Optimizing ListViews with Volley in Android
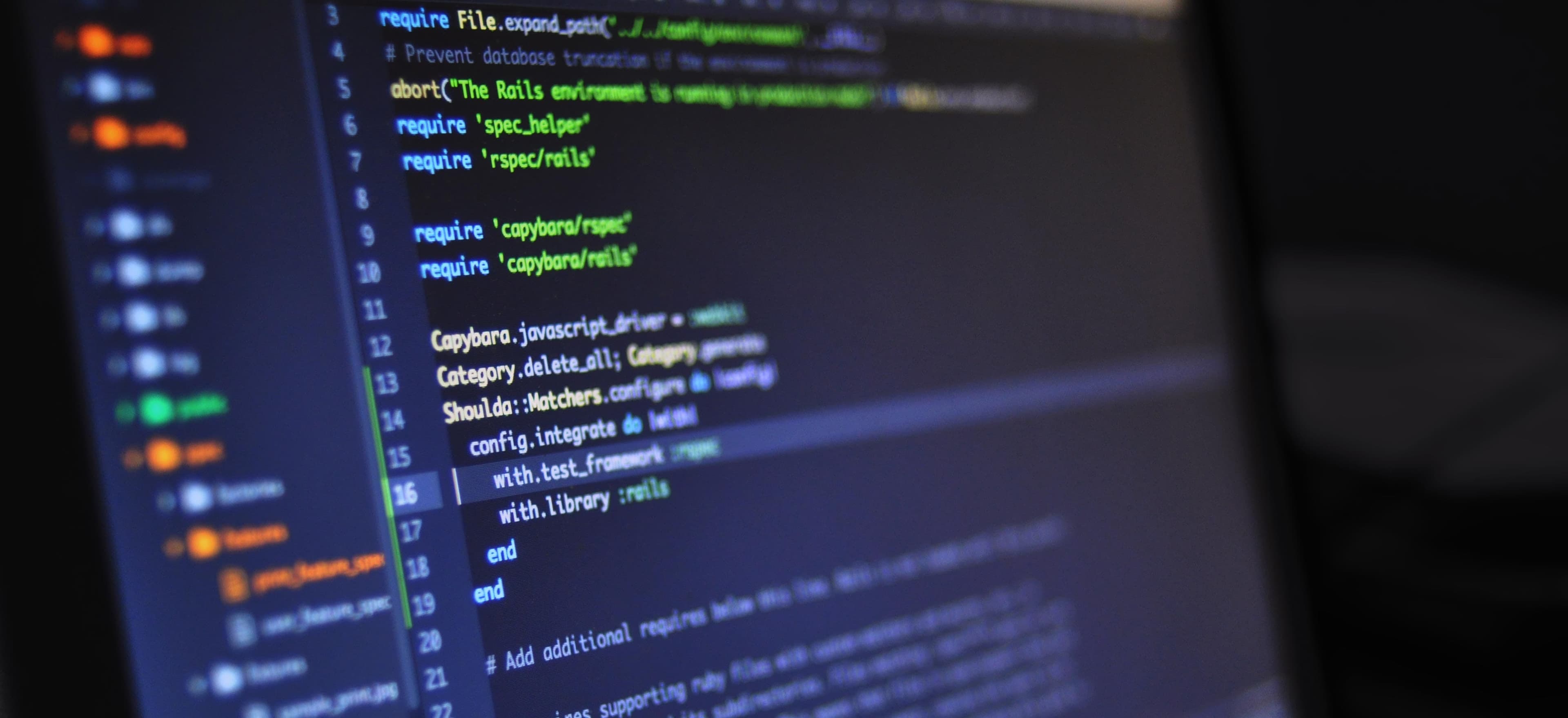
- Published on
Optimizing ListViews with Volley in Android
In Android app development, displaying lists of data is a common requirement. Whether it's a list of products, contacts, or messages, the ListView is a fundamental component for presenting scrollable lists of data. However, when dealing with large datasets or remote data fetching, optimizing the ListView becomes crucial for maintaining a smooth and efficient user experience.
In this article, we'll explore how to optimize ListViews in Android using Volley, a widely used HTTP library for network operations, to efficiently fetch and display data in a ListView.
Why Optimize ListViews with Volley?
ListViews in Android are populated using adapters that fetch and load data into individual list items. When dealing with remote data, fetching and loading data can be a time-consuming process, especially if not implemented efficiently. Volley comes into the picture as an efficient networking library that handles HTTP requests and responses asynchronously, making it ideal for optimizing the fetching and loading of data in ListViews.
Setting Up Volley in Your Android Project
Before diving into optimizing ListViews, you need to integrate Volley into your Android project. Adding Volley to your project is straightforward and can be done by including the Volley library as a dependency in your app's build.gradle file.
dependencies {
implementation 'com.android.volley:volley:1.2.0'
}
After adding the dependency, synchronize your project to ensure that the Volley library is downloaded and available for use in your project.
