Tackling Date & Float Binding Problems in Java JDBC
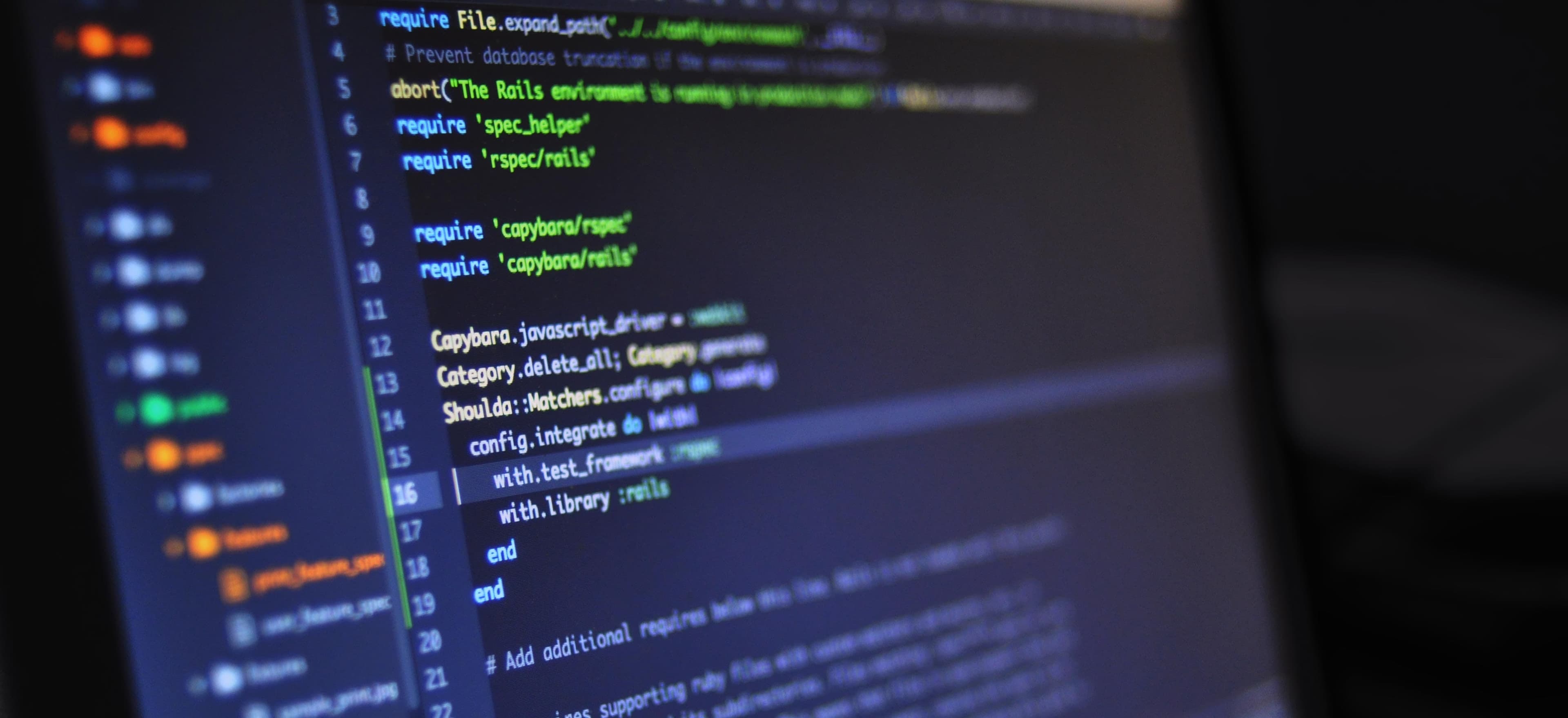
- Published on
Tackling Date & Float Binding Problems in Java JDBC
When it comes to database interactions in Java, JDBC (Java Database Connectivity) stands out as a powerful API. However, developers often encounter challenges, especially when dealing with special data types like dates and floats. This blog post aims to provide a comprehensive overview of how to effectively handle these data types in Java JDBC, alleviating problems that can arise during binding. We'll discuss best practices, pitfalls, and offer code snippets illustrating these concepts.
