Mastering Asynchronous Programming in Android Apps
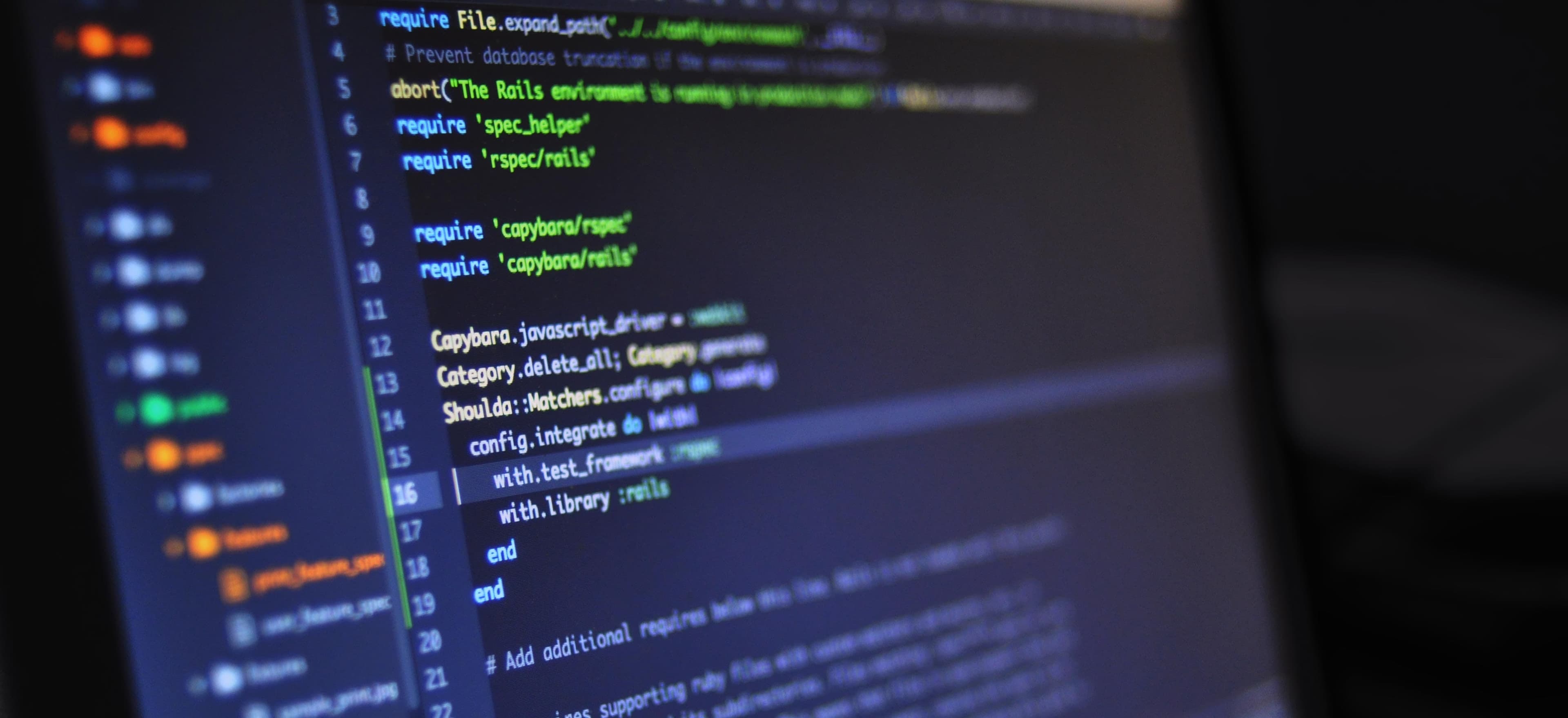
- Published on
Mastering Asynchronous Programming in Android Apps
As mobile developers, we are often faced with the challenge of creating seamless user experiences. Users dislike delays, and anything that slows down the UI can lead to frustration. One of the most effective ways to tackle this is through asynchronous programming. In this post, we'll explore the various ways to implement asynchronous programming in Android apps while ensuring your app remains responsive and user-friendly.
