Inheritance and Polymorphism: Problems of Independent Implementation
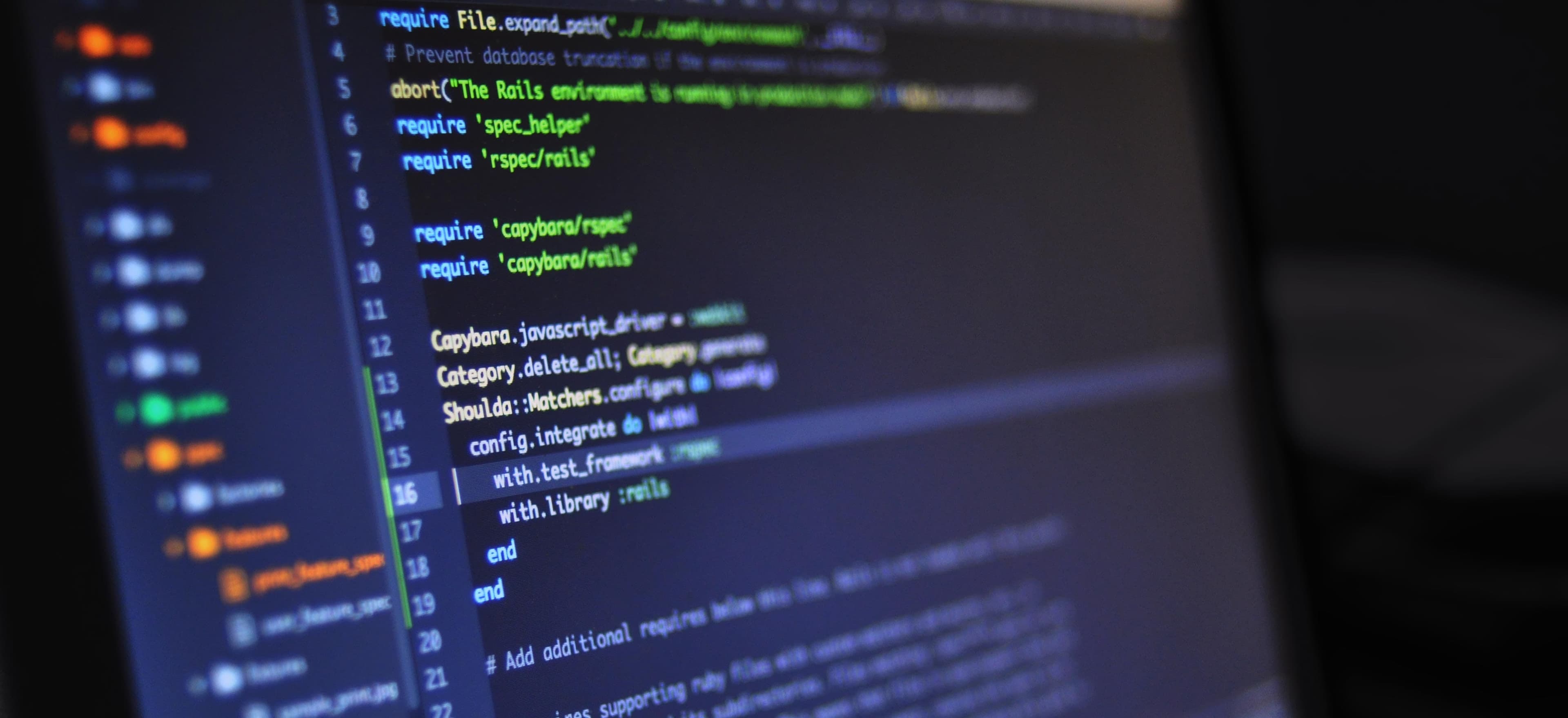
- Published on
The Problems of Independent Implementation: Inheritance and Polymorphism
Inheritance and polymorphism are fundamental concepts in object-oriented programming. They empower developers to create relationships between classes and enable code reuse. However, independent implementation of these concepts can lead to several problems.
The Scenario
Suppose we have a superclass Shape
with subclasses Circle
, Square
, and Triangle
. Each subclass implements a method calculateArea()
to compute its respective area. Additionally, we have a ShapeCalculator
class that takes an array of Shape
objects and calculates the total area.
The Implementation
Let's first examine the independent implementation of the calculateArea()
method in each subclass:
class Shape {
// other properties and methods
public double calculateArea() {
return 0.0; // Default implementation
}
}
class Circle extends Shape {
private double radius;
public double calculateArea() {
return Math.PI * radius * radius; // Specific to circle
}
}
class Square extends Shape {
private double side;
public double calculateArea() {
return side * side; // Specific to square
}
}
class Triangle extends Shape {
private double base;
private double height;
public double calculateArea() {
return 0.5 * base * height; // Specific to triangle
}
}
Each subclass implements its own calculateArea()
method tailored to its specific shape.
The Problem
Code Duplication
The calculateArea()
method is duplicated across the subclasses, violating the DRY (Don't Repeat Yourself) principle.
Limited Reusability
If we need to calculate the total perimeter instead of the total area, we would have to create a new method in the Shape
class and override it in each subclass, resulting in redundant code.
The Solution: Polymorphism
Leveraging Polymorphism
By using polymorphism, we can address the issues of code duplication and limited reusability. We can define the calculateArea()
method as an abstract method in the Shape
class and override it in each subclass. This enforces the specific implementation of calculateArea()
for each shape.
The Revised Implementation
Let's refactor the code to utilize polymorphism:
abstract class Shape {
// other properties and methods
public abstract double calculateArea(); // Abstract method
}
class Circle extends Shape {
private double radius;
@Override
public double calculateArea() {
return Math.PI * radius * radius; // Specific to circle
}
}
class Square extends Shape {
private double side;
@Override
public double calculateArea() {
return side * side; // Specific to square
}
}
class Triangle extends Shape {
private double base;
private double height;
@Override
public double calculateArea() {
return 0.5 * base * height; // Specific to triangle
}
}
By making calculateArea()
an abstract method in the Shape
class, we ensure that each subclass provides its own implementation.
The Advantages
Code Reusability
We can now create a generic method in the ShapeCalculator
class to calculate the total area by iterating through an array of Shape
objects without worrying about the specific shape implementations. This enhances reusability and maintains the DRY principle.
Extensibility
If we decide to add a new shape, such as Rectangle
, we can simply create a new subclass of Shape
and implement the calculateArea()
method without modifying existing classes. This demonstrates the extensibility achieved through polymorphism.
The Bottom Line
Independent implementation of inheritance and polymorphism can lead to code duplication and limited reusability. By leveraging polymorphism, we can address these issues and create a more maintainable and extensible codebase. Embracing the principles of object-oriented programming, such as abstraction and inheritance, enables us to build robust and scalable software systems.
In conclusion, embracing polymorphism ensures a flexible, maintainable, and scalable codebase, thereby enhancing the efficiency and reusability of the code. By leveraging the power of abstraction, inheritance, and polymorphism, we can create a more maintainable and extensible codebase. This approach exemplifies the best practices in object-oriented programming, leading to robust and scalable software systems.
Interested in diving deeper into the concept of polymorphism? Check out this article that provides further insights into how polymorphism works in Java.
In conclusion, embracing polymorphism ensures a flexible, maintainable, and scalable codebase, thereby enhancing the efficiency and reusability of the code. By leveraging the power of abstraction, inheritance, and polymorphism, we can create a more maintainable and extensible codebase. This approach exemplifies the best practices in object-oriented programming, leading to robust and scalable software systems.