Understanding Data Types in JDBC
Before delving into solutions, it’s essential to understand how JDBC handles various data types. JDBC utilizes the java.sql
package, which includes classes designed to represent SQL types. Noteworthy among these are:
java.sql.Date
: Represents SQL DATE type.java.sql.Time
: Represents SQL TIME type.java.sql.Timestamp
: Represents SQL TIMESTAMP type.java.lang.Float
: Represents SQL FLOAT type.
Each of these classes serves a critical role in ensuring that data is communicated between Java applications and databases efficiently and accurately.
Common Issues with Date and Float Binding
Though JDBC provides essential interfaces for handling these data types, binding issues frequently occur. For instance, improper handling of date formats can lead to SQL exceptions, and floats may produce unexpected outputs due to precision loss.
To grasp these issues, let's examine the most prevalent pitfalls:
-
Date Formatting Confusion: SQL databases may require specific date formats. If the Java application format does not match, it leads to binding errors.
-
Precision Loss in Floats: Floats can behave unexpectedly due to imprecise calculations, which may affect how data is stored and retrieved in the database.
To overcome these challenges, we need to adopt good practices and utilize JDBC's capabilities effectively.
Binding Dates in JDBC
Let's first look into binding a date to a prepared statement. Here's a step-by-step guide with an illustrative code snippet.
Code Example: Binding Dates
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.sql.Date;
public class DateBindingExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/testdb";
String username = "root";
String password = "password";
String sql = "INSERT INTO events (event_name, event_date) VALUES (?, ?)";
try (Connection conn = DriverManager.getConnection(url, username, password);
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, "Java Conference");
// Correctly bind DATE
Date eventDate = Date.valueOf("2023-09-30");
pstmt.setDate(2, eventDate);
pstmt.executeUpdate();
System.out.println("Event inserted successfully.");
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Commentary on Date Binding
In the above example, we create a date object utilizing the Date.valueOf("yyyy-MM-dd")
method. Ensuring the format matches the SQL standard is crucial for smooth operations. If you receive a date in a different format from the user, you may need to convert it accordingly using a SimpleDateFormat
prior to binding.
Handling Float Data Types in JDBC
Floats can also present complexities, especially when high precision is a concern. Below is a code snippet to handle binding of float values correctly.
Code Example: Binding Floats
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class FloatBindingExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/testdb";
String username = "root";
String password = "password";
String sql = "INSERT INTO measurements (item_name, item_value) VALUES (?, ?)";
try (Connection conn = DriverManager.getConnection(url, username, password);
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, "Temperature");
// Correctly bind FLOAT
float itemValue = 25.75f; // Use float data type
pstmt.setFloat(2, itemValue);
pstmt.executeUpdate();
System.out.println("Measurement inserted successfully.");
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Commentary on Float Binding
In the code above, we use the pstmt.setFloat(2, itemValue)
method to bind the float. It's crucial to note that while floats are acceptable, for even higher precision requirements, it's often better to use double
and pstmt.setDouble()
for value binding. This ensures more accurate representation in databases especially where precision can significantly alter data outcomes.
Best Practices for Binding Data in JDBC
While being aware of binding techniques is fundamental, following best practices enhances your code's reliability and maintainability:
-
Utilize Prepared Statements: Always use prepared statements, as they not only protect against SQL injection but also streamline data binding.
-
Handle Exceptions Gracefully: SQL exceptions must be caught effectively to ensure your application does not fail silently. Logging errors can help in debugging.
-
Employ Object-Relational Mapping (ORM): Libraries like Hibernate can abstract these issues, handling conversions seamlessly.
-
Stay Updated on SQL Standards: Regularly check if your SQL database has any updates or changes in data binding requirements.
-
Refer to Established Resources: If you encounter specific issues, leverage existing resources for solutions. For instance, you can refer to the article on Fix PDO BindParam Issues with MySQL Date & Float Types for insights when transitioning concepts from PHP PDO to JDBC.
Key Takeaways
Handling date and float binding issues in Java JDBC can be straightforward with the right understanding and practices. By recognizing common pitfalls and utilizing proper binding methods, developers can ensure robust application performance when interacting with SQL databases.
JDBC provides a solid foundation for database interactions, but awareness of these nuances can save a great deal of time and frustration. Remember to follow best practices, utilize community resources, and stay aware of updates in your database systems.
For any ongoing development project, incorporating these lessons can significantly improve data accuracy and reliability in your Java applications. Happy coding!
Checkout our other articles