Implementing the Trait Pattern in Java
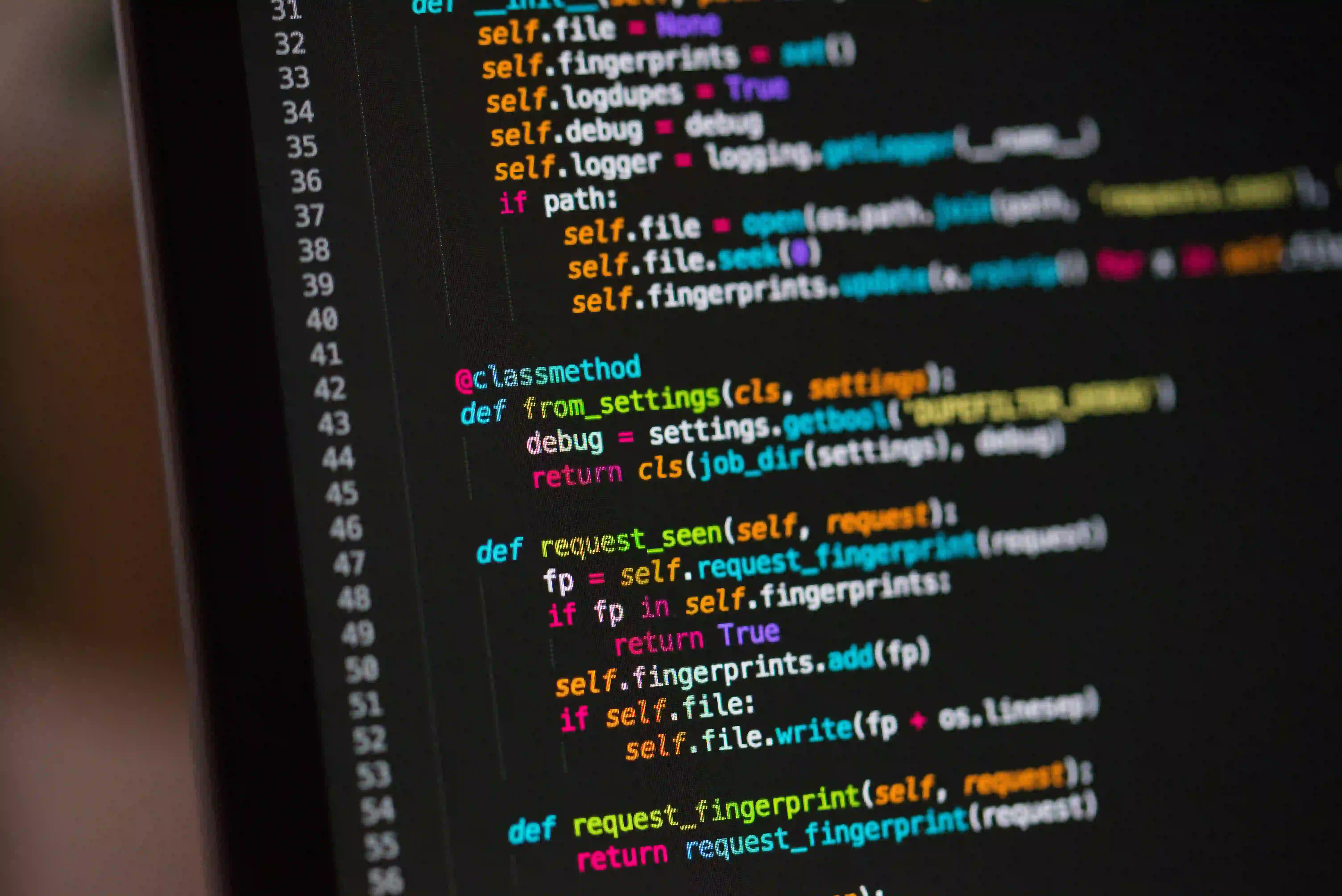
When it comes to designing and implementing object-oriented software in Java, the trait pattern is a powerful tool that can greatly enhance code reusability and maintainability. By allowing multiple inheritance of behavior, the trait pattern enables the composition of classes without the need for traditional inheritance hierarchies. This blog post will delve into the concept of traits, discuss their advantages, and provide a practical example of implementing the trait pattern in Java.
