Handling Dynamic Dialogs with Functional Programming
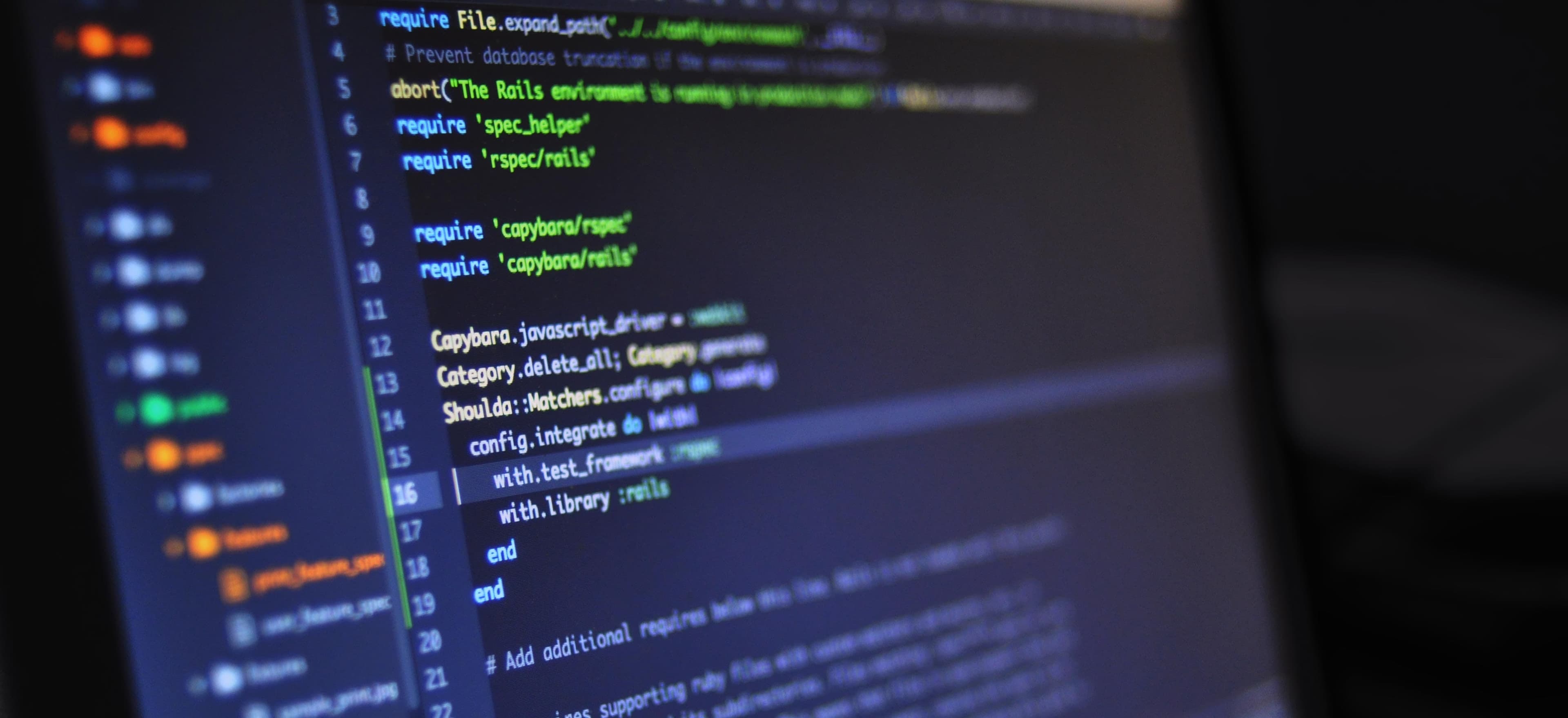
- Published on
A Guide to Handling Dynamic Dialogs with Functional Programming in Java
Dynamic dialogs play a crucial role in the user experience of modern applications. These dialogs adapt to changes in the application's state and user interactions, providing a more responsive and intuitive interface. In this guide, we'll explore how to handle dynamic dialogs using functional programming in Java. Leveraging functional programming principles can simplify the management of dynamic dialogs, making the code more modular, composable, and maintainable.
Why Functional Programming?
Functional programming promotes writing code in a declarative and expressive manner, focusing on the evaluation of functions and immutable data. This paradigm encourages the use of higher-order functions, which can be particularly useful for managing dynamic dialogs. By using functions as first-class citizens, we can create elegant and reusable solutions for building and handling dynamic dialogs.
