Java Solutions for Managing Your Financial Data Efficiently
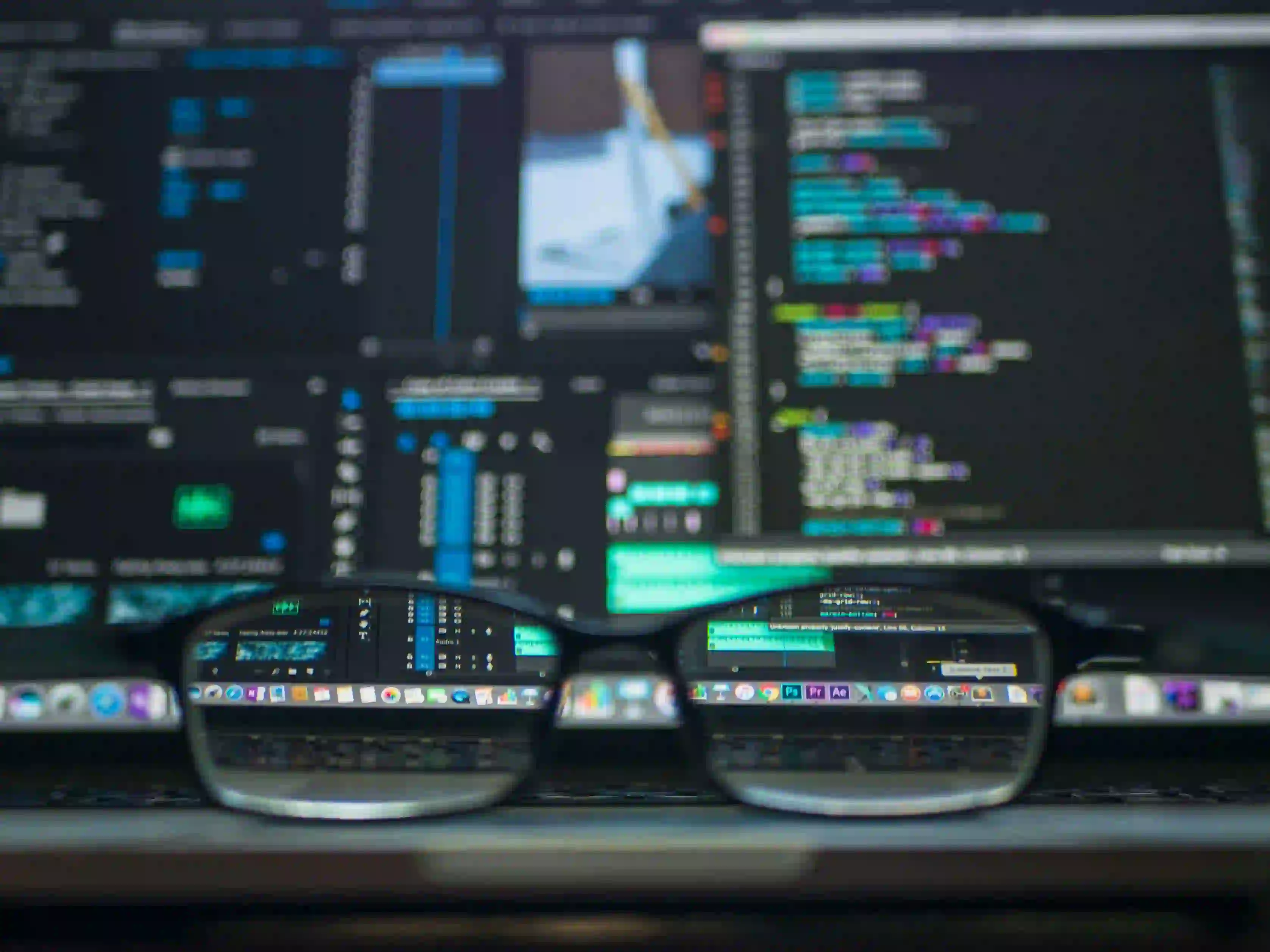
Java Solutions for Managing Your Financial Data Efficiently
In our digital age, the ability to efficiently manage financial data is paramount. Java, a powerful object-oriented programming language, offers a robust set of tools and libraries that can simplify this task. Whether you're developing a complex financial application or a simple personal budgeting tool, Java provides numerous solutions that enhance data handling and processing.
In this blog post, we’ll explore some effective Java solutions for managing your financial data, including data structures, libraries, and frameworks that handle financial calculations, data storage, and reporting. We’ll also incorporate insights related to cash management, aligning with the principles discussed in the article "Maximizing Your Cash Reserves: A Prepper's Guide" found at youvswild.com.
1. Choosing the Right Data Structure
When managing financial data, selecting an appropriate data structure can significantly impact the performance and readability of your application. Here are some common data structures used in financial applications:
Arrays and ArrayLists
Arrays are ideal for a fixed-size collection of data. For example, a simple budget may store monthly expenses like this:
double[] monthlyExpenses = new double[12]; // 12 months of expenses
However, if expenses can vary in number, using an ArrayList
is more efficient:
import java.util.ArrayList;
ArrayList<Double> expenses = new ArrayList<>();
expenses.add(300.00); // Add expenses dynamically
Why Use ArrayLists?
