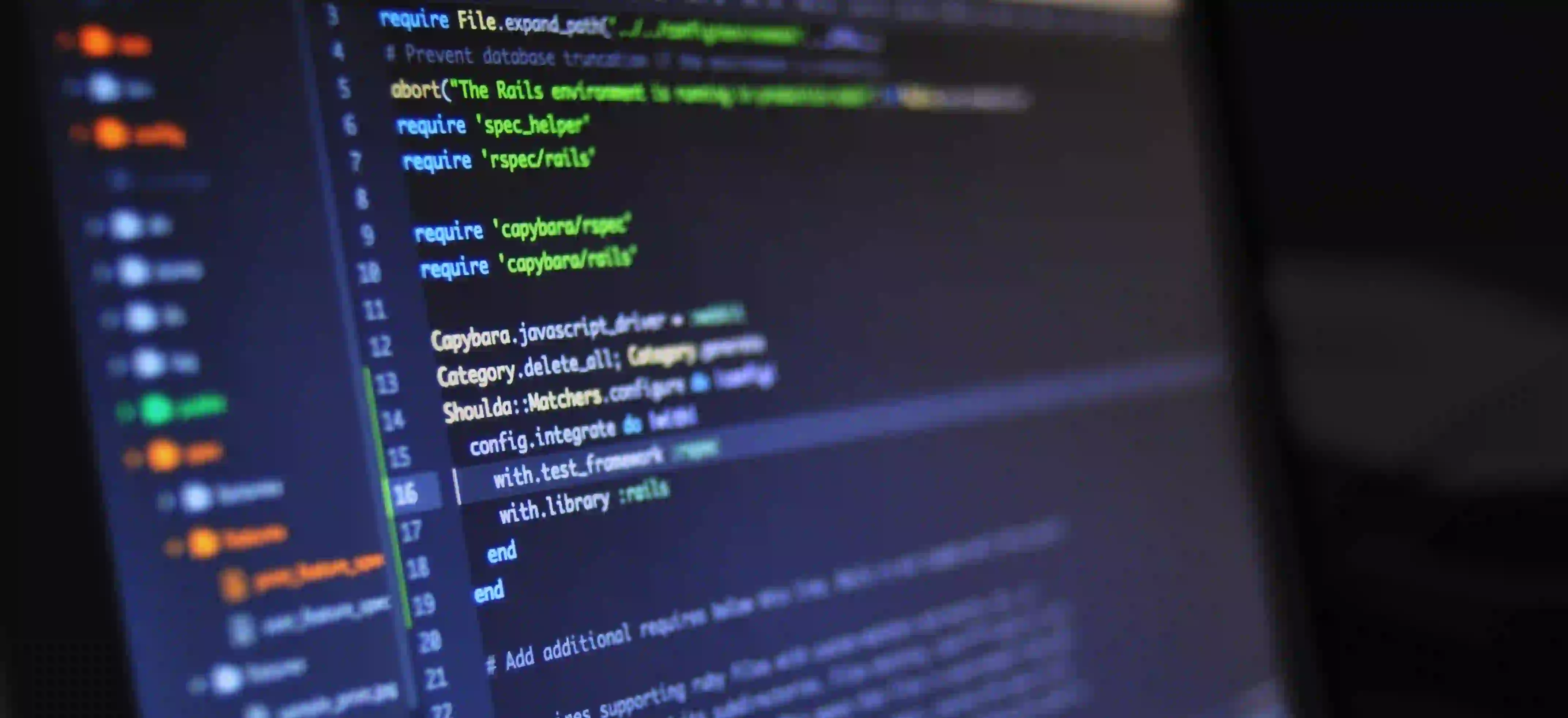
Eliminate GIF Play Buttons in Java Web Apps for Better UX
In the ever-evolving world of web development, user experience (UX) stands at the forefront of considerations. With the growing importance of accessibility, developers are tasked with making web applications more user-friendly. One common pain point in user interfaces, especially in Java web apps, is the presence of GIF play buttons. While they can add vibrancy to websites, they can also hinder user experience, particularly for those using accessibility tools.
In this blog post, we will explore how to eliminate GIF play buttons in your Java web applications. We will cover the technical aspects, discuss the implications for accessibility, and guide you through code snippets that facilitate this process. If you're keen on enhancing user experiences and ensuring accessibility, you've landed on the right page.
