Mastering Java Form Validation: A Styling Perspective
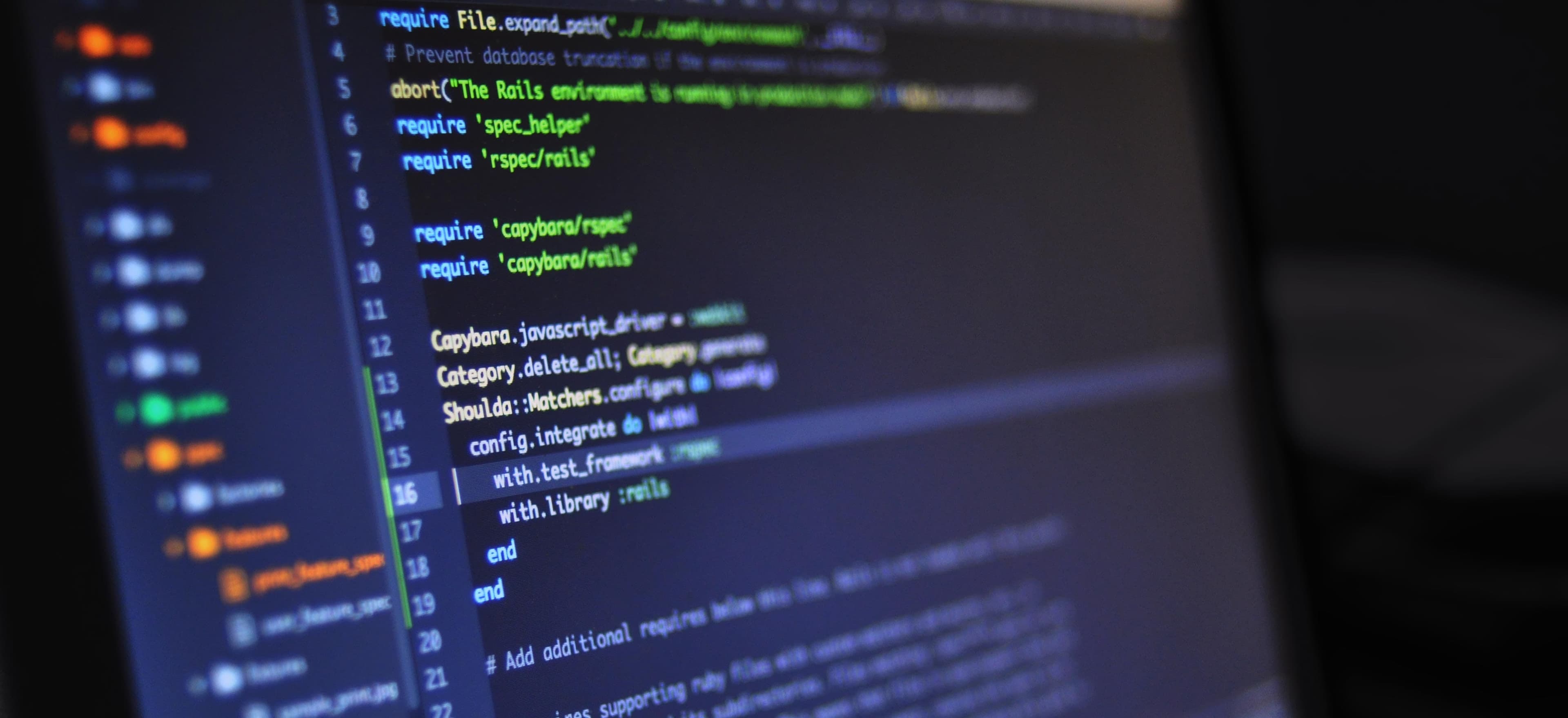
- Published on
Mastering Java Form Validation: A Styling Perspective
Form validation is an essential aspect of web development, ensuring that user input aligns with the expected parameters. While robust validation mechanisms may primarily focus on functionality, the presentation of these validations plays a significant role in user experience. In this blog post, we will delve into the intricacies of form validation in Java, emphasizing a stylish user interface while maintaining impeccable functionality.
Understanding Form Validation
Form validation serves two primary purposes:
- Data Integrity: Ensures that the data being collected is accurate and in the right format.
- User Experience: Provides real-time feedback to users, making it easier for them to understand what input is required.
In Java, form validation can be achieved through various frameworks and libraries, such as Java Server Faces (JSF) or Spring. Both frameworks provide built-in support for form validation, making it less cumbersome for developers.
Why Focus on Styling?
In modern web applications, aesthetic considerations have become as crucial as functional ones. A well-styled form not only guides users but also enhances the overall appeal of the application.
In the article titled Overcoming Styling Challenges for Form Inputs, the author explores various design challenges specific to form inputs. This excellent resource discusses numerous strategies to design forms that are both aesthetically pleasing and functional.
Java Form Validation Basics
Before we dive into styling