ArrayLists are resizable, offering dynamic memory handling that is crucial for applications where the number of data points isn't known upfront.
2. Handling Financial Calculations
Financial applications often require precise calculations involving currency, percentages, and time. For this, Java provides the BigDecimal
class.
Example: Interest Calculation
import java.math.BigDecimal;
import java.math.MathContext;
public class FinancialCalculator {
public static BigDecimal calculateInterest(BigDecimal principal, BigDecimal rate, int time) {
BigDecimal interest = principal.multiply(rate).multiply(new BigDecimal(time));
return interest.divide(new BigDecimal(100), MathContext.DECIMAL128); // Ensuring precision
}
}
// Usage
BigDecimal principal = new BigDecimal("1000.00");
BigDecimal rate = new BigDecimal("5.00");
int time = 2;
BigDecimal interest = FinancialCalculator.calculateInterest(principal, rate, time);
System.out.println("Interest earned: " + interest);
Why Use BigDecimal?
BigDecimal handles high-precision calculations, important for financial applications where rounding errors can lead to significant discrepancies.
3. Data Persistence with Databases
JDBC for Database Operations
Java Database Connectivity (JDBC) allows applications to connect and execute queries on databases, making it perfect for storing and retrieving financial data. Below is a basic JDBC setup.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class DatabaseManager {
private static final String URL = "jdbc:mysql://localhost:3306/yourDatabase";
private static final String USER = "username";
private static final String PASSWORD = "password";
public Connection connect() throws Exception {
return DriverManager.getConnection(URL, USER, PASSWORD);
}
public void insertExpense(double amount, String category) throws Exception {
String query = "INSERT INTO expenses (amount, category) VALUES (?, ?)";
try (Connection conn = connect();
PreparedStatement stmt = conn.prepareStatement(query)) {
stmt.setDouble(1, amount);
stmt.setString(2, category);
stmt.executeUpdate();
}
}
public void getExpenses() throws Exception {
String query = "SELECT * FROM expenses";
try (Connection conn = connect();
PreparedStatement stmt = conn.prepareStatement(query);
ResultSet rs = stmt.executeQuery()) {
while (rs.next()) {
System.out.println("Expense: " + rs.getDouble("amount") +
", Category: " + rs.getString("category"));
}
}
}
}
// Usage
DatabaseManager dbManager = new DatabaseManager();
dbManager.insertExpense(150.00, "Groceries");
dbManager.getExpenses();
Why Use JDBC?
JDBC provides a standard interface for interacting with relational databases, crucial for reliable data management and retrieval in financial applications.
4. Reporting and Visualization
Once data is stored and processed, reporting is essential. Java libraries such as JasperReports can create dynamic reports from your financial data.
Integrating JasperReports
Below is a brief example of how one might set up a report.
import net.sf.jasperreports.engine.*;
import java.util.HashMap;
public class ReportGenerator {
public void generateExpenseReport() throws Exception {
JasperReport report = JasperCompileManager.compileReport("expenseReport.jrxml");
HashMap<String, Object> parameters = new HashMap<>();
// Add any parameters required for the report
JasperPrint print = JasperFillManager.fillReport(report, parameters, new JRBeanCollectionDataSource(getExpenseData()));
JasperExportManager.exportReportToPdfFile(print, "ExpenseReport.pdf");
}
private List<Expense> getExpenseData() {
// Populate your data from the database or other sources here
return new ArrayList<>(); // Placeholder
}
}
Why Use a Reporting Library?
Using dedicated reporting libraries allows developers to create unified visual representations of financial data, aiding in decision-making processes.
5. Incorporating Best Practices for Financial Management
While coding and tools are vital, having effective financial management strategies is equally important. In light of the article "Maximizing Your Cash Reserves: A Prepper's Guide", integrating lessons from effective financial practices can be beneficial.
- Budgeting: Use your applications to set budgets for various categories.
- CSV Exports: Develop functionalities to export data in formats like CSV for easy sharing and analysis.
- Regular Reports: Automate regular expense reports to ensure you are on track with your financial goals.
For further insights on managing your finances and improving your cash reserves, check out the detailed strategies shared at youvswild.com.
Lessons Learned
Managing financial data efficiently with Java encompasses various aspects including choosing the right data structure, utilizing libraries for financial calculations, connecting with databases, and creating reports. As you develop your financial applications, keep in mind the principles discussed here, alongside those found in "Maximizing Your Cash Reserves: A Prepper's Guide". By leveraging Java's robust features, you can create a complete and efficient financial management tool that assists you in achieving your financial goals.
Additional Resources
With these tools and techniques at your disposal, you'll be well-equipped to tackle any financial management challenge using Java. Happy coding!