Using Functional Interfaces
Java 8 introduced functional interfaces, which are interfaces with a single abstract method. These interfaces can be used to represent functions, making them ideal for modeling dialog behaviors in a functional programming style. Let's consider a scenario where we need to create a dynamic dialog that prompts the user for input. We can define a functional interface to handle the dialog behavior:
@FunctionalInterface
interface DialogHandler {
void handleDialog();
}
In this example, DialogHandler
represents a function that takes no arguments and returns void, effectively encapsulating the behavior of the dialog.
Composing Dialog Behavior
Functional programming enables us to compose complex behaviors from simpler functions. We can use higher-order functions to combine individual dialog behaviors into more sophisticated interactions. This approach promotes code reuse and modular design. For instance, we can define a higher-order function to compose multiple dialog handlers into a sequential workflow:
public class DialogComposer {
public static DialogHandler compose(DialogHandler... handlers) {
return () -> {
for (DialogHandler handler : handlers) {
handler.handleDialog();
}
};
}
}
Here, the compose
method takes an array of DialogHandler
instances and returns a new DialogHandler
that sequentially executes each handler's behavior. This allows us to build complex dialog interactions from smaller, reusable components.
Leveraging Lambda Expressions
Lambda expressions in Java enable concise representation of anonymous functions, aligning with the functional programming paradigm. Utilizing lambda expressions can make our code more expressive and succinct, especially when defining dialog behaviors. Consider the following example of using lambda expressions to create dialog handlers:
DialogHandler greetingDialog = () -> System.out.println("Hello, welcome to the application!");
DialogHandler userInputDialog = () -> {
// Logic to prompt the user for input
System.out.println("Please enter your name: ");
// Additional input handling logic
};
// Compose the dialog handlers
DialogHandler combinedDialog = DialogComposer.compose(greetingDialog, userInputDialog);
In this example, we define greetingDialog
and userInputDialog
using lambda expressions, encapsulating the specific behaviors of each dialog. We then compose these individual dialog handlers using the DialogComposer
, demonstrating the power of lambda expressions in creating modular and composable code for dynamic dialogs.
Handling Dynamic State
Dynamic dialogs often rely on the application's state to determine their behavior. Functional programming provides elegant ways to manage state using immutable data and pure functions. By embracing immutability and pure functions, we can ensure that our dialog handling logic remains predictable and free from side effects.
Let's consider a scenario where the visibility of a dialog depends on the user's authentication status. We can represent the dialog's visibility as a function of the application's state using a predicate:
@FunctionalInterface
interface VisibilityPredicate {
boolean isDialogVisible();
}
Here, VisibilityPredicate
defines a function that returns a boolean, indicating whether the dialog should be visible based on the application's state.
We can then use this predicate to conditionally execute the dialog behavior:
public class ConditionalDialogHandler {
public static DialogHandler createConditionalDialog(
VisibilityPredicate visibilityPredicate, DialogHandler dialogHandler) {
return () -> {
if (visibilityPredicate.isDialogVisible()) {
dialogHandler.handleDialog();
}
};
}
}
In this example, the createConditionalDialog
method takes a VisibilityPredicate
and a DialogHandler
, returning a new DialogHandler
that executes the provided dialog only when the visibility predicate is true. This approach allows us to handle dynamic dialog visibility based on the application's state using functional programming principles.
Final Considerations
Dynamic dialogs are essential components of modern applications, and managing their behavior can become complex as applications grow in size and complexity. By leveraging functional programming concepts such as functional interfaces, higher-order functions, lambda expressions, and immutable data, we can simplify the management of dynamic dialogs in Java. These principles promote modularity, reusability, and maintainability, resulting in more robust and expressive code for handling dynamic dialogs.
Incorporating functional programming techniques into dialog handling not only improves the codebase but also enhances the overall user experience by enabling responsive and adaptive dialog interactions within the application.
Functional programming provides a powerful paradigm for managing dynamic user interfaces, and its principles can be applied to a wide range of interactive components beyond dialogs, making it a valuable approach for developers seeking to create flexible and scalable applications.
By embracing functional programming, developers can build more resilient and maintainable solutions for handling dynamic dialogs, ultimately leading to more robust and user-friendly applications.
To further explore the power of functional programming in Java, consider learning more about Java streams and how they can be used to process and manipulate data in a functional style. The Java Streams API documentation provides valuable insights into leveraging functional programming to work with collections and data streams effectively.
In summary, leveraging functional programming for handling dynamic dialogs in Java offers a compelling approach to creating responsive, adaptable, and maintainable user interfaces, further enriching the user experience and the overall quality of software applications.