
Solution
Be specific in your cache eviction strategy. Instead of evicting all entries, target specific keys. Here’s how to do that:
@CacheEvict(value = "squares", key = "#number")
public void evictSquare(int number) {
// Only evicting the entry for the provided number
}
This targeted approach prevents the unnecessary removal of unrelated cache entries.
3. Ignoring Cache Configuration
Starting with the default cache configuration might lead to inefficient cache usage. Developers sometimes overlook the need to define cache expiration policies or maximum size limits.
Example Pitfall
If your cache never expires or has no size limit, it can grow uncontrollably, leading to increased memory usage.
Solution
Configure your cache properties to include maximum sizes and expiration times. Here’s an example of how to configure Ehcache as an external cache provider in application.properties
:
spring.cache.ehcache.config=classpath:ehcache.xml
And in your ehcache.xml
:
<ehcache>
<diskStore path="java.io.tmpdir" />
<defaultCache
maxEntriesLocalHeap="10000"
eternal="false"
timeToIdleSeconds="120"
timeToLiveSeconds="300"
overflowToDisk="true" />
<cache name="squares" maxEntriesLocalHeap="100" timeToIdleSeconds="300" />
</ehcache>
Setting these parameters ensures that your application manages memory efficiently.
4. Ignoring Asynchronous Callbacks
Another common pitfall is failing to recognize the importance of asynchronous callbacks in managing cache eviction.
Example Pitfall
When another thread modifies the underlying data, a cache entry might not be evicted. This situation can amplify the problem of stale data.
Solution
Consider using asynchronous event listeners for cache eviction or employing other reactive mechanisms, like Spring WebFlux, for managing cache consistency.
Monitoring Cache Performance
Once you implement caching, it is essential to monitor its performance regularly. Tools like Spring Boot Actuator can provide insights into the cache, including the number of hits, misses, and eviction counts.
Adding Actuator Dependency
In your pom.xml
, include the Spring Actuator dependency:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Enabling Caching Metrics
To enable caching metrics, add the following to your application.properties
:
management.metrics.cache.cache-names=squares
management.metrics.cache.squares.enabled=true
You can then access the metrics via the Actuator endpoints, providing a powerful mechanism to evaluate caching effectiveness.
Closing Remarks
Mastering cache eviction in Spring caching involves understanding the intricacies and potential pitfalls involved in caching mechanisms. Always remember to:
- Properly utilize cache eviction annotations.
- Avoid over-relying on default cache settings.
- Configure caching suited to your application needs.
- Manage cache eviction for concurrency.
- Regularly monitor cache performance.
By following the best practices outlined in this post, you can ensure that your application remains efficient, responsive, and robust. For additional insights, consider visiting the Spring Caching Documentation.
Happy coding!