Optimizing Animation Performance in JavaFX
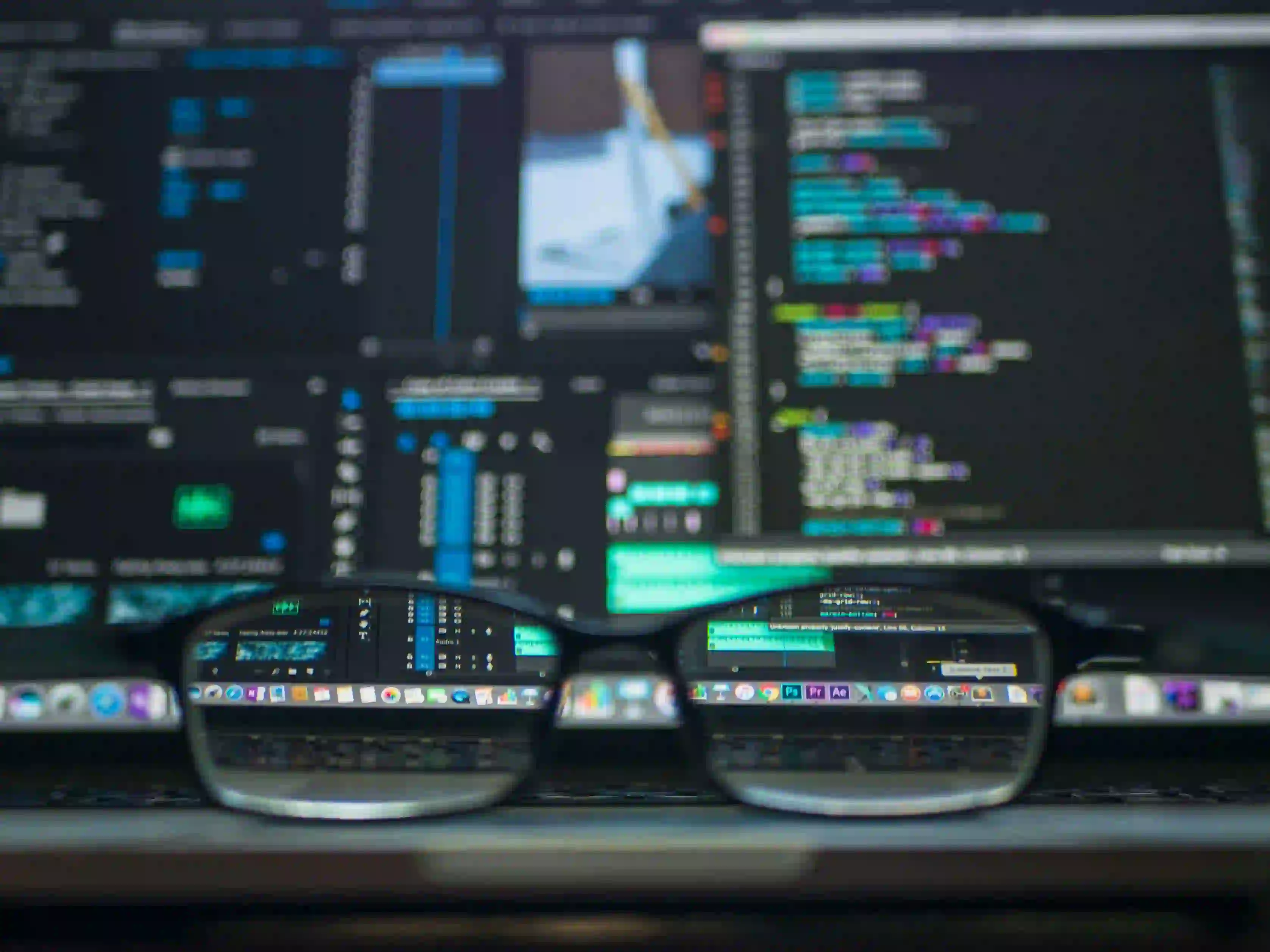
Improving Animation Performance in JavaFX
Animation plays a crucial role in modern UI/UX design, creating a more engaging and interactive user experience. However, when it comes to implementing animations in Java applications, performance issues can often arise. In this blog post, we will explore various techniques to optimize animation performance in JavaFX, ensuring smooth and efficient animations in your applications.
