Optimizing Performance with Spring 5 Caching
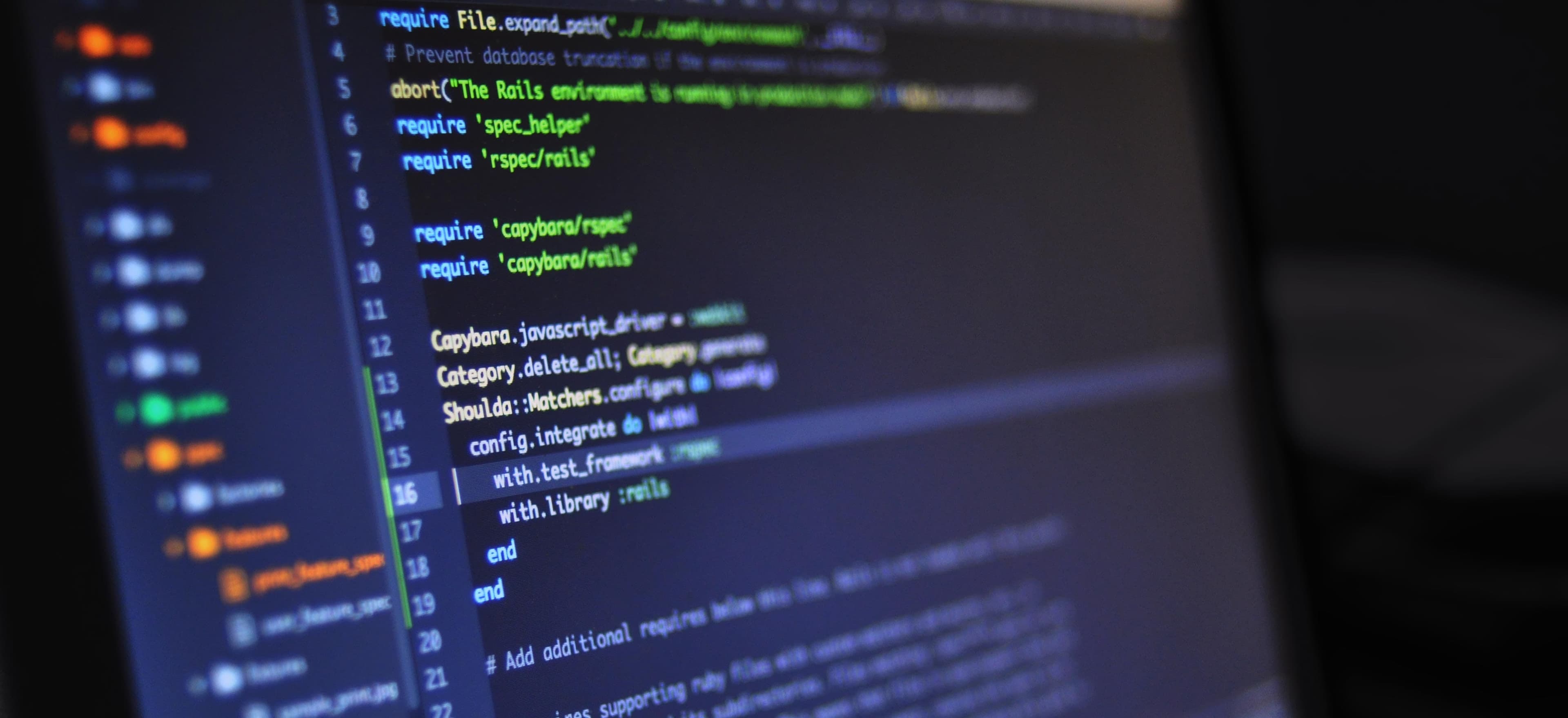
- Published on
Improving Application Performance with Spring 5 Caching
In today's fast-paced world, users expect applications to be responsive and quick. Performance is a critical aspect of any application, and inefficient data retrieval can severely impact the user experience. Caching is a technique used to improve application performance by storing frequently accessed data in memory. In this article, we will explore how to leverage caching in a Java application using the Spring 5 framework.
Understanding Caching
Caching involves storing data in a temporary storage area that can be quickly accessed. When data is requested, the application first checks the cache. If the data is present, it is retrieved from the cache instead of the original data source, such as a database or an external API. This reduces the latency and improves the overall performance of the application.
Introducing Spring 5 Caching
Spring 5 provides support for caching through its caching abstraction. This abstraction allows developers to easily integrate caching capabilities into their applications without being tied to a specific caching implementation. Spring's caching abstraction supports popular caching providers such as Ehcache, Caffeine, Redis, and more.
Configuring Spring 5 Caching
Adding Dependencies
To start using caching with Spring 5, we need to include the appropriate dependencies in our project. For example, if we want to use Ehcache as our caching provider, we can include the following dependencies in our pom.xml
file for a Maven-based project:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
<dependency>
<groupId>net.sf.ehcache</groupId>
<artifactId>ehcache</artifactId>
</dependency>
Enabling Caching
Next, we need to enable caching in our Spring application. We can achieve this by annotating the main application class with @EnableCaching
:
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@EnableCaching
public class MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
}
By adding @EnableCaching
, we enable Spring's caching support for our application.
Applying Caching Annotations
Spring's caching abstraction provides annotations such as @Cacheable
, @CachePut
, and @CacheEvict
to control caching behavior at the method level.
-
@Cacheable
: This annotation is used to cache the result of a method. When the method is invoked, the return value is cached based on the method parameters. Subsequent invocations of the method with the same parameters will retrieve the cached result instead of executing the method again. -
@CachePut
: This annotation is used to update the cache with the result of a method. It always triggers the execution of the method and updates the cache with the method's return value. -
@CacheEvict
: This annotation is used to remove entries from the cache. It can be applied to a method to evict specific cache entries based on the method parameters, or it can clear the entire cache.
Let's look at an example of using @Cacheable
:
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Service;
@Service
public class BookService {
@Cacheable("books")
public Book findBookByIsbn(String isbn) {
// This method will be cached based on the isbn parameter
return databaseService.findBookByIsbn(isbn);
}
}
In this example, the findBookByIsbn
method will be cached based on the isbn
parameter. Subsequent calls with the same isbn
will retrieve the cached result, eliminating the need to query the database again.
Choosing the Right Caching Provider
When selecting a caching provider, it's essential to consider factors such as performance, scalability, and ease of use. Different caching providers offer various features and trade-offs.
-
Ehcache: Ehcache is a popular choice for caching in Java applications. It provides fast in-memory caching and supports disk storage for overflow. Additionally, it seamlessly integrates with Spring through the
EhCacheManagerFactoryBean
. -
Caffeine: Caffeine is a high-performance, near-optimal caching library. It provides an in-memory cache with high hit rates and automatic eviction based on usage patterns.
-
Redis: Redis is an in-memory data store that can be used as a caching provider. It offers persistence, replication, and built-in fault tolerance, making it a robust choice for distributed caching.
Best Practices for Caching
To make the most of caching in Spring 5, consider the following best practices:
-
Identify frequently accessed data: Analyze your application's data access patterns to identify the most frequently accessed data. Cache such data to reduce the load on the underlying data source.
-
Use caching for read-heavy operations: Caching is particularly effective for read-heavy operations where the data is accessed more frequently than it is updated.
-
Fine-tune caching parameters: Adjust caching parameters such as expiration time, eviction policies, and cache sizes to optimize performance based on your application's requirements.
-
Monitor and measure: Use tools like Micrometer or Spring Boot Actuator to monitor cache usage, hit rates, and performance metrics. This data can help you make informed decisions about cache configuration and optimizations.
Final Considerations
Incorporating caching into your Spring 5 application is a powerful way to enhance performance and improve the overall user experience. By leveraging Spring's caching abstraction and choosing the right caching provider, you can efficiently manage data access and significantly reduce response times. Remember to analyze your application's access patterns, apply caching selectively, and continuously monitor and optimize your caching strategy for the best results.
To delve deeper into caching with Spring 5, consider exploring the official Spring documentation and experimenting with different caching providers to determine the best fit for your application's needs.
Optimizing performance through caching is a crucial aspect of modern application development. With Spring 5, you have a powerful ally to bolster your application's responsiveness and scalability.