Enhancing Java Web Apps: Fixing Faded Nav-Link Colors
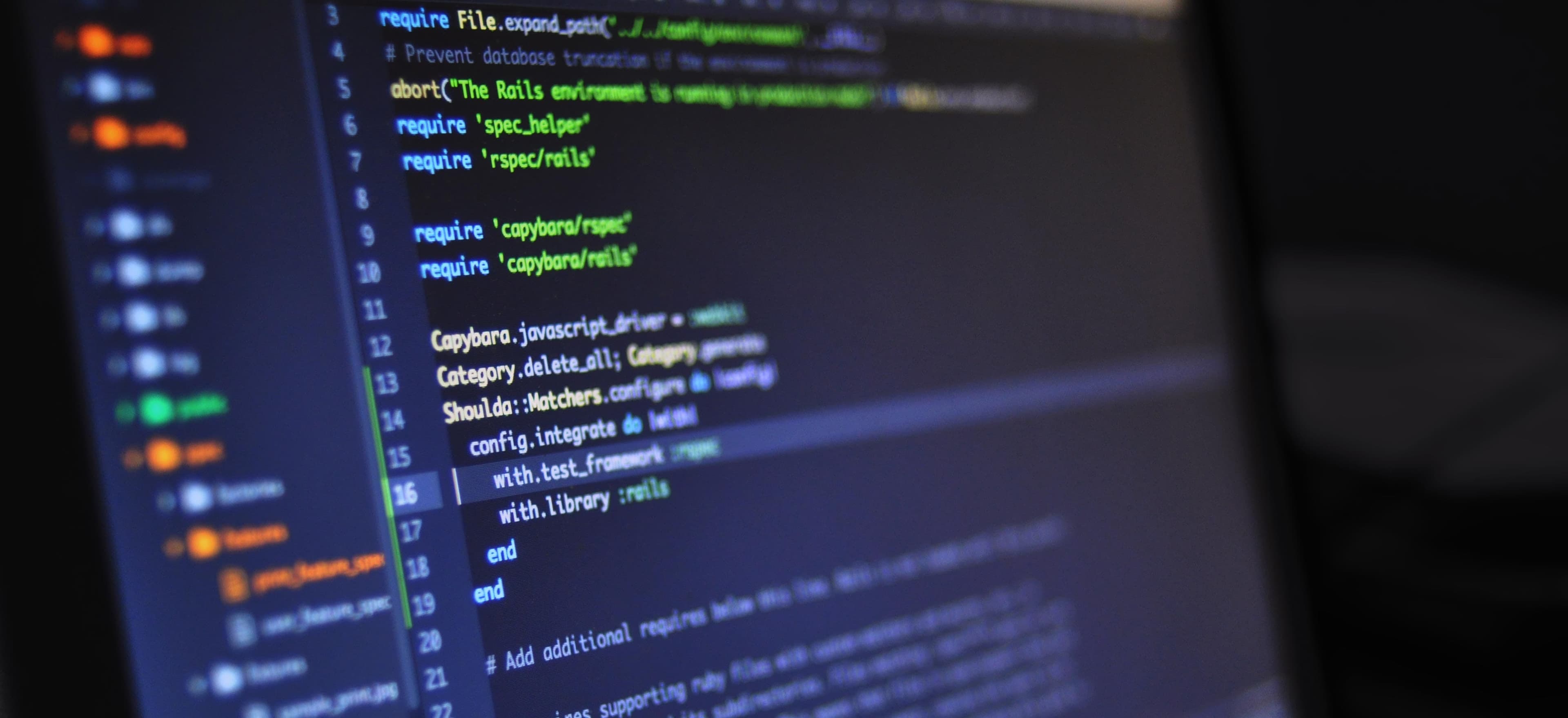
- Published on
Enhancing Java Web Apps: Fixing Faded Nav-Link Colors
In the world of web application development, user experience is paramount. A crucial aspect of this experience comes from the visual components of your application, such as navigation links. If your website's navigation links appear faded or do not stand out, it can lead to a poor user experience. This blog post will discuss how to fix faded nav-link colors in Java web applications and ensure that your site's navigation is clear and engaging.
Understanding the Importance of Navigation Links
Navigation links serve as the backbone of any web application. They guide users through services and content, ensuring a seamless experience. Faded or poorly colored links can signal unresponsiveness or neglect and may discourage visitors from exploring your site. Thus, ensuring your nav-link colors are vibrant and recognizable is essential.
Where the Problem Lies
Faded nav-link colors typically stem from CSS issues rather than Java itself. Despite this, Java developers often encounter these problems within frameworks that integrate CSS styling. For example, when using frameworks such as Spring or JavaServer Faces (JSF), it's easy for CSS to override your intended color styles.
The Root Causes
- CSS Overriding: Colors defined in your CSS can be unintentionally overridden by other styles.
- Browser Incompatibilities: Some CSS properties may not render consistently across different browsers.
- Poor Design Contrast: Colors might be too similar to the background, making them hard to see.
To tackle this issue effectively, we will use CSS to enhance the colors of navigation links while maintaining a clean, professional look.
Fixing Faded Nav-Link Colors in CSS
First, ensure that you have established a CSS file linked to your HTML. Here’s how you can set up the colors for your navigation links:
Example CSS
.nav-link {
color: #007BFF; /* A vibrant blue for the links */
text-decoration: none; /* Removes underlines */
transition: color 0.3s; /* Smooth transition on hover */
}
.nav-link:hover {
color: #0056b3; /* Darker blue on hover */
}
.nav-link:visited {
color: #6c757d; /* Muted color for visited links */
}
.nav-link:active {
color: #004085; /* Even darker for clicked links */
}
Commentary on the Code
- The
.nav-link
class begins with a clear and vibrant blue (#007BFF
), creating immediate recognition. - The use of
text-decoration: none;
ensures that links are not underlined, presenting a cleaner UI. - The
transition: color 0.3s;
property enhances the user experience by providing visual feedback when users hover. - Having different states (
hover
,visited
, andactive
) gives users further context on their interaction with links.
These modifications not only improve visibility but also contribute to the overall aesthetic of your application.
Ensuring Consistency: CSS Specificity
One of the common pitfalls of styling link colors is CSS specificity. If your styles are being overridden, you need to ensure your selectors are specific enough.
Example of Overriding
Suppose you have the following HTML structure:
<nav>
<ul>
<li><a href="#" class="nav-link">Home</a></li>
<li><a href="#" class="nav-link">About</a></li>
<li><a href="#" class="nav-link">Services</a></li>
</ul>
</nav>
If there’s a more generic selector, such as:
a {
color: gray;
}
This will override your .nav-link
color. To fix this, you can increase specificity:
nav .nav-link {
color: #007BFF;
}
This ensures that your intended styles take precedence, giving you more control over the appearance.
Testing Across Different Browsers
Once you've made your changes, it's critical to test your styles across multiple browsers. Browsers can behave differently, and a style that works in Chrome might not render exactly the same in Firefox or Safari.
- Use Browser Developer Tools: Most browsers have built-in developer tools to test CSS changes quickly.
- Cross-Browser Testing Tools: Platforms like BrowserStack allow you to see how your site performs on various devices.
Integrating Dynamic Features with Java
For Java-based frameworks that dynamically generate your HTML (like JSF), you can use backend logic to control link behavior. For example, you can conditionally render color classes based on user login status or page status.
Example in JSF
<h:outputLink value="/home" styleClass="#{userBean.isLoggedIn ? 'nav-link logged-in' : 'nav-link'}">
Home
</h:outputLink>
This example uses a conditional expression to apply different CSS classes based on the user state. This leads to better visual cues for users, enhancing their experience.
Addressing Accessibility Issues
In addition to aesthetics, consider accessibility. It’s vital that your nav-links are not only visually appealing but also accessible:
- Contrast Ratios: Ensure that your text color and background color have a sufficient contrast ratio. Use tools like the WebAIM Contrast Checker to verify.
- Semantic HTML: Utilize proper HTML elements (
<nav>
,<ul>
,<li>
, etc.) to guarantee screen readers can interpret your links correctly.
Lessons Learned
In summary, fixing faded nav-link colors in Java web applications requires a comprehensive understanding of CSS and browser behaviors. By implementing vibrant colors, ensuring specificity in your CSS, conducting comprehensive testing, and integrating Java dynamic features, you can dramatically enhance the user experience.
For further information on tackling CSS-related issues, you might find the article "How to Solve Faded Nav-Link Colors in Your CSS" helpful. It addresses common pitfalls and solutions for CSS styling, which can be beneficial when designing your web application's user interface. Read more about it here: infinitejs.com/posts/solve-faded-nav-link-colors-css.
Empowering your users with a visually engaging and accessible navigation will undoubtedly keep them coming back—and that is the ultimate goal of any web application developer.
Feel free to tweak, expand, or create further discussions on the themes presented in this blog. Your feedback and queries are crucial in crafting content that resonates with the developer community. Happy coding!