Implementing a Custom Adapter for ListView
To optimize the ListView with Volley, we need to create a custom adapter that efficiently handles data loading and populating the ListView with fetched data. Let's create a custom adapter that leverages Volley for data fetching and loading.
public class CustomListAdapter extends BaseAdapter {
private Context context;
private List<DataModel> dataList;
private LayoutInflater inflater;
private ImageLoader imageLoader;
private RequestQueue requestQueue;
public CustomListAdapter(Context context, List<DataModel> dataList) {
this.context = context;
this.dataList = dataList;
inflater = LayoutInflater.from(context);
requestQueue = Volley.newRequestQueue(context);
imageLoader = new ImageLoader(requestQueue, new ImageLoader.ImageCache() {
private final LruCache<String, Bitmap> cache = new LruCache<>(20);
@Override
public Bitmap getBitmap(String url) {
return cache.get(url);
}
@Override
public void putBitmap(String url, Bitmap bitmap) {
cache.put(url, bitmap);
}
});
}
// Implement getView, getItem, getItemId, and getCount methods
// ...
static class ViewHolder {
ImageView thumbnail;
TextView title;
TextView description;
}
}
In the above code, we create a custom adapter CustomListAdapter
that extends BaseAdapter
. Within the constructor, we initialize the RequestQueue
and ImageLoader
provided by Volley, allowing efficient HTTP request handling and image loading for each list item.
Loading Data into ListItems with Volley
Now that we have our custom adapter set up, we can leverage Volley to efficiently load data into the ListView. Let's look at how we can use Volley to fetch data and populate the ListView with the fetched data.
public class CustomListAdapter extends BaseAdapter {
// ... (previous code)
@Override
public View getView(final int position, View view, ViewGroup parent) {
final ViewHolder holder;
if (view == null) {
holder = new ViewHolder();
view = inflater.inflate(R.layout.list_item, null);
holder.thumbnail = view.findViewById(R.id.thumbnail);
holder.title = view.findViewById(R.id.title);
holder.description = view.findViewById(R.id.description);
view.setTag(holder);
} else {
holder = (ViewHolder) view.getTag();
}
DataModel data = dataList.get(position);
holder.title.setText(data.getTitle());
holder.description.setText(data.getDescription());
String imageUrl = data.getImageUrl();
imageLoader.get(imageUrl, new ImageLoader.ImageListener() {
@Override
public void onResponse(ImageLoader.ImageContainer response, boolean isImmediate) {
Bitmap bitmap = response.getBitmap();
if (bitmap != null) {
holder.thumbnail.setImageBitmap(bitmap);
}
}
@Override
public void onErrorResponse(VolleyError error) {
// Handle error
}
});
return view;
}
// ...
}
In the getView
method of our custom adapter, we use Volley's ImageLoader
to asynchronously load images for each list item. This approach ensures that images are loaded efficiently without blocking the UI thread, contributing to a smooth user experience when scrolling through the ListView. Additionally, data such as titles and descriptions are directly set to the corresponding views, ensuring efficient data loading for non-image content.
Optimizing Network Requests with Volley
One of the key advantages of using Volley for ListView optimization is its ability to optimize network requests. Volley provides request prioritization, caching, and efficient request management, which can significantly improve the performance of data fetching operations in ListViews.
// Prioritizing network requests with Volley
StringRequest highPriorityRequest = new StringRequest(Request.Method.GET, highPriorityUrl, response -> {
// Handle high priority response
}, error -> {
// Handle error
});
highPriorityRequest.setPriority(Request.Priority.HIGH);
requestQueue.add(highPriorityRequest);
In the above example, we prioritize a specific network request using Volley's request prioritization. By assigning a high priority to critical data fetching operations, we ensure that essential data is fetched and loaded promptly, enhancing the overall user experience of the ListView.
Handling Image and Network Caching
Caching images and network responses is another crucial aspect of optimizing ListViews with Volley. By efficiently caching images and network responses, we can reduce redundant network calls and improve the loading speed of data in ListViews.
Volley provides built-in support for both image caching and network response caching, allowing us to take advantage of these features in ListView optimization.
// Enabling image and network response caching with Volley
requestQueue = Volley.newRequestQueue(context);
imageLoader = new ImageLoader(requestQueue, new ImageLoader.ImageCache() {
private final LruCache<String, Bitmap> cache = new LruCache<>(20);
@Override
public Bitmap getBitmap(String url) {
return cache.get(url);
}
@Override
public void putBitmap(String url, Bitmap bitmap) {
cache.put(url, bitmap);
}
});
In the above code snippet, we configure an image cache for the ImageLoader
provided by Volley. By caching images, we can avoid redundant image downloads, leading to improved ListView performance and reduced data usage.
Final Considerations
Optimizing ListViews in Android with Volley is crucial for delivering a smooth and efficient user experience when dealing with large datasets or remote data fetching. By leveraging Volley's asynchronous request handling, image loading, network request prioritization, and caching capabilities, developers can ensure that ListViews perform optimally and provide a seamless user experience.
Incorporating Volley into custom adapters for ListView data loading enables efficient handling of network operations, contributing to faster data fetching and improved overall performance. Moreover, Volley's built-in support for image and network response caching further enhances ListView optimization by reducing unnecessary network calls and speeding up data loading.
By following the best practices and leveraging the features provided by Volley, Android developers can effectively optimize ListViews to deliver a compelling user experience while efficiently managing data fetching and loading operations.
Start optimizing your ListViews with Volley today and elevate your Android app's performance and user satisfaction!
Remember, optimizing ListViews is just one aspect of creating high-performance Android apps. Keep exploring and experimenting with different strategies to continually enhance the user experience of your Android applications.