Choosing the Right RxJava Operator for Async Data Processing
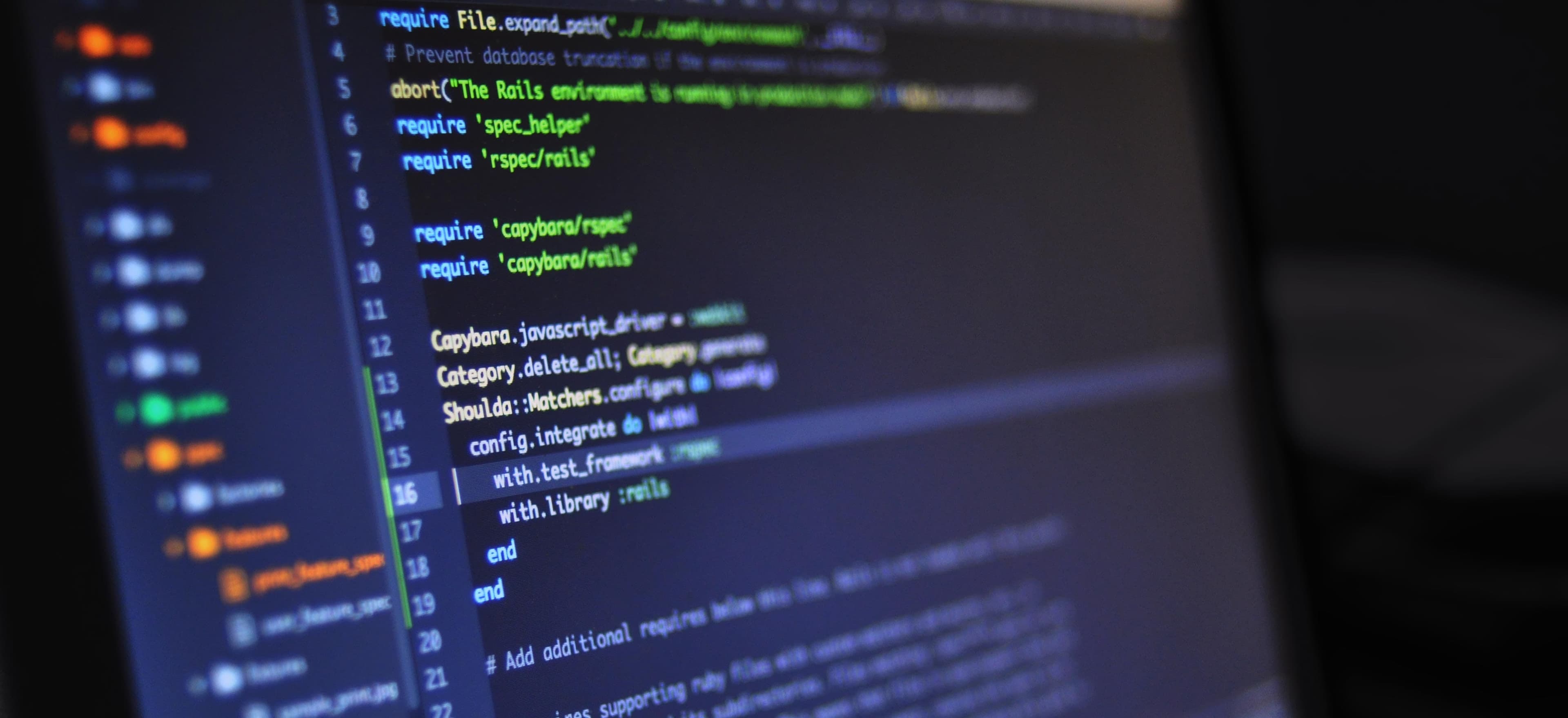
- Published on
Choosing the Right RxJava Operator for Async Data Processing
Asynchronous data processing is a crucial component of modern software development. With the rise of event-driven architectures and the need for responsive user interfaces, handling asynchronous operations efficiently is a top priority for many developers. In Java, one popular tool for managing asynchronous operations is RxJava, a library for composing asynchronous and event-based programs using observable sequences.
When working with RxJava, choosing the right operator for processing asynchronous data is key to writing efficient and maintainable code. In this article, we will explore some common scenarios for asynchronous data processing and discuss the RxJava operators that best suit each use case.
Filtering Data with RxJava
One common task when working with asynchronous data is filtering out unwanted elements from a stream. RxJava provides a variety of operators for this purpose, such as filter
, take
, and skip
.
Example:
Observable<Integer> dataStream = Observable.just(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
dataStream
.filter(value -> value % 2 == 0) // Keep only even numbers
.subscribe(System.out::println);
In this example, the filter
operator is used to keep only the even numbers from the data stream.
Transforming Data with RxJava
Another common use case for asynchronous data processing is transforming the elements in a stream based on certain criteria. RxJava offers operators like map
, flatMap
, and scan
for these scenarios.
Example:
Observable<String> stringStream = Observable.just("Rx", "is", "awesome");
stringStream
.map(String::toUpperCase) // Convert each string to uppercase
.subscribe(System.out::println);
Here, the map
operator is used to transform each string to uppercase before it's emitted downstream.
Combining Data with RxJava
There are also situations where you need to combine multiple sources of asynchronous data into a single stream. RxJava provides operators such as merge
, zip
, and concat
for this purpose.
Example:
Observable<String> source1 = Observable.just("Hello", "RxJava");
Observable<String> source2 = Observable.just("is", "awesome");
Observable.zip(source1, source2, (s1, s2) -> s1 + " " + s2) // Combine elements from both sources
.subscribe(System.out::println);
In this example, the zip
operator is used to combine elements from two sources into a single string before emitting it downstream.
Handling Errors with RxJava
Error handling is a crucial aspect of asynchronous data processing. RxJava provides operators like onErrorResumeNext
, retry
, and onErrorReturn
for dealing with errors gracefully.
Example:
Observable<String> dataSource = Observable.error(new RuntimeException("Data source failed"));
dataSource
.onErrorResumeNext(Observable.just("Default", "data")) // Fallback to a default data source
.subscribe(System.out::println, Throwable::printStackTrace);
In this example, the onErrorResumeNext
operator is used to provide a fallback data source in case the original source encounters an error.
Managing Backpressure with RxJava
When dealing with asynchronous data sources that produce events at a high rate, it's essential to handle backpressure to prevent overwhelming the downstream consumers. RxJava offers operators like onBackpressureBuffer
, onBackpressureDrop
, and onBackpressureLatest
to manage backpressure.
Example:
Flowable.range(1, 1000000)
.onBackpressureBuffer(1000) // Buffer up to 1000 items
.observeOn(Schedulers.io())
.subscribe(System.out::println);
In this example, the onBackpressureBuffer
operator is used to buffer incoming items and limit the rate of emission to downstream consumers.
Key Takeaways
When it comes to asynchronous data processing in Java, RxJava provides a powerful set of operators for handling a wide range of scenarios. By choosing the right operator for each use case, developers can ensure that their asynchronous code is both efficient and maintainable.
Understanding the various RxJava operators and their use cases is essential for leveraging the full potential of reactive programming in Java. Whether it's filtering, transforming, combining, error handling, or backpressure management, RxJava has a solution for every asynchronous data processing challenge.
By mastering these operators and applying them thoughtfully, developers can write code that is not only responsive and resilient but also easier to reason about and maintain in the long run.
To delve deeper into RxJava and its operators, check out the official RxJava documentation and the RxJava GitHub repository. Happy coding!