Taming Event Time in Apache Flink: Key Challenges Explained
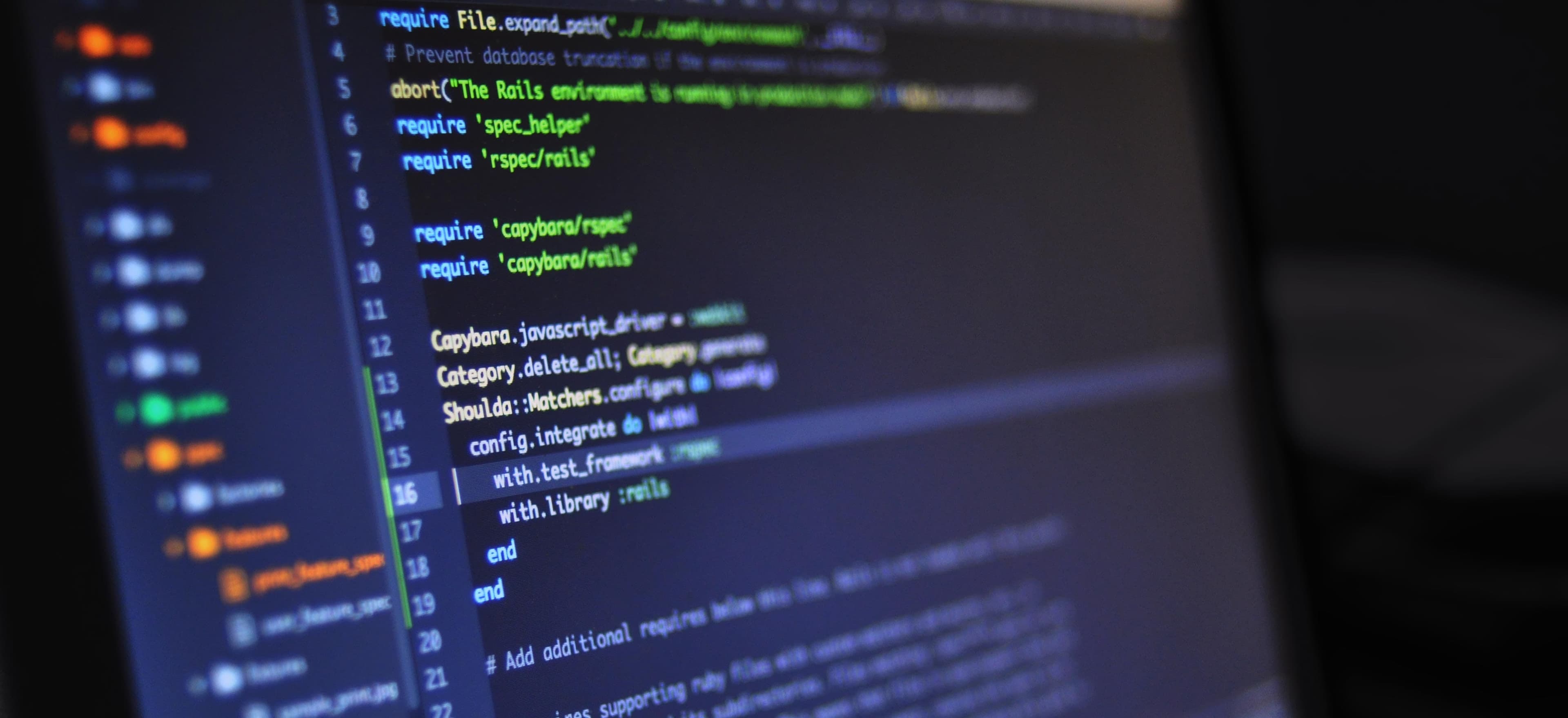
- Published on
Taming Event Time in Apache Flink: Key Challenges Explained
Apache Flink is a powerful stream processing framework that facilitates the processing of real-time data streams with remarkable performance. One of the core aspects of Flink is its ability to handle event-time processing, which allows you to account for out-of-order events and late arrivals. However, working with event time can be challenging. This blog post will guide you through these challenges and how to address them effectively with Apache Flink.
Understanding Event Time
Before diving into the challenges, let's clarify what we mean by event time. In streaming applications, events may not arrive in the order they were generated. Event time refers to the time at which the event occurred, as opposed to processing time, which is the time at which the event is processed by the system.
Example: Consider a temperature sensor that reports its readings. The sensor generates data points at different times, say:
- Event 1: 10:00 AM - 25C
- Event 2: 10:02 AM - 26C
- Event 3: 10:01 AM - 24C
Notice that Event 3 arrived after Event 2, even though it was generated earlier. Relying solely on processing time could lead to inaccurate results.
Why Is Event Time Important?
Handling event time correctly is crucial for aggregations, windowing operations, and performing time-based queries accurately. It ensures that your computations are based on when events actually happened rather than when they were processed.
