Building Resilient Camel Applications with Hystrix DSL
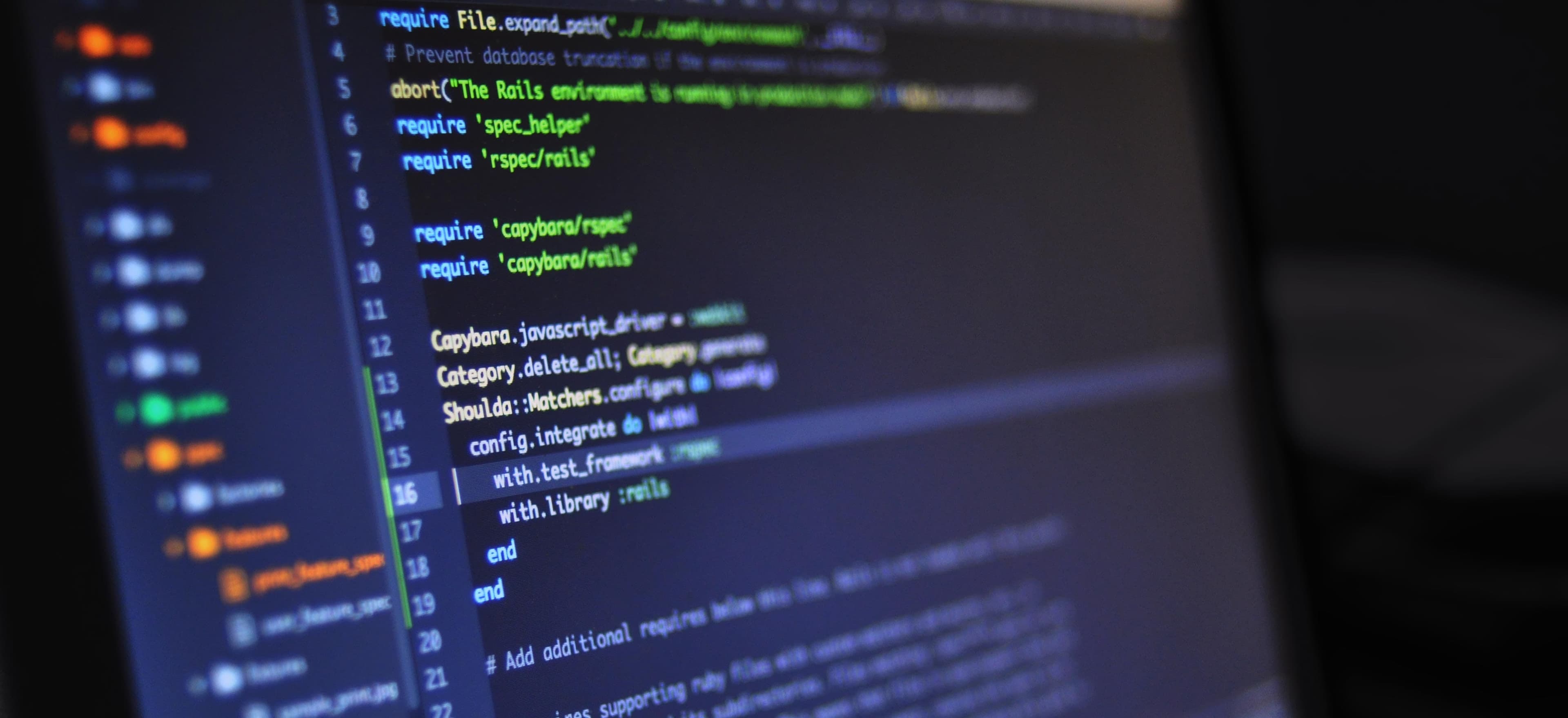
- Published on
Building Resilient Camel Applications with Hystrix DSL
In today's fast-paced, interconnected world, building resilient and fault-tolerant applications is a must. With the rise of microservices architecture, it's essential to handle potential failures gracefully to ensure the overall stability of the system. In the world of Java, Apache Camel is a powerful integration framework widely used for building enterprise integration solutions. In this blog post, we will explore how to leverage the Hystrix DSL to build resilient Camel applications.
Understanding Hystrix
Hystrix is a latency and fault tolerance library designed to isolate points of access to remote systems, services, and 3rd party libraries, stop cascading failures, and enable resilience in complex distributed systems where failure is inevitable. It achieves this by implementing the Circuit Breaker pattern, which can prevent a network or service failure from cascading to other services.
