Troubleshooting Remote Control Connectivity Issues
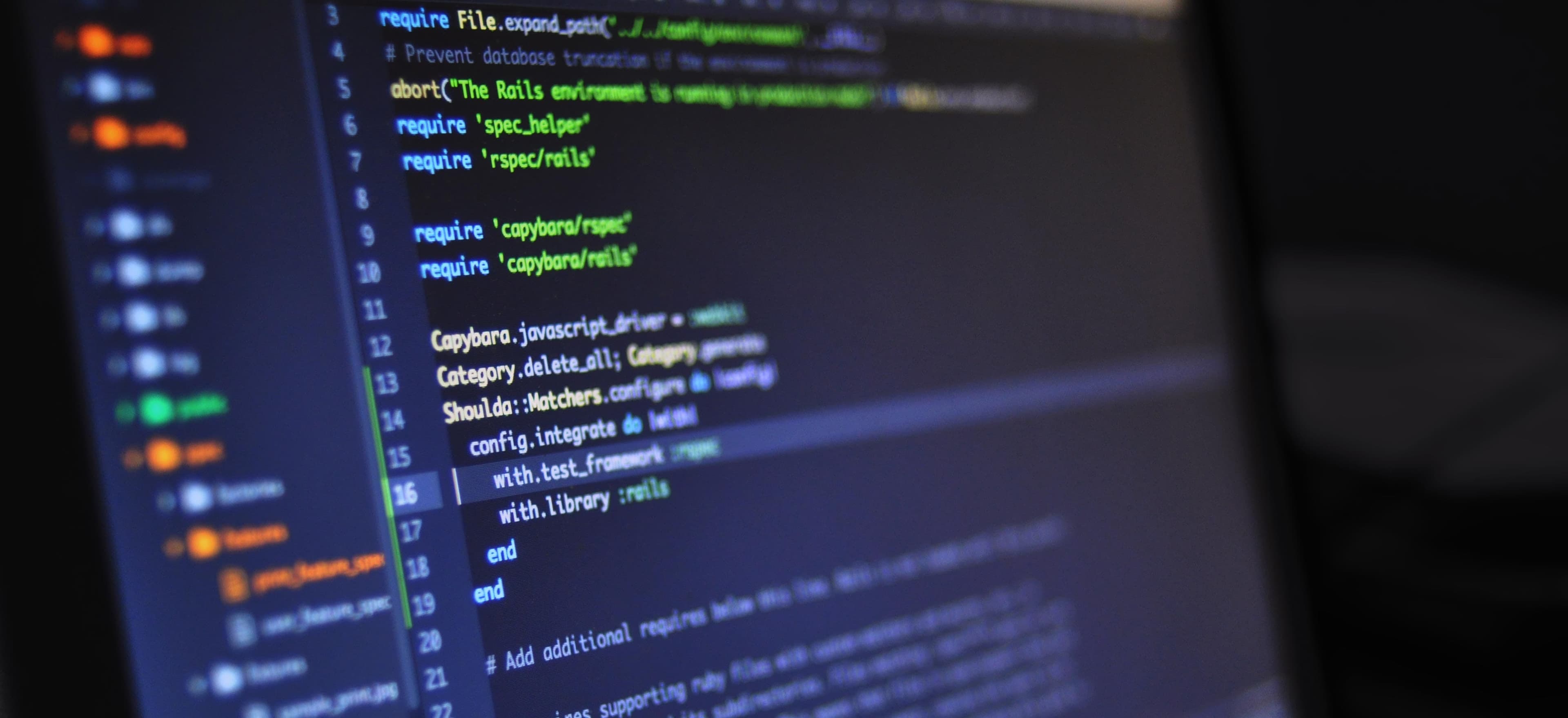
- Published on
Troubleshooting Remote Control Connectivity Issues
As a Java developer, working with remote services or APIs is inevitable. However, connectivity issues can often arise, causing frustration and delays. In this post, we will discuss some common connectivity issues when working with remote services in Java, and how to troubleshoot and resolve them effectively.
1. Check Network Connectivity
Before diving into the code, it's crucial to verify the network connectivity from the machine where your Java application is running. Use tools like ping
or telnet
to ensure that the remote server is accessible from your environment.
try {
InetAddress address = InetAddress.getByName("remote-server-url");
if (address.isReachable(10000)) {
System.out.println("Host is reachable");
} else {
System.out.println("Host is NOT reachable");
}
} catch (IOException e) {
System.err.println("Exception occurred: " + e.getMessage());
}
2. Verify Firewall Settings
Firewalls can sometimes block outgoing connections from your Java application. Ensure that the necessary ports are open and the firewall rules permit outbound connections to the remote server.
3. Check Proxy Settings
If your network requires a proxy for outbound connections, ensure that the proxy settings are configured correctly in your Java application.
System.setProperty("https.proxyHost", "your-proxy-host");
System.setProperty("https.proxyPort", "your-proxy-port");
4. Verify SSL/TLS Configuration
When connecting to a remote server over HTTPS, ensure that the SSL/TLS configuration is set up correctly. This involves validating the server's SSL certificate and configuring the truststore appropriately.
System.setProperty("javax.net.ssl.trustStore", "path/to/truststore.jks");
System.setProperty("javax.net.ssl.trustStorePassword", "truststore-password");
5. Handle Timeouts
Timeouts are crucial when dealing with remote services. Set appropriate connection and read timeouts to prevent your application from hanging indefinitely.
int connectionTimeout = 5000; // 5 seconds
int readTimeout = 10000; // 10 seconds
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setConnectTimeout(connectionTimeout);
conn.setReadTimeout(readTimeout);
6. Use Robust Retry Mechanisms
Intermittent network issues can be resolved with retry mechanisms to reattempt the connection in case of transient failures.
int maxTries = 3;
int delay = 1000; // 1 second delay between retries
for (int i = 0; i < maxTries; i++) {
try {
// Make the remote service call
break;
} catch (IOException e) {
Thread.sleep(delay);
}
}
7. Logging and Monitoring
Implement robust logging within your application to capture network-related errors and monitor the network traffic to identify patterns or anomalies.
In conclusion, troubleshooting remote control connectivity issues in Java requires a combination of network diagnostics, configuration verification, and robust error handling within your application. By following the steps outlined in this post, you can effectively identify and resolve connectivity issues, ensuring smooth interactions with remote services.
Checkout our other articles