Optimizing Visibility in Weave and Docker Deployments
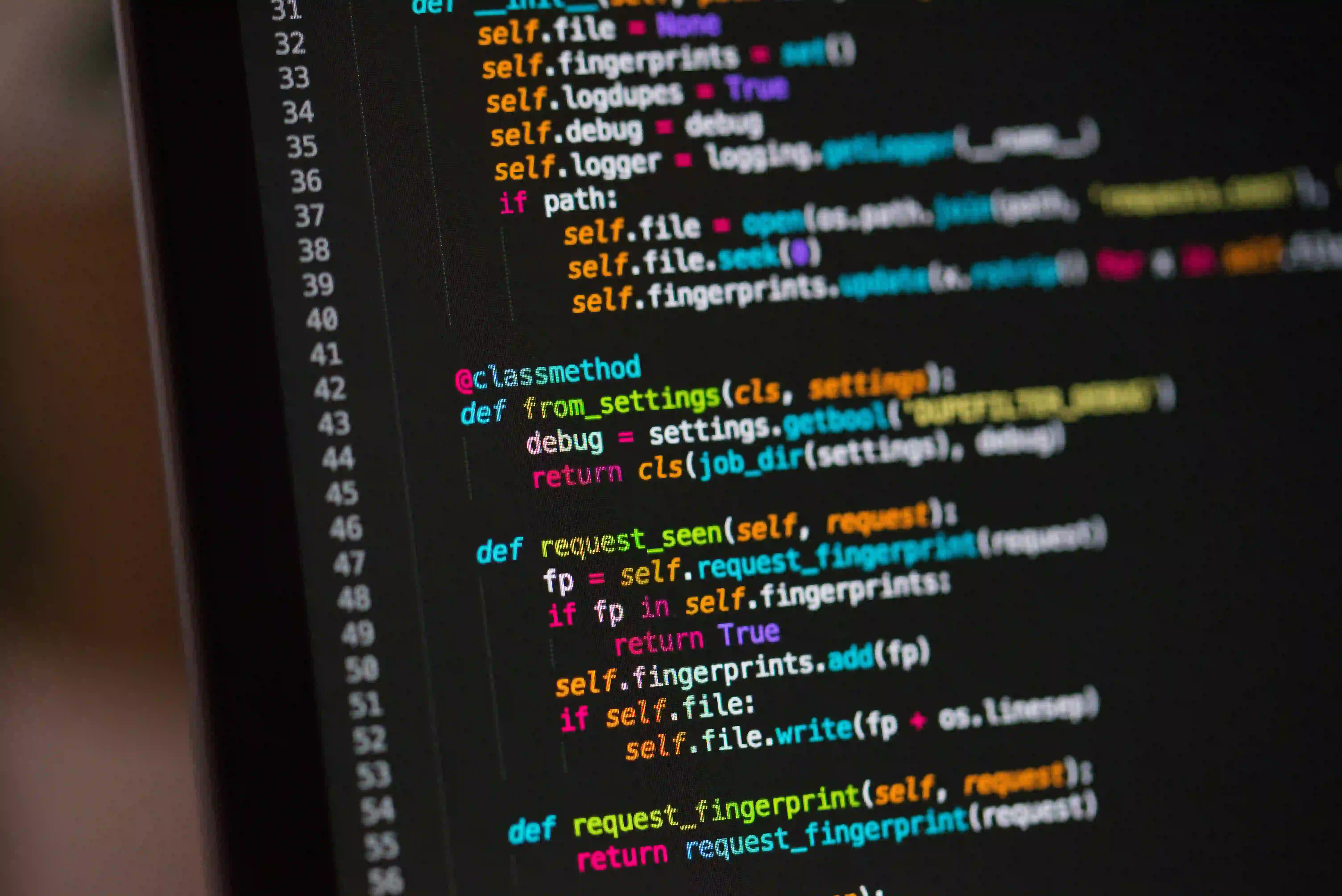
Best Practices for Optimizing Java Applications in Weave and Docker Deployments
In the world of modern software development, containerization has become a widely adopted practice. Docker, being a leading container platform, provides a consistent environment for applications to run in any environment - from the developer's laptop to a testing stage and then into production.
However, optimizing Java applications within the Docker environment can be a challenging task. In this post, we'll explore some best practices for maximizing visibility and performance when deploying Java applications in Weave and Docker. We'll cover tips for efficient resource utilization, monitoring, and logging to ensure your Java applications run smoothly in these containerized environments.
Tip 1: Asynchronous Logging
In Java applications, logging is an essential part of monitoring and troubleshooting. However, traditional logging mechanisms can impact performance, especially in a containerized environment where resources are constrained.
Using asynchronous logging libraries like Log4j 2 or LMAX Disruptor can significantly improve application performance by offloading logging tasks to background threads. This prevents the logging mechanism from blocking the main application thread, allowing it to focus on critical tasks.
// Using Log4j 2 for asynchronous logging
Logger logger = LogManager.getLogger(MyClass.class);
logger.info("This is an asynchronous log message");
Tip 2: JVM Heap Configuration
When running Java applications in Docker containers, it's crucial to optimize the JVM heap settings to make efficient use of resources. By default, Java applications allocate a significant amount of memory to the heap, which may not be ideal in a containerized environment.
Setting the appropriate -Xmx
and -Xms
values according to the container's resource constraints can prevent memory overallocation and improve overall performance.
# Example JVM heap settings in Dockerfile
ENV JAVA_OPTS="-Xms256m -Xmx512m"
Tip 3: Utilize Health Checks
Weave and Docker provide health check capabilities to monitor the status of containerized applications. Integrating health checks into your Java applications allows the container orchestrator to assess their health and take appropriate actions in case of failures.
By implementing custom health checks within your Java code and exposing endpoints for health status, you enable Weave and Docker to ensure high availability and reliability of your applications.
// Example implementation of a custom health check endpoint
@GetMapping("/health")
public ResponseEntity<String> checkHealth() {
// Implement custom health checks here
if (isHealthy) {
return ResponseEntity.ok("Healthy");
} else {
return ResponseEntity.status(HttpStatus.SERVICE_UNAVAILABLE).body("Unhealthy");
}
}
Tip 4: Container Resource Limits
In a shared containerized environment, it's essential to define resource limits for your Java containers to prevent them from monopolizing system resources. Weave and Docker allow you to specify CPU and memory limits for each container, ensuring fair resource allocation across the environment.
# Example Docker Compose service configuration with resource limits
services:
my-java-app:
image: my-java-app:latest
deploy:
resources:
limits:
cpus: '0.5'
memory: 512M
Tip 5: Monitoring with Prometheus and Grafana
To gain visibility into the performance of your Java applications in Weave and Docker, integrating monitoring tools like Prometheus and Grafana can provide valuable insights. These tools allow you to collect metrics, visualize performance data, and set up alerting for potential issues.
By instrumenting your Java code with Prometheus client libraries and creating dashboards in Grafana, you can proactively monitor the health and performance of your applications.
// Using Prometheus Java client for instrumentation
// Register custom metrics and collect data for monitoring
To Wrap Things Up
Optimizing Java applications in Weave and Docker deployments requires careful consideration of resource utilization, logging, monitoring, and health checks. By following these best practices, you can ensure that your Java applications run efficiently and reliably within containerized environments.
Remember to continually evaluate and adapt your deployment strategies as your application evolves, and leverage the rich ecosystem of tools and practices within the Docker and Weave ecosystem to maximize the visibility and performance of your Java applications.
Want to dive deeper into Docker and Kubernetes? Check out this Docker Deep Dive course. For more insights on Java application performance optimizations, refer to this article.