Understanding Traits
A trait can be conceptualized as a set of methods that can be applied to various classes. Unlike traditional inheritance, where a subclass inherits behavior from a single parent class, a class can include multiple traits, each providing a distinct set of methods. This allows for a more flexible and modular approach to code composition, as classes can be constructed dynamically from a combination of traits, without being bound to a specific inheritance chain.
Advantages of Traits
- Code Reusability: Traits enable the reuse of code across multiple classes without requiring a deep inheritance hierarchy. This promotes modular and composable designs, reducing the need for duplicating code.
- Flexibility: By allowing classes to dynamically acquire behavior from multiple sources, traits provide a high degree of flexibility in constructing class hierarchies and composing functionality.
- Avoiding Diamond Problem: The diamond problem, which occurs in languages with multiple inheritance, is mitigated by the trait pattern as it does not introduce conflicts arising from shared base classes.
Implementing a Trait in Java
Let's consider a practical example to understand how traits can be implemented in Java. Suppose we have two classes, Bird
and Fish
, each representing a type of animal. We want to define a common behavior for these classes, such as movement()
, eat()
, and reproduce()
. Instead of creating a traditional inheritance hierarchy, we can design traits for these behaviors and compose them into our classes.
First, let's define a trait for movement()
:
public interface Movable {
void movement();
}
In this trait, we declare the method movement()
without providing an implementation. This allows classes to implement the Movable
trait and define their specific movement behavior.
Next, we create a trait for eat()
:
public interface Eatable {
void eat();
}
Similarly, the Eatable
trait declares the eat()
method, leaving the implementation to the classes that use this trait.
Finally, a trait for reproduce()
can be defined:
public interface Reproducible {
void reproduce();
}
Composing Traits into Classes
Now, let's see how we can compose these traits into our Bird
and Fish
classes.
We start by implementing the Bird
class:
public class Bird implements Movable, Eatable, Reproducible {
// Implement the Movable trait
@Override
public void movement() {
System.out.println("Flies in the sky");
}
// Implement the Eatable trait
@Override
public void eat() {
System.out.println("Eats seeds and insects");
}
// Implement the Reproducible trait
@Override
public void reproduce() {
System.out.println("Lays eggs for reproduction");
}
// Other bird-specific methods and properties
}
By implementing the Movable
, Eatable
, and Reproducible
traits, the Bird
class acquires the behavior defined in each of these traits.
Similarly, the Fish
class can be implemented in a similar fashion:
public class Fish implements Movable, Eatable, Reproducible {
// Implement the Movable trait
@Override
public void movement() {
System.out.println("Swims in the water");
}
// Implement the Eatable trait
@Override
public void eat() {
System.out.println("Feeds on plankton and smaller fish");
}
// Implement the Reproducible trait
@Override
public void reproduce() {
System.out.println("Lays eggs for reproduction");
}
// Other fish-specific methods and properties
}
Using Traits for Composition
One of the key advantages of the trait pattern is its ability to dynamically compose classes from a combination of traits. This allows for a high degree of flexibility in designing class hierarchies and promoting code reusability.
Suppose we want to create a new class, Penguin
, which shares the traits of both Bird
and Fish
. Using the trait pattern, we can easily compose the desired behavior for the Penguin
class by including the Movable
trait from the Bird
class and the Eatable
and Reproducible
traits from the Fish
class.
public class Penguin implements Movable, Eatable, Reproducible {
// Implement the Movable trait from Bird
@Override
public void movement() {
System.out.println("Swims in the water and walks on land");
}
// Implement the Eatable trait from Fish
@Override
public void eat() {
System.out.println("Consumes fish and small crustaceans");
}
// Implement the Reproducible trait from Fish
@Override
public void reproduce() {
System.out.println("Lays eggs for reproduction");
}
// Other penguin-specific methods and properties
}
In this example, the Penguin
class is composed of traits from both the Bird
and Fish
classes, showcasing the flexibility and modularity of the trait pattern.
Closing the Chapter
In conclusion, the trait pattern provides a powerful mechanism for composing classes in Java, offering benefits such as code reusability, flexibility in class hierarchy design, and mitigation of the diamond problem. By using traits, developers can create modular and composable code that is easier to maintain and extend.
Java's interface mechanism, combined with default methods introduced in Java 8, makes it a suitable language for implementing the trait pattern. Leveraging these language features, developers can effectively apply the trait pattern to design robust and flexible class hierarchies.
In your own projects, consider how the trait pattern can enhance the maintainability and reusability of your code. By embracing the principles of traits, you can create software that is easier to extend, maintain, and reason about.
References: