Optimizing Wordcount Performance in Apache Storm with Scala
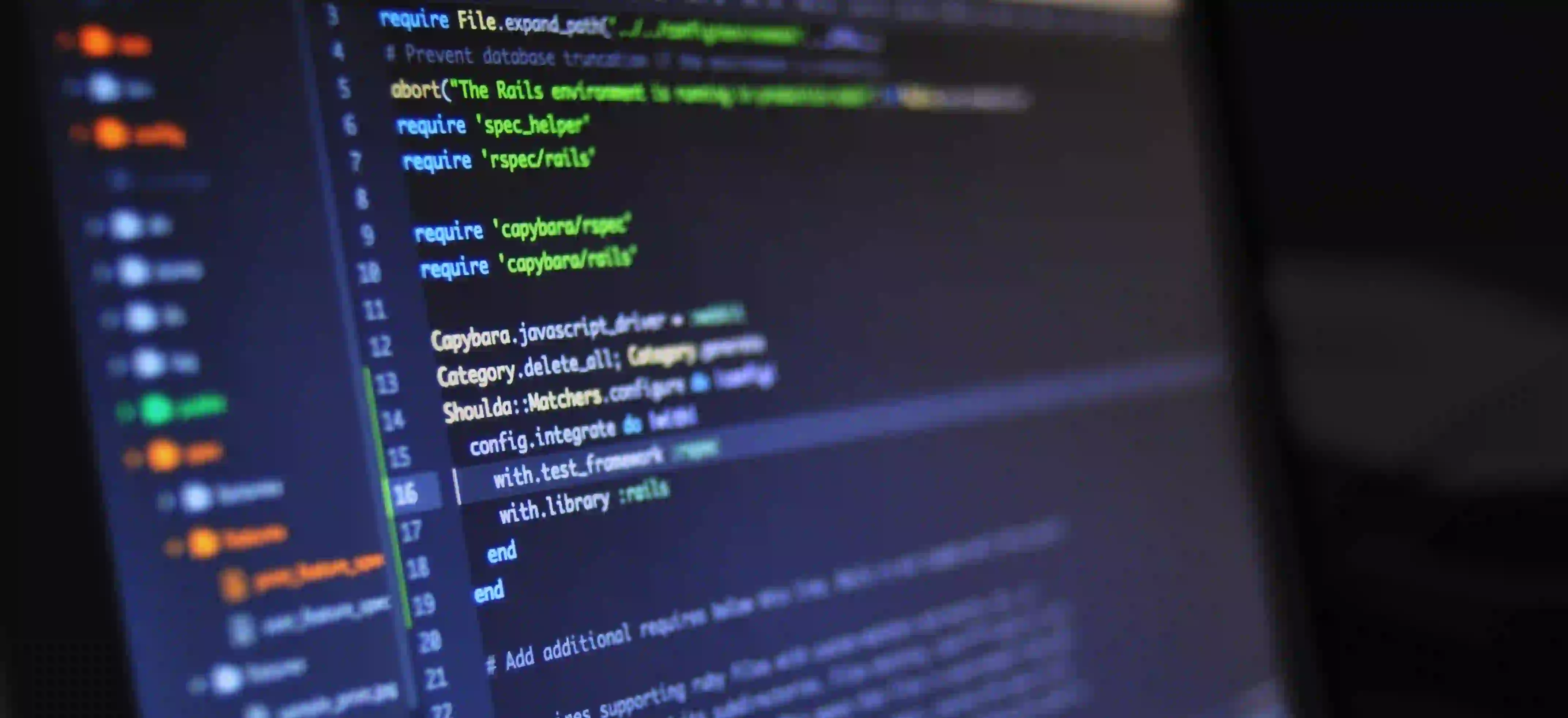
Improving Wordcount Performance in Apache Storm with Scala
Apache Storm is a real-time computation system that allows for distributed processing of streaming data. When working with large-scale data, optimizing performance becomes crucial. In this post, we will discuss how to improve the performance of a wordcount topology in Apache Storm using Scala. We'll explore various techniques and best practices to optimize the wordcount performance in Apache Storm while leveraging the power of Scala.
Understanding the Wordcount Topology
The wordcount topology is a common example used to demonstrate the basic concepts of Apache Storm. It involves processing a stream of text data, splitting the text into individual words, and then counting the occurrences of each word. While seemingly simple, optimizing the performance of this topology is essential, especially when dealing with large volumes of data.
Leveraging Scala for Performance Gains
Scala, known for its concise syntax and functional programming capabilities, can be leveraged to optimize the performance of Apache Storm topologies. By utilizing Scala’s powerful features, we can write efficient, high-performance code for our wordcount topology.
Using Immutable Collections for Efficiency
In Scala, immutable collections are the default choice, promoting safer and more efficient code. When implementing the wordcount topology in Apache Storm using Scala, leveraging immutable collections such as List
or Map
can lead to better performance and concurrency control.
// Example of using immutable map for wordcount
val words: List[String] = // obtain list of words from stream
val wordCountMap: Map[String, Int] = words.groupBy(identity).mapValues(_.size)
Using immutable collections ensures thread safety and avoids the need for expensive synchronization mechanisms, resulting in better performance.
Employing Pattern Matching for Stream Processing
Scala’s pattern matching is a powerful feature that can be used for efficient stream processing in Apache Storm. Pattern matching allows for concise and expressive handling of different cases, which can lead to optimized performance in processing incoming data streams.
// Using pattern matching for stream processing
def processWord(word: String, countMap: Map[String, Int]): Map[String, Int] = countMap.get(word) match {
case Some(count) => countMap + (word -> (count + 1))
case None => countMap + (word -> 1)
}
By leveraging pattern matching, we can write streamlined and performant code for processing incoming data within the wordcount topology.
Implementing Functional Programming Concepts
Scala’s support for functional programming enables the use of higher-order functions, immutability, and composition, which can contribute to the optimization of the wordcount topology in Apache Storm.
// Using functional programming for wordcount
val words: List[String] = // obtain list of words from stream
val wordCountMap: Map[String, Int] = words.foldLeft(Map.empty[String, Int]) { (countMap, word) =>
countMap + (word -> (countMap.getOrElse(word, 0) + 1))
}
By employing functional programming concepts such as foldLeft
, we can write concise and efficient code for wordcount processing, enhancing the overall performance of the Apache Storm topology.
Leveraging Parallel Processing with Scala
Scala provides robust support for parallel processing through constructs like parallel collections and parallel processing libraries. By exploiting Scala’s parallel processing capabilities, we can optimize the performance of the wordcount topology in Apache Storm, especially when dealing with sizable data streams.
// Leveraging parallel collections for wordcount
val words: List[String] = // obtain list of words from stream
val wordCountMap: Map[String, Int] = words.par.groupBy(identity).mapValues(_.size)
Utilizing parallel collections in Scala enables concurrent processing of data, leading to potential performance gains in the wordcount topology within Apache Storm.
To Wrap Things Up
In conclusion, by employing the features and capabilities of Scala, we can optimize the performance of the wordcount topology in Apache Storm. Leveraging immutable collections, pattern matching, functional programming concepts, and parallel processing capabilities can contribute to the efficient processing of streaming data within Apache Storm. By embracing Scala’s strengths, we can enhance the performance and scalability of real-time processing applications built on Apache Storm while achieving optimal wordcount performance.
To delve deeper into Apache Storm, Scala, and performance optimization, refer to the official Apache Storm documentation, Scala official website, and Scala parallel collections guide. Happy optimizing!
By incorporating these techniques and best practices, you can boost the performance of your Apache Storm wordcount topology while benefiting from the elegance and power of Scala.