Understanding the Performance Challenges
Before delving into optimization techniques, it's essential to understand the performance challenges involved in animation rendering. JavaFX, being a retained mode rendering API, requires frequent updates to the scene graph for animations. This can lead to performance bottlenecks, especially when dealing with complex or high-frequency animations.
Leveraging Hardware Acceleration
One of the most effective ways to improve animation performance in JavaFX is by leveraging hardware acceleration. JavaFX provides support for hardware acceleration through the Prism rendering pipeline, which offloads the rendering tasks to the GPU, resulting in faster and smoother animations.
To enable hardware acceleration in JavaFX, you can use the following system property:
System.setProperty("prism.order", "d3d");
By setting the prism.order
property to "d3d"
, you instruct JavaFX to use the Direct3D pipeline for rendering, provided that the system supports it. This can significantly boost animation performance, especially on systems with dedicated GPUs.
Caching and Bitmap Caching
Another technique to enhance animation performance in JavaFX is by utilizing caching and bitmap caching. Caching allows JavaFX to render complex nodes or effects into an image, which is then reused during subsequent frames, reducing the rendering overhead.
You can enable cache for a node in JavaFX as follows:
Node node = new Node();
node.setCache(true);
Bitmap caching, on the other hand, caches the rendered content of a node as a bitmap, which is particularly useful for nodes with expensive rendering operations, such as complex shapes or effects.
Node node = new Node();
node.setCache(true);
node.setCacheHint(CacheHint.SPEED);
By setting the cache hint to CacheHint.SPEED
, you prioritize speed over memory usage, which is beneficial for animations requiring frequent updates.
Using Pulse Animation
JavaFX provides the Pulse
API, which allows you to synchronize animations with the pulse mechanism of the JavaFX rendering pipeline. By aligning your animations with the pulse, you can achieve smoother transitions and avoid off-cycle rendering, thus improving overall animation performance.
Here's an example of using the Pulse
API to create a synchronized animation:
AnimationTimer timer = new AnimationTimer() {
@Override
public void handle(long now) {
// Update animation logic here
}
};
timer.start();
By leveraging the AnimationTimer
and aligning the animation updates with the pulse, you can ensure optimal performance and visual consistency in your animations.
Optimizing Animation Logic
In addition to rendering optimizations, refining the animation logic can also contribute to improved performance. When updating animations, ensure that you minimize unnecessary computations and only modify the necessary nodes or properties for each frame. This approach can reduce the processing overhead and enhance the overall responsiveness of the animations.
Preferring Shape-based Nodes
When designing UI elements for animated scenes, it's advisable to prefer shape-based nodes, such as Rectangle
, Circle
, or Polygon
, over complex image-based nodes. Shape-based nodes are more lightweight and easier for the rendering pipeline to handle, leading to better animation performance, especially in scenarios involving a large number of nodes.
Using JavaFX Animation API
JavaFX provides a comprehensive set of animation APIs, such as Transition
, Timeline
, and KeyFrame
, which offer predefined animation behaviors and interpolation options. Leveraging these built-in animation classes not only simplifies the animation implementation but also ensures optimized performance, as they are designed to work efficiently within the JavaFX rendering pipeline.
Utilizing Scene Graph Optimization Techniques
Optimizing the structure of the scene graph can significantly impact animation performance. Minimize the depth of the scene graph by flattening hierarchies and avoiding unnecessary groupings. Additionally, consider using static or reusable nodes whenever possible to reduce the overhead of scene graph updates during animations.
Profiling and Monitoring Performance
Lastly, it's essential to profile and monitor the performance of your JavaFX animations using tools like VisualVM or Java Mission Control. These tools can provide insights into CPU, GPU, and memory usage, helping you identify potential bottlenecks and optimize your animations accordingly.
Closing Remarks
Optimizing animation performance in JavaFX is crucial for delivering a seamless and enjoyable user experience. By leveraging hardware acceleration, caching, synchronization with the pulse, and refining animation logic, you can ensure that your JavaFX animations run smoothly and efficiently. Additionally, utilizing the JavaFX animation API and applying scene graph optimization techniques can further enhance the performance of your animations. Remember to profile and monitor performance to fine-tune your optimizations effectively.
With these optimization techniques in mind, you can elevate the visual appeal of your JavaFX applications while maintaining optimal performance.
For further reading, consider exploring Oracle's official documentation for JavaFX and the JavaFX performance tips provided by the OpenJFX project.
Happy optimizing!