Introducing Hystrix DSL for Camel
Camel, being a versatile integration framework, provides support for using Hystrix. With the Hystrix DSL, developers can easily integrate Hystrix commands into their Camel routes and apply fault tolerance patterns without complex coding.
Setting up the Environment
Before we dive into the implementation, make sure you have the following dependencies in your Maven pom.xml
file:
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-hystrix-starter</artifactId>
<version>x.x.x</version>
</dependency>
Replace x.x.x
with the latest version of Camel.
Implementing Hystrix DSL in Camel Routes
To integrate Hystrix DSL into Camel routes, you can use the hystrix
component in the route definition. Let's consider an example where we have a simple Camel route that consumes messages from a queue and processes them.
import org.apache.camel.builder.RouteBuilder;
public class MyRouteBuilder extends RouteBuilder {
@Override
public void configure() throws Exception {
from("activemq:queue:inputQueue")
.hystrix()
.to("bean:myBean")
.onFallback()
.log("Fallback executed")
.end()
.to("direct:nextRoute");
}
}
In this example, the hystrix()
method is used to wrap the processing logic within a Hystrix command. If the downstream service or bean invocation fails, the fallback route defined by .onFallback()
will be executed. This allows for graceful degradation and fallback strategies in case of failures.
Understanding the 'Why' Behind the Code
The hystrix()
method indicates that the subsequent processing steps should be enclosed within a Hystrix command. This is beneficial because it isolates the execution and provides fault tolerance. The .onFallback()
method defines the fallback route to be executed if the Hystrix command fails, ensuring graceful handling of failures.
Configuring Hystrix Parameters
Hystrix provides a range of configuration options to control the behavior of the Hystrix commands. These parameters include execution timeout, circuit breaker settings, thread pool configuration, and more. Let's see how we can configure these parameters in the Camel route.
import org.apache.camel.builder.RouteBuilder;
import org.apache.camel.component.hystrix.metrics.servlet.HystrixEventStreamServlet;
public class MyRouteBuilder extends RouteBuilder {
@Override
public void configure() throws Exception {
from("activemq:queue:inputQueue")
.hystrix()
.hystrixConfiguration()
.executionTimeoutInMilliseconds(500)
.circuitBreakerRequestVolumeThreshold(20)
.metricsRollingStatisticalWindowInMilliseconds(10000)
.metricsRollingStatisticalWindowBuckets(10)
.ThreadPoolKey("MyThreadPool")
.ThreadPoolCoreSize(10)
.ThreadPoolMaxSize(15)
.end()
.to("bean:myBean")
.onFallback()
.log("Fallback executed")
.end()
.to("direct:nextRoute");
}
}
In this example, we have configured various Hystrix parameters using the hystrixConfiguration()
method. These settings allow fine-grained control over the behavior of the Hystrix commands, such as setting the execution timeout, defining circuit breaker thresholds, and tuning the thread pool settings for Hystrix command execution.
Understanding the 'Why' Behind the Code
Configuring Hystrix parameters is crucial to tailor the behavior of Hystrix commands based on the application's specific requirements. For instance, setting the executionTimeoutInMilliseconds
ensures that the Hystrix command does not exceed a certain time threshold, preventing long-running or stuck commands. Similarly, tuning circuit breaker thresholds and thread pool settings can directly impact the resilience and performance of the system.
Monitoring Hystrix Metrics
Monitoring and understanding the Hystrix metrics can provide valuable insights into the performance and resilience of the Camel application. Hystrix provides a dashboard for visualizing these metrics, which can be enabled in a standalone Hystrix dashboard or integrated with tools like Turbine or Hystrix Dashboard.
Key Takeaways
In conclusion, leveraging the Hystrix DSL in Apache Camel allows developers to build resilient and fault-tolerant integration solutions effortlessly. By integrating the Circuit Breaker pattern and fault tolerance mechanisms, Camel applications can gracefully handle failures and prevent cascading issues in distributed systems. The configurable nature of Hystrix parameters enables fine-tuning the resilience strategy based on specific application requirements. Monitoring Hystrix metrics provides valuable insights into the system's performance and resilience. As businesses continue to rely on interconnected systems and microservices, building resilient Camel applications with Hystrix DSL is crucial for ensuring the stability and reliability of the overall ecosystem.
Start building resilient Camel applications with Hystrix DSL today and empower your integration solutions with fault tolerance and resilience.
Explore Apache Camel for more integration possibilities.