Key Challenges in Event Time Processing
1. Out-of-Order Events
One common challenge in real-time data processing is the anomaly of out-of-order events. Events may arrive late, or in unexpected sequences due to network latency, system failures, and other reasons.
Solution: Watermarks Flink uses watermarks to track the progress of event time. A watermark signifies that no events with a timestamp less than or equal to the watermark will arrive.
Here's a simple code snippet demonstrating how to set a watermark strategy in Flink:
import org.apache.flink.streaming.api.environment.StreamExecutionEnvironment;
import org.apache.flink.streaming.api.datastream.DataStream;
import org.apache.flink.streaming.api.functions.timestamps.WatermarkStrategy;
public class EventProcessing {
public static void main(String[] args) throws Exception {
final StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
DataStream<Event> eventStream = env.fromElements(new Event(1, 1000L),
new Event(2, 1500L), new Event(3, 1200L));
// Set watermark strategy to handle late events
eventStream
.assignTimestampsAndWatermarks(
WatermarkStrategy
.forBoundedOutOfOrderness(Duration.ofMillis(300))
.withTimestampAssigner((event, timestamp) -> event.getTimestamp()))
// Further processing
.print();
env.execute("Event Time Processing with Watermarks");
}
}
Why? The code assigns timestamps to events while allowing for a small amount of out-of-order event time. The watermark signifies that events later than 300 milliseconds past the maximum observed timestamp are considered stale.
For more detailed information on watermarks, you can refer to the official Flink documentation here: Watermarks in Flink.
2. Late Events
Even with watermarks, late events may still slip through. Defining a strategy for handling these late events is crucial.
Solution: Side Outputs Flink allows you to route late events to side outputs for further handling. Here's how you can implement side outputs:
import org.apache.flink.streaming.api.environment.StreamExecutionEnvironment;
import org.apache.flink.streaming.api.datastream.DataStream;
import org.apache.flink.streaming.api.datastream.OutputTag;
import org.apache.flink.streaming.api.functions.timestamps.WatermarkStrategy;
public class LateEventHandler {
private static final OutputTag<Event> lateOutputTag = new OutputTag<Event>("late-events") {};
public static void main(String[] args) throws Exception {
final StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
DataStream<Event> eventStream = env.fromElements(new Event(1, 1000L),
new Event(2, 1500L), new Event(3, 1200L));
DataStream<Event> processedStream = eventStream
.assignTimestampsAndWatermarks(
WatermarkStrategy
.forBoundedOutOfOrderness(Duration.ofMillis(300))
.withTimestampAssigner((event, timestamp) -> event.getTimestamp()))
.process(new KeyedProcessFunction<Integer, Event, Event>() {
@Override
public void processElement(Event event, Context ctx, Collector<Event> out) throws Exception {
// Check if the event is late
if (ctx.timestamp() > event.getTimestamp()) {
ctx.output(lateOutputTag, event);
} else {
// Process the event normally
out.collect(event);
}
}
});
// Accessing late events
DataStream<Event> lateEvents = processedStream.getSideOutput(lateOutputTag);
// Further processing
processedStream.print();
lateEvents.print();
env.execute("Late Event Handling with Side Outputs");
}
}
Why? The above code snippet illustrates how to monitor and manage late events by directing them to a side output. This allows for the proper handling of such events instead of dropping them outright.
3. Event-Time Windows
Managing windows in event-time processing can be complex, particularly with late and out-of-order events. You need to determine how to treat windows that can't complete due to delayed events.
Solution: Allowed Lateness Flink allows you to set an "allowed lateness" parameter when defining event-time windows.
import org.apache.flink.streaming.api.environment.StreamExecutionEnvironment;
import org.apache.flink.streaming.api.datastream.DataStream;
import org.apache.flink.streaming.api.windowing.time.Time;
import org.apache.flink.streaming.api.windowing.assigners.TimeWindows;
public class WindowingExample {
public static void main(String[] args) throws Exception {
final StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
DataStream<Event> eventStream = env.fromElements(new Event(1, 1000L),
new Event(2, 1500L), new Event(3, 1200L));
eventStream
.keyBy(Event::getKey)
.window(Time.seconds(5))
.allowedLateness(Time.seconds(2))
.sum("value")
.print();
env.execute("Event-Time Windowing with Allowed Lateness");
}
}
Why? By using allowed lateness, you permit events to be considered for processing even after the window has closed but before the specified lateness period has expired. This feature is essential for ensuring you account for events that arrive later than expected.
The Last Word
In conclusion, handling event time effectively in Apache Flink comes with its own set of challenges including out-of-order events, late events, and proper management of event-time windows. By strategically using watermarks, side outputs, and allowed lateness, you can ensure accurate event-time processing.
To further enhance your understanding of Apache Flink, consider exploring additional resources or communities, such as the Apache Flink User Mailing List for practical insights and troubleshooting help.
With the strategies outlined in this post, you can pave your way toward mastering event-time processing in Apache Flink, ultimately leading to more reliable data streaming applications.