Efficient Integration Testing with Maven and Docker
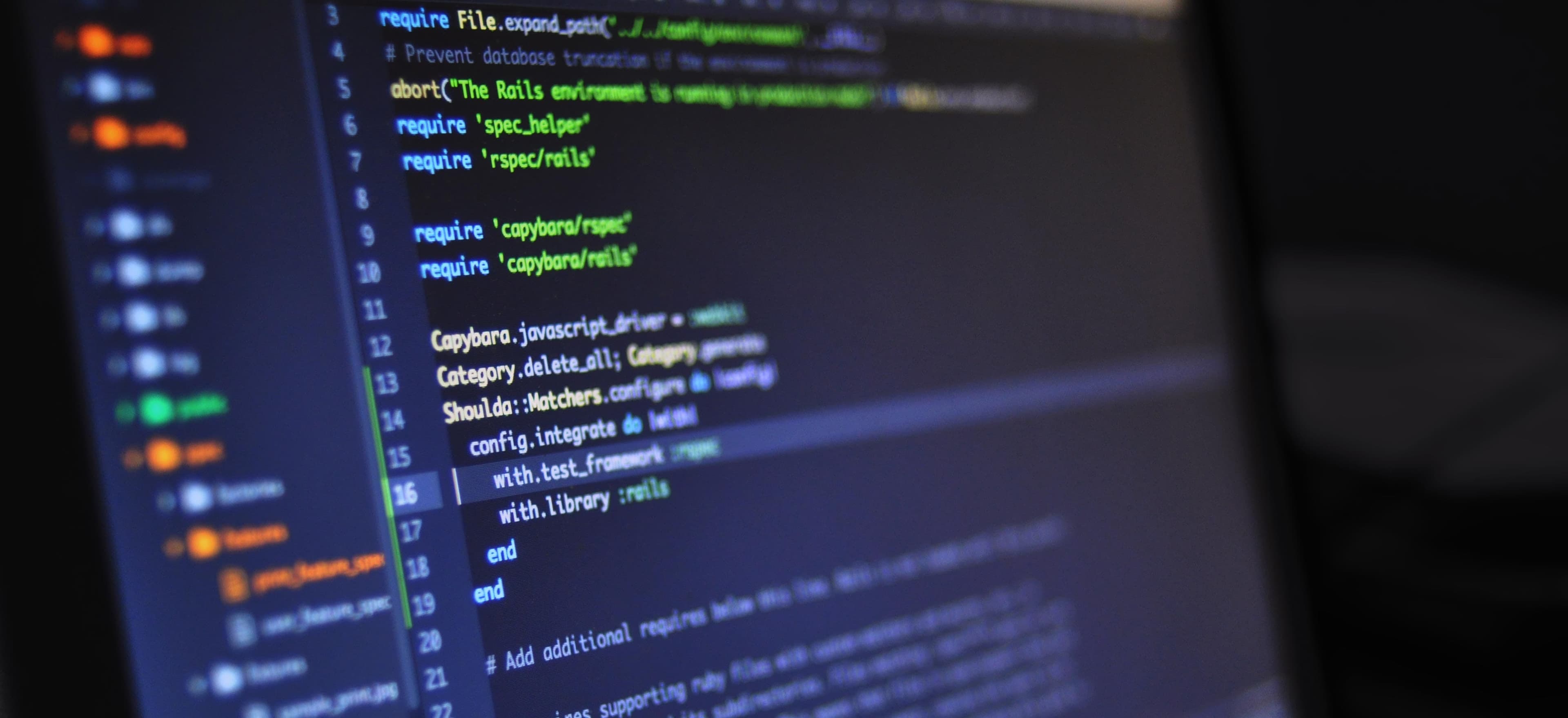
- Published on
Efficient Integration Testing with Maven and Docker
Integration testing is a crucial part of the software development lifecycle, ensuring that all the components of an application work together as expected. In this article, we will explore how to efficiently perform integration testing using Maven and Docker. We will discuss the benefits of integration testing, setting up a sample Java application, integrating Maven with Docker, and finally, running integration tests using Docker containers.
Why Integration Testing?
Integration testing verifies the interaction between different parts of a system, catching issues that unit tests might miss. It ensures that components work together as intended, identifying integration flaws early in the development process. This type of testing boosts confidence in the overall system and reduces the likelihood of encountering major issues in production.
