The Intricacies of Filesystemmap in Python
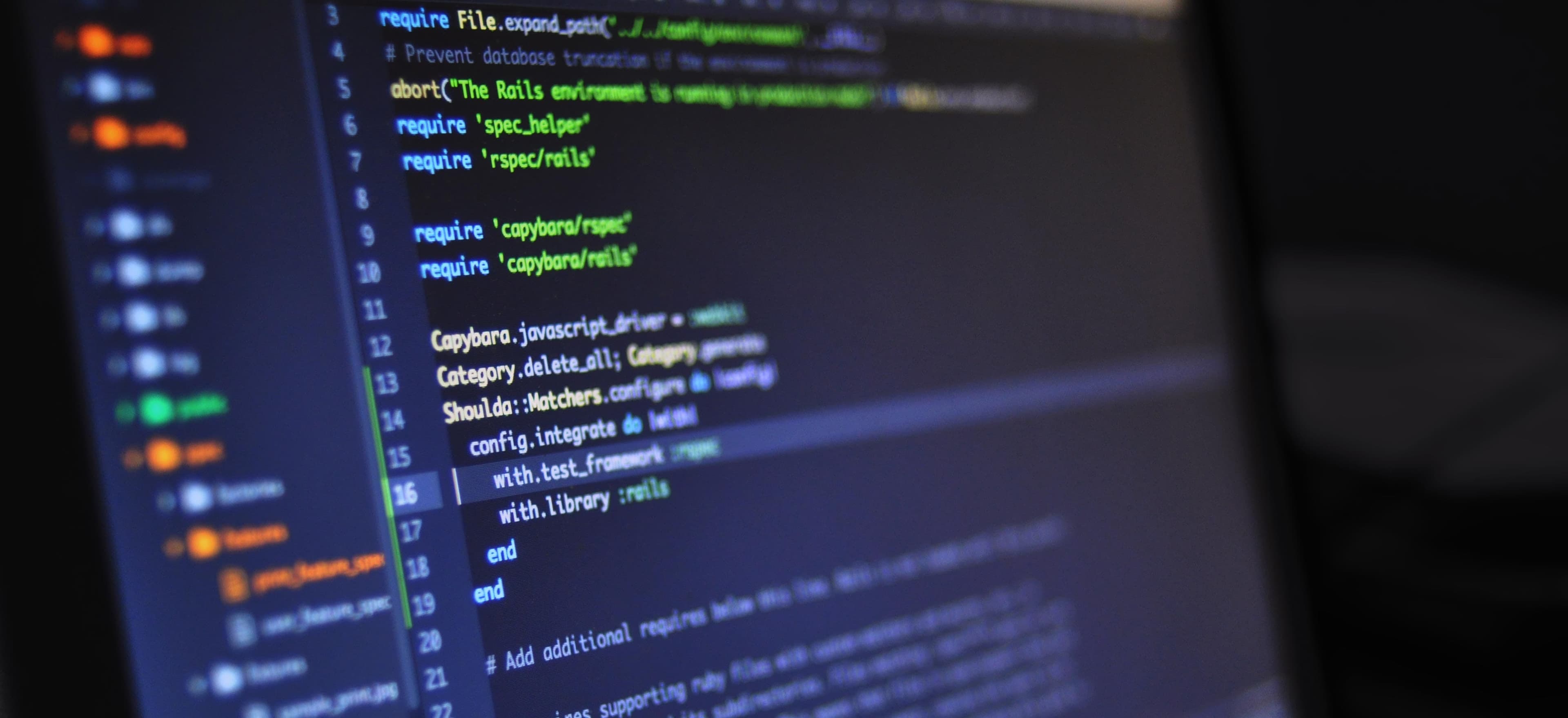
- Published on
Understanding the FilesystemMap in Python: A Comprehensive Guide
When working with file systems in Python, it's essential to have a clear understanding of how to interact with the files and directories present. The fsmap
module in Python provides a powerful and intuitive way to work with file systems, offering a range of functionalities for managing, navigating, and manipulating files and directories.
What is FilesystemMap?
The fsmap
module in Python allows us to create a logical representation of the file system, essentially mapping the structure of directories and files within a Python object. This enables seamless interaction with the files and directories, providing the ability to perform operations such as reading, writing, and deleting files, creating directories, and navigating through the file system.
Getting Started with FilesystemMap
To begin using the fsmap
module, it first needs to be installed using pip:
pip install fsmap
Once installed, the module can be imported into your Python script:
import fsmap
Creating a FilesystemMap
Creating a FilesystemMap
object is the initial step in working with the file system. This can be achieved by providing the root path of the file system you want to map:
fs = fsmap.FilesystemMap('/path/to/root')
This creates a FilesystemMap
object, representing the file system rooted at the specified path.
Navigating the FilesystemMap
The FilesystemMap
offers various methods for navigating through the file system. One such method is listdir
, which lists the contents of a directory:
contents = fs.listdir('/path/to/directory')
print(contents)
This will retrieve and print the contents of the specified directory within the mapped file system.
Accessing File Contents
To read the contents of a file within the mapped file system, the read_file
method can be used:
file_contents = fs.read_file('/path/to/file.txt')
print(file_contents)
Here, the contents of the specified file are read and printed.
Modifying FilesystemMap
The FilesystemMap
provides functionality for modifying the file system, such as creating new directories or files and deleting existing ones. For instance, to create a new directory:
fs.mkdir('/path/to/new/directory')
This creates a new directory within the mapped file system.
Integrating FilesystemMap with Existing Code
One of the key advantages of using fsmap
is its seamless integration with existing code that interacts with the file system. By employing FilesystemMap
to represent the file system, existing code can be adapted to utilize the mapped representation, enabling enhanced control and management of the file system without requiring significant changes to the existing codebase.
Closing Remarks
In conclusion, the fsmap
module in Python provides a powerful and flexible way to work with file systems, offering a range of functionalities for managing files and directories. By creating a logical representation of the file system, it simplifies the process of interacting with the file system, enabling developers to perform operations with ease and efficiency.
To delve further into the capabilities of fsmap
, the official documentation here offers comprehensive insights and examples.
Incorporating fsmap
into your Python projects can elevate the ease and efficiency with which the file system is managed, making it an invaluable tool for developers across various domains.