Navigating the Complexities of Jakarta EE's Java SE Level
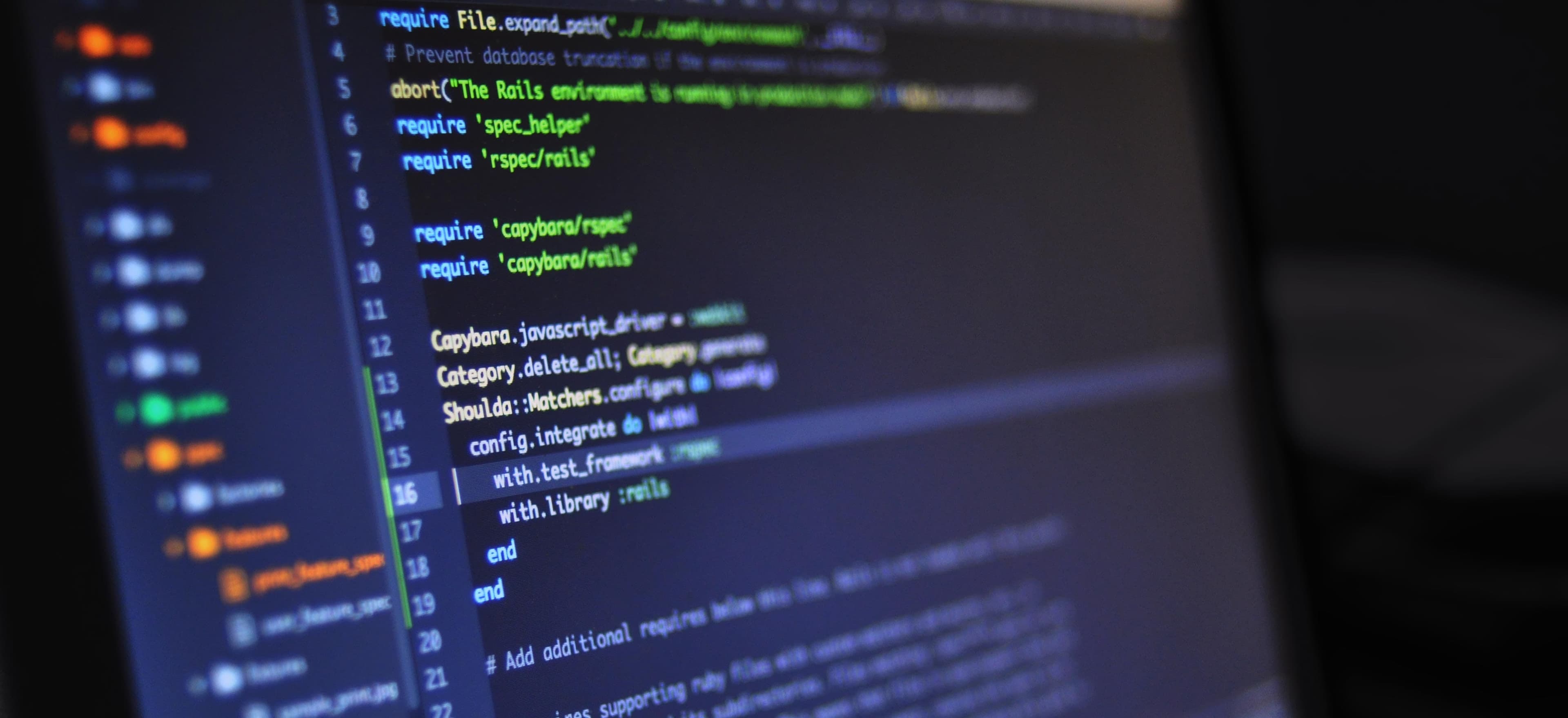
- Published on
Understanding Jakarta EE's Java SE Level
When it comes to Jakarta EE, understanding the Java SE level is crucial. Jakarta EE, formerly known as Java EE, offers a powerful platform for building enterprise-level applications. At its core, Jakarta EE is built on top of Java SE, which provides the foundation for the platform. In this article, we will explore the intricacies of Jakarta EE's Java SE level and how it impacts enterprise application development.
What is Jakarta EE?
Jakarta EE is a collection of technologies and APIs for the Java platform designed to support enterprise applications. It provides a set of specifications and APIs that simplify the process of building enterprise applications, making them portable, scalable, and secure.
The Relationship Between Jakarta EE and Java SE
Java Platform, Standard Edition (Java SE) serves as the foundation for Jakarta EE. This means that all the capabilities and features provided by Java SE are inherited by Jakarta EE applications. Java SE provides the basic libraries and APIs that are essential for any Java application, including those built with Jakarta EE.
Key Aspects of Jakarta EE's Java SE Level
1. Language Features
Java SE provides the language features that form the basis of Jakarta EE development. This includes the syntax, data types, control structures, and other fundamental aspects of the Java programming language.
2. Core Libraries
The core libraries of Java SE, such as java.lang
, java.util
, and java.io
, are fundamental to Jakarta EE development. These libraries provide essential utilities and data structures that are used extensively in enterprise applications.
3. Threading and Concurrency
Java SE's support for threading and concurrency directly benefits Jakarta EE applications, enabling them to handle multiple tasks efficiently and take advantage of modern multi-core processors.
4. Security
Security is a critical aspect of enterprise applications. Java SE's security features, including the Java Authentication and Authorization Service (JAAS) and the Java Cryptography Architecture (JCA), form the backbone of security in Jakarta EE applications.
5. Networking
Networking capabilities provided by Java SE enable Jakarta EE applications to communicate over various protocols, such as HTTP, HTTPS, and TCP/IP, facilitating interactions with clients and other services.
6. File I/O
File handling and I/O operations are essential in enterprise applications. Jakarta EE leverages Java SE's rich set of I/O APIs for reading from and writing to files, streams, and other I/O resources.
Code Examples
Let's dive into some code examples to illustrate the importance of Java SE in Jakarta EE development.
Example 1: Utilizing Java SE's Collections Framework in Jakarta EE
import java.util.List;
public class EmployeeService {
private List<Employee> employees;
public EmployeeService(List<Employee> employees) {
this.employees = employees;
}
public List<Employee> getEmployees() {
return employees;
}
}
In this example, the Jakarta EE EmployeeService
class leverages Java SE's List
interface from the java.util
package to manage a collection of employees.
Example 2: Implementing Concurrent Processing in Jakarta EE
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class TaskProcessor {
private ExecutorService executor = Executors.newFixedThreadPool(10);
public void processTasks(List<Runnable> tasks) {
for (Runnable task : tasks) {
executor.submit(task);
}
}
}
Here, the Jakarta EE TaskProcessor
class uses Java SE's ExecutorService
and Executors
to enable concurrent processing of tasks within an enterprise application.
Wrapping Up
In conclusion, understanding Jakarta EE's Java SE level is fundamental to developing robust and efficient enterprise applications. By leveraging the rich features and capabilities provided by Java SE, Jakarta EE empowers developers to build scalable, secure, and portable enterprise solutions. As you delve deeper into Jakarta EE development, remember that a strong grasp of Java SE is the cornerstone of your success.
To further explore Jakarta EE and Java SE, refer to the official documentation for Jakarta EE and Java SE.
In your journey with Jakarta EE, embrace the power of Java SE, and let it propel your enterprise applications to new heights.
Checkout our other articles