Handling Future Timeouts in Google Guava: A Guide
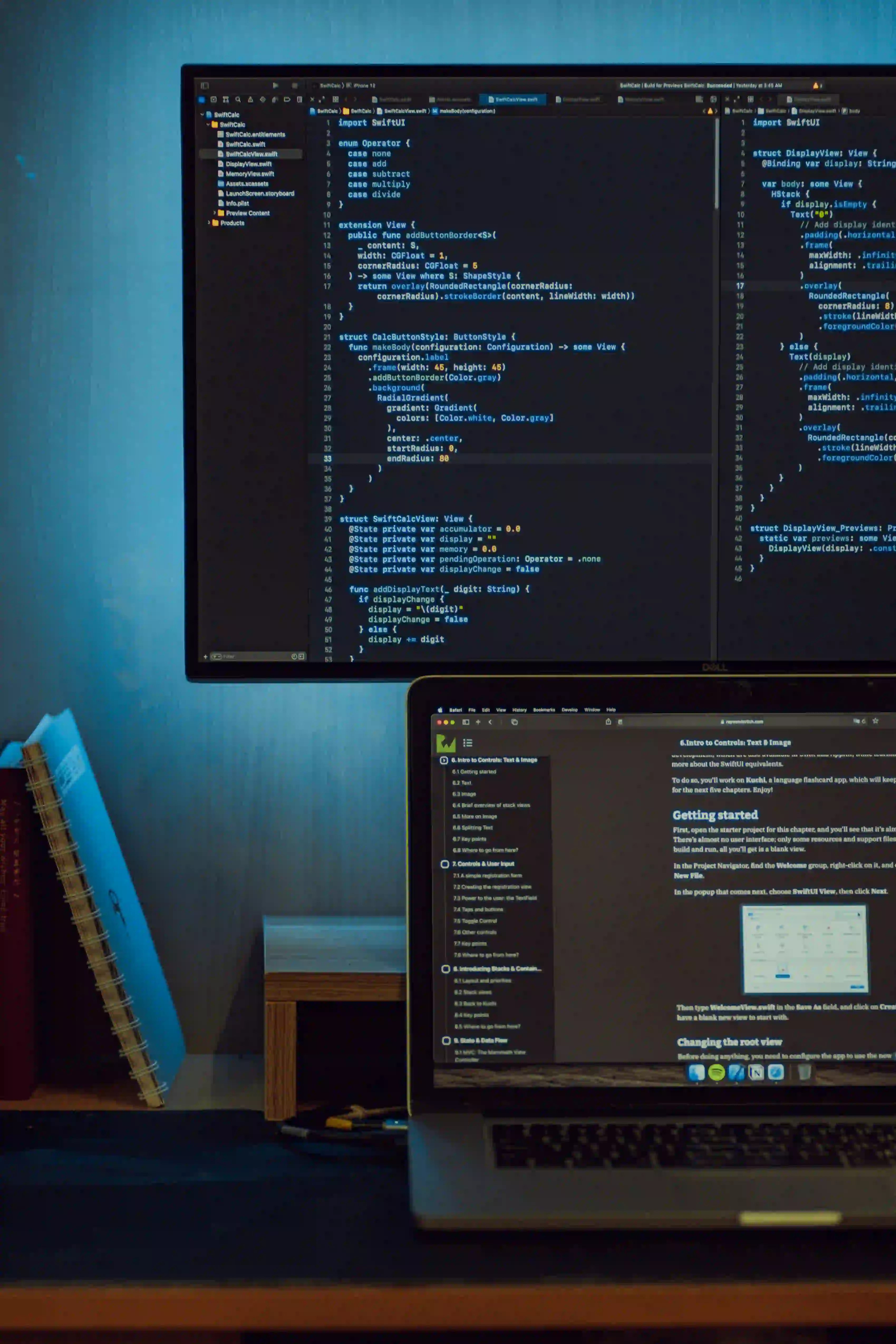
Handling Future Timeouts in Google Guava: A Guide
In the world of concurrent programming, timeouts play a crucial role. They help prevent applications from hanging indefinitely while waiting for a task to complete. Java’s Future
interface is typically used for asynchronous computation. However, managing timeouts effectively can be challenging, especially in complex applications. In this post, we will delve into handling future timeouts seamlessly using Google Guava, a powerful library that provides simple ways to extend Java's concurrency framework.
What is Google Guava?
Google Guava is an open-source set of core libraries for Java, developed by Google. It offers numerous utilities that can enhance productivity, including collections, caching, primitives support, concurrency libraries, and more. Notably, Guava's handling of futures and timeouts makes asynchronous processing much simpler and more intuitive.
Understanding the Basics
Before diving into implementation, let’s briefly look at the Future
interface and how it operates.
When a task is executed asynchronously, it returns a Future
object:
Future<String> future = executorService.submit(() -> {
// Simulate a long-running task
Thread.sleep(2000);
return "Task completed!";
});
In this example, a future represents a task that will complete after 2 seconds. However, without a timeout, you risk blocking indefinitely if something goes wrong.
Why Use a Timeout?
Using a timeout allows us to prevent long waits for a result. If the task doesn’t complete within the specified time, we can handle it gracefully, perhaps logging the error or providing feedback to the user. Guava simplifies this with its Futures
utility class.
Implementing Timeouts with Guava
Guava provides a helper method called Futures.withTimeout()
. It allows you to specify a timeout for your futures easily. Here’s how you can implement it.
Setting Up Your Environment
To use Guava, you'll first need to include it in your project. If you're using Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>31.1-jre</version>
</dependency>
Make sure to replace the version number with the latest one available.
Example Code Snippet
Here’s a straightforward example to illustrate handling future timeouts with Guava’s Futures.
import com.google.common.util.concurrent.*;
import java.util.concurrent.*;
public class FutureTimeoutExample {
public static void main(String[] args) {
ExecutorService executorService = Executors.newSingleThreadExecutor();
// Create a Callable task
Callable<String> longRunningTask = () -> {
Thread.sleep(5000); // Simulate a long-running task
return "Task completed!";
};
// Submit the task to the executor
ListenableFuture<String> future = MoreExecutors.listeningDecorator(executorService).submit(longRunningTask);
try {
// Set a timeout of 2 seconds
String result = Futures.getUnchecked(Futures.withTimeout(future, 2, TimeUnit.SECONDS));
System.out.println(result);
} catch (TimeoutException e) {
System.out.println("The task timed out.");
} catch (ExecutionException e) {
System.out.println("Execution failed: " + e.getCause());
} finally {
executorService.shutdown();
}
}
}
Explanation of the Code
- Executor Service: We create a single-threaded executor to which we will submit our task.
- Callable Task: The
longRunningTask
simulates a task that sleeps for 5 seconds. - ListenableFuture: We use
MoreExecutors.listeningDecorator()
to convert the standardFuture
intoListenableFuture
, which provides additional features such as callbacks. - Timeout Handling: Using
Futures.withTimeout()
, we specify that we expect a result within 2 seconds. If the task does not complete in this time, it will throw aTimeoutException
. - Error Handling: We manage
TimeoutException
separately, and also deal with any potentialExecutionException
that might arise if the task fails for other reasons.
Benefits of Using Guava for Future Timeouts
- Simplification: Guava’s utility methods simplify working with complex concurrency tasks.
- Clearer Intent: Using
withTimeout
clearly conveys the intention of handling task completion within a specific timeframe. - Robust Error Handling: The structured approach to handling exceptions enables you to cater to various scenarios effectively.
Best Practices
When working with futures and timeouts in Guava, consider the following best practices:
-
Choose Appropriate Timeout Values: Analyze your application's requirements. Too short of a timeout can result in frequent timeouts, whereas too long can negate the advantage of using a timeout.
-
Use ListenableFutures: When using Guava, favor
ListenableFuture
overFuture
for its enhanced capabilities, including support for asynchronous listeners. -
Graceful Shutdown: Always remember to shut down your executor service gracefully to free up resources.
-
Follow Up with Polling: If your task has dependencies, consider polling for results rather than relying solely on immediate returns.
Additional Resources
For a deeper dive into concurrency in Java and how Google Guava fits into the landscape, consider visiting:
Lessons Learned
Handling future timeouts effectively can dramatically improve the reliability of your Java applications. By utilizing Guava’s Futures
utility, developers can manage asynchronous tasks with ease and clarity. The examples and practices shared in this guide provide a solid foundation for implementing timeout handling in your own projects. Embrace Guava and empower your Java applications with better concurrency management!