Setting Up a Sample Java Application
For the purpose of this tutorial, let's consider a simple Java application - a RESTful API built with Spring Boot. You can use any Java application, but having a sample application will help in understanding the integration testing process better.
Here's a basic example of a RESTful API endpoint using Spring Boot:
@RestController
public class HelloController {
@RequestMapping("/")
public String index() {
return "Greetings from Spring Boot!";
}
}
Integrating Maven with Docker
Benefits of Docker for Integration Testing
Docker provides a consistent environment for application components to run, making it an ideal choice for integration testing. By containerizing the components, we can ensure that the tests run in an isolated and reproducible environment, regardless of the host system.
Docker Maven Plugin
The docker-maven-plugin
allows us to build and run Docker images as part of the Maven build lifecycle. We can use this plugin to create containers for our application and any dependencies needed for integration testing.
First, we need to add the docker-maven-plugin
to our Maven project's pom.xml
:
<build>
<plugins>
<plugin>
<groupId>com.spotify</groupId>
<artifactId>docker-maven-plugin</artifactId>
<version>1.2.0</version>
<configuration>
<!-- Docker configuration goes here -->
</configuration>
</plugin>
</plugins>
</build>
Creating a Docker Image
We can use the docker-maven-plugin
to build a Docker image for our sample application. Here's an example configuration for creating a Docker image:
<configuration>
<imageName>sample-app</imageName>
<dockerDirectory>src/main/docker</dockerDirectory>
<resources>
<resource>
<targetPath>/</targetPath>
<directory>${project.build.directory}</directory>
<include>${project.build.finalName}.jar</include>
</resource>
</resources>
</configuration>
In this configuration, we specify the image name, the location of the Dockerfile (in the dockerDirectory
), and the resources to be included in the image.
Running Integration Tests with Docker Containers
Now that we have our Docker image ready, we can use it to run integration tests for our application. We will leverage the failsafe
plugin in Maven to execute our integration tests.
Setting Up Failsafe Plugin
We need to configure the failsafe
plugin in our pom.xml
to execute the integration tests. Here's an example configuration:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-failsafe-plugin</artifactId>
<version>3.0.0-M5</version>
<executions>
<execution>
<goals>
<goal>integration-test</goal>
<goal>verify</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
Configuring Integration Tests
To run our integration tests inside Docker containers, we need to configure the plugin to start and stop the containers during the pre-integration-test
and post-integration-test
phases. Here's an example configuration:
<configuration>
<systemPropertyVariables>
<spring.profiles.active>test</spring.profiles.active>
</systemPropertyVariables>
<containers>
<container>
<name>sample-app-test</name>
<wait>
<time>120000</time>
</wait>
</container>
</containers>
</configuration>
In this configuration, we set an active Spring profile for testing and specify the Docker container to be started and waited upon.
The Bottom Line
In this tutorial, we explored the advantages of integration testing, set up a sample Java application, integrated Maven with Docker using the docker-maven-plugin
, and ran integration tests with Docker containers using the failsafe
plugin. By leveraging Docker for integration testing, we ensure consistent and reliable testing environments, ultimately leading to higher-quality software.
By following these steps, you can streamline your integration testing process and build robust, reliable applications with confidence.
Integration testing is a crucial part of the software development lifecycle, ensuring that all parts of an application work together as expected. Here's a basic example of a RESTful API endpoint using Spring Boot:
@RestController
public class HelloController {
@RequestMapping("/")
public String index() {
return "Greetings from Spring Boot!";
}
}
Integrating Maven with Docker
Docker provides a consistent environment for application components to run, making it an ideal choice for integration testing.
The docker-maven-plugin
allows us to build and run Docker images as part of the Maven build lifecycle.
Creating a Docker Image
We can use the docker-maven-plugin
to build a Docker image for our sample application.
In this configuration, we specify the image name, the location of the Dockerfile, and the resources to be included in the image.
Running Integration Tests with Docker Containers
Now that we have our Docker image ready, we can use it to run integration tests for our application.
We need to configure the failsafe
plugin in our pom.xml
to execute the integration tests.
To run our integration tests inside Docker containers, we need to configure the plugin to start and stop the containers during the pre-integration-test
and post-integration-test
phases.
The Bottom Line
In this tutorial, we explored the advantages of integration testing, set up a sample Java application, integrated Maven with Docker using the docker-maven-plugin
, and ran integration tests with Docker containers using the failsafe
plugin. By leveraging Docker for integration testing, we ensure consistent and reliable testing environments, ultimately leading to higher-quality software.