Understanding the Challenge
The issue with GIF play buttons primarily revolves around accessibility. Users of assistive technologies may struggle with GIFs that automatically play or require a click to start. This can lead to confusion or a less-than-ideal interaction with your application. By removing unnecessary play buttons, you pave the way for a smoother experience.
To delve deeper into accessibility's significance, consider reading the article titled "Boost Accessibility: Hide GIF Play Buttons With Ease", which discusses effective techniques for web developers.
Why Eliminate GIF Play Buttons?
-
Improve Navigation: Users with cognitive disabilities may have a harder time comprehending which elements can be interacted with. By eliminating play buttons, the interface becomes straightforward.
-
Reduce Clutter: GIF play buttons can overcrowd your UI. A cleaner design often leads to better usability.
-
Enhance Performance: Fewer interactive elements can lead to improved loading times and performance metrics.
-
Accessibility Compliance: Striving for more inclusive designs boosts your website's compliance with standards like the Web Content Accessibility Guidelines (WCAG).
Getting Started
Before we start writing code, it's important to set up our Java web application. Make sure you have the Java Development Kit (JDK) installed, and that you're using a modern web framework such as Spring Boot or Jakarta EE to build your web application.
Elimination via CSS
A straightforward way to remove GIF play buttons is by using CSS to hide it from the view. You can target the specific elements that correspond to the play buttons and set their display
property to none
.
/* CSS to hide GIF play buttons */
.play-button {
display: none; /* Hides the play button */
}
Why This Works: By targeting the button class in the CSS, the play button becomes invisible to all users. This approach simplifies the user interface while remaining non-intrusive.
Implementation in Java Web Applications
For a concrete example, let’s assume you have a Java web application using a server-side templating engine like JSP (JavaServer Pages). Here’s how you might implement the CSS alongside your Java code.
Step 1: Create Your HTML Structure
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>GIF Example</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="gif-container">
<img src="path_to_your_gif.gif" alt="Description of GIF" />
<button class="play-button">Play</button> <!-- This will be hidden -->
</div>
</body>
</html>
Step 2: Apply the CSS
As outlined previously, you will add the following CSS to your stylesheet:
.play-button {
display: none; /* Hides the play button */
}
Dynamically Generated Content
In many Java applications, content can be dynamically generated. Let's consider how we could achieve the same result with a Java backend.
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@WebServlet("/displayGif")
public class GifDisplayServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setAttribute("gifPath", "path_to_your_gif.gif");
request.getRequestDispatcher("/displayGif.jsp").forward(request, response);
}
}
And the corresponding JSP page:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<link rel="stylesheet" type="text/css" href="styles.css">
</head>
<body>
<div class="gif-container">
<img src="${gifPath}" alt="Description of GIF" />
<button class="play-button">Play</button> <!-- This button will be hidden -->
</div>
</body>
</html>
Why Use Dynamic Content: This method enables you to serve GIFs efficiently and keep your code clean and manageable. By controlling the GIF dynamically, you can apply more complex transformations later if needed.
Leveraging JavaScript
If you need to maintain play buttons for other settings, JavaScript can programmatically hide or remove them on the fly.
document.addEventListener("DOMContentLoaded", function() {
const playButton = document.querySelector('.play-button');
if (playButton) {
playButton.style.display = 'none'; // Hides the play button
}
});
Why This Matters: Adding a JavaScript solution allows you to control the DOM dynamically, promoting greater interactivity while still maintaining user-focused design concepts.
Best Practices
-
Test for Accessibility: Always use accessibility testing tools to evaluate your application's performance. It's crucial to confirm that removing elements has not negatively impacted usability.
-
Optimize GIF Usage: Minimize the number of GIFs used in your application. Focus on quality over quantity to avoid clutter and confusion.
-
User Feedback: Encourage user feedback on the changes you implement. This can provide invaluable insights and help you enhance UX further.
Lessons Learned
Eliminating GIF play buttons may seem like a small change, but it can have a significant impact on user experience. By simplifying your Java web applications and ensuring they are accessible, you set your users up for success, creating a more inclusive digital landscape.
If you're interested in learning more about accessibility in web development, don't forget to check out "Boost Accessibility: Hide GIF Play Buttons With Ease". Embrace best practices today, and watch your web applications thrive.
Through these efforts, you not only enhance user experience but also affirm your commitment to inclusive design. Start implementing these techniques and observe the difference they make!