Common OAuth Scenarios That Trip Up Spring Security Users
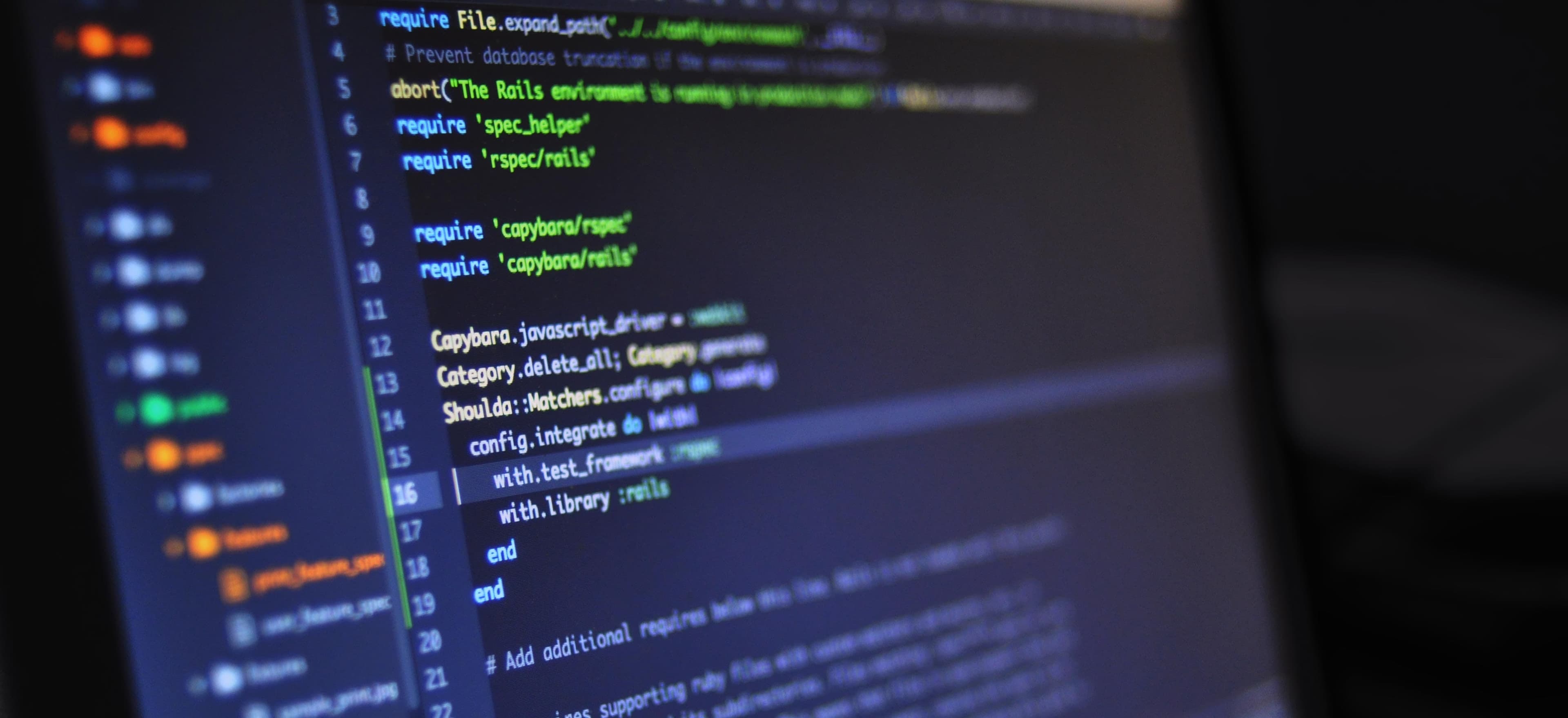
- Published on
Common OAuth Scenarios That Trip Up Spring Security Users
OAuth is a powerful protocol widely used for delegated authorization, allowing users to share specific bits of their data with an application while keeping their usernames and passwords hidden. While Spring Security provides a robust framework for implementing OAuth 2.0 in Java applications, developers often encounter stumbling blocks along the way. In this blog post, we will discuss common pitfalls and scenarios that can trip up Spring Security users when implementing OAuth, along with best practices and code examples.
What is OAuth?
Before diving into the common scenarios, it is essential to understand what OAuth is and how it works. OAuth 2.0 is an authorization framework that enables applications (clients) to obtain limited access to user accounts on an HTTP service, such as Facebook, Google, or GitHub, without exposing the user's password.
In essence, OAuth consists of four main roles:
- Resource Owner - The user who owns the data.
- Client - The application requesting access to the user's data.
- Resource Server - The server hosting the protected resources.
- Authorization Server - The server issuing access tokens to the client.
For an in-depth understanding of OAuth concepts, you can refer to the official OAuth 2.0 specification.
Common OAuth Scenarios
1. Misconfigured Redirect URIs
The Problem
One of the most common mistakes developers encounter when implementing OAuth is misconfigured redirect URIs. The redirect URI is crucial for receiving the authorization code after the user grants or denies access. A mismatch between the registered redirect URI and the one sent in requests can lead to authorization failures.
The Solution
Ensure that the redirect URI registered with the authorization server exactly matches the one configured in your application. Here's how to set it up in your Spring application:
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/", "/oauth2/**").permitAll()
.anyRequest().authenticated()
.and()
.oauth2Login()
.loginPage("/login")
.defaultSuccessUrl("/home", true);
}
}
In this snippet, ensure that the redirect URI for your application matches the one specified in the OAuth provider's console.
2. Incorrect Scopes
The Problem
Another scenario that can trip up developers is specifying the wrong scopes when requesting access. Scopes limit the access that the client has to the resource owner's data. Wrongly configured or missing scopes can lead to "permission denied" errors.
The Solution
Carefully read the documentation of the OAuth provider you are working with to understand the available scopes.
Here's how to set the scopes in your application:
@Bean
public ClientRegistrationRepository clientRegistrationRepository() {
return new InMemoryClientRegistrationRepository(this.googleClientRegistration());
}
private ClientRegistration googleClientRegistration() {
return ClientRegistration.withRegistrationId("google")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.scope("openid", "profile", "email") // Correctly set scopes here
.authorizationUri("https://accounts.google.com/o/oauth2/auth")
.tokenUri("https://oauth2.googleapis.com/token")
.userInfoUri("https://www.googleapis.com/oauth2/v3/userinfo")
.redirectUriTemplate("{baseUrl}/login/oauth2/code/{registrationId}")
.build();
}
3. Failing to Handle Token Expiry
The Problem
OAuth tokens have lifespan limits. Access tokens typically expire after a short duration, while refresh tokens can last longer. Failing to handle token expiry correctly can cause your application to stop functioning as intended when the token is no longer valid.
The Solution
To handle token expiry effectively, implement token refresh logic. While Spring Security OAuth provides built-in support for this, developers must ensure their application requests refresh tokens when initially obtaining access.
Here’s an example of how to implement the refresh token logic:
RestTemplate restTemplate = new RestTemplate();
String refreshToken = "your-refresh-token"; // Get refresh token from your store
String clientId = "your-client-id";
String clientSecret = "your-client-secret";
String refreshTokenUri = "https://oauth2.googleapis.com/token";
MultiValueMap<String, String> request = new LinkedMultiValueMap<>();
request.add("client_id", clientId);
request.add("client_secret", clientSecret);
request.add("refresh_token", refreshToken);
request.add("grant_type", "refresh_token");
ResponseEntity<Map> response = restTemplate.postForEntity(
refreshTokenUri,
request,
Map.class
);
// Extract new access token
String newAccessToken = (String) response.getBody().get("access_token");
By implementing proper token management, your application can gracefully recover from token expiry scenarios.
4. Scattered User Context Management
The Problem
Managing user sessions across various components of your application can become complicated, especially in microservices architectures. It’s common for developers to overlook maintaining user context, leading to inconsistent states and improper permissions.
The Solution
Use a centralized session management system. Tools like Spring Session or dedicated identity management solutions can help manage user sessions effectively.
Here’s how you can set up Spring Session:
<dependency>
<groupId>org.springframework.session</groupId>
<artifactId>spring-session-data-redis</artifactId>
</dependency>
With Spring Session, you can establish a distributed session management system, enhancing consistency across interactions in your microservices.
5. Ignoring Security Best Practices
The Problem
One of the last common pitfalls is ignoring security best practices. OAuth authentication flows can become vulnerable if not implemented correctly, risking user data exposure.
The Solution
Regularly update dependencies, perform security audits, and employ additional security measures like:
- Enabling HTTPs to encrypt traffic.
- Using state parameters to prevent CSRF attacks.
- Validating token signatures.
Implementing security measures in your Spring Security configuration can go a long way in ensuring the protection of user data:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/", "/oauth2/**").permitAll()
.anyRequest().authenticated()
.and()
.oauth2Login()
.defaultSuccessUrl("/home", true)
.and()
.csrf().csrfTokenRepository(CookieCsrfTokenRepository.withHttpOnlyFalse()); // CSRF Protection
}
Final Considerations
Implementing OAuth with Spring Security can be straightforward and efficient, if you are aware of the common pitfalls. From ensuring redirect URIs are correctly set to managing token expiry effectively, understanding these scenarios is key to a robust OAuth implementation.
If you want to delve deeper into OAuth and Spring Security, I recommend checking out Spring Security's official guide for comprehensive details and best practices.
Incorporating these insights and following best practices will not only enhance your application's security but also provide a seamless experience for your users. Remember, being proactive about potential issues is far better than being reactive!