Understanding Asynchronous Programming
Asynchronous programming allows a program to perform tasks without blocking the main execution thread. In Android, the main thread is responsible for updating the UI. If you execute a long-running operation, such as a network request or database query, on the main thread, it will cause the UI to freeze, leading to a poor user experience.
There's an array of tools and libraries specifically aimed to manage asynchronous operations in Android, including:
- AsyncTask (deprecated in Android 11)
- Handlers and Runnable
- Executors
- Kotlin coroutines
- RxJava
In the following sections, we will discuss these options while providing code snippets to illustrate their use.
Kotlin Coroutines: The Future of Asynchronous Programming
Kotlin Coroutines is a powerful feature that has simplified asynchronous programming in Android. This feature allows developers to write asynchronous code in a sequential manner, making it more maintainable and easier to read.
Getting Started with Coroutines
To start using coroutines, you need to add the Kotlin Coroutine dependency to your project:
// Add this to your build.gradle (Module) file
dependencies {
implementation 'org.jetbrains.kotlinx:kotlinx-coroutines-core:1.5.0'
implementation 'org.jetbrains.kotlinx:kotlinx-coroutines-android:1.5.0'
}
Example: Fetching Data Asynchronously
Here’s how to make a network request using coroutines:
import kotlinx.coroutines.*
import retrofit2.Retrofit
import retrofit2.http.GET
// Define a Retrofit service
interface ApiService {
@GET("data/endpoint")
suspend fun fetchData(): Response<Data>
}
fun fetchData() {
CoroutineScope(Dispatchers.IO).launch {
try {
val response = service.fetchData()
withContext(Dispatchers.Main) {
if (response.isSuccessful) {
updateUI(response.body())
} else {
showError(response.errorBody())
}
}
} catch (e: Exception) {
withContext(Dispatchers.Main) {
showError(e.message)
}
}
}
}
Code Explanation
- CoroutineScope (Dispatchers.IO): This specifies that the coroutine will run in the IO thread, which is optimized for IO operations like network calls and database interactions.
- launch: This function is used to start a new coroutine.
- suspend: This keyword indicates that the function can be paused and resumed while waiting for the result, allowing other tasks to run in the meantime.
- withContext(Dispatchers.Main): This switches the context back to the main thread to update the UI once data is retrieved.
For more information on Kotlin Coroutines, check the official documentation.
Using Executors for Background Work
Executors are part of the Java Concurrency framework and can be a good fit if you need simpler thread management.
import java.util.concurrent.Executors
// Create a single-threaded executor
val executor = Executors.newSingleThreadExecutor()
executor.execute {
// Background work here
val data = fetchDataFromDatabase()
// Update UI on the main thread (ensure to run this on UI thread)
runOnUiThread {
updateUI(data)
}
}
Code Explanation
- Executors.newSingleThreadExecutor(): Creates an executor that uses a single worker thread to execute tasks. This is useful for serializing tasks.
- execute(): Submits a task for execution. The task runs asynchronously, ensuring that the main thread remains unblocked.
- runOnUiThread(): This utility method allows you to switch back to the UI thread for UI updates.
Closing Remarks: Choosing the Right Tool for Asynchronous Programming
In this blog post, we covered various methods for asynchronous programming in Android, especially focusing on Kotlin Coroutines. While there are different ways to handle asynchronous tasks, using coroutines is recommended for its simplicity and scalability.
If you want to dive deeper into Android development or stay updated with the latest tools, consider following resources like the Android Developer's Guide or Kotlin Documentation.
To summarize:
- Utilize Kotlin Coroutines for clean and efficient asynchronous code.
- Use Executors when working with Java and needing basic thread management.
- Avoid using deprecated methods like AsyncTask to ensure the future-proofing of your code.
By mastering asynchronous programming, not only will you enhance the performance of your Android apps, but you'll also provide a significantly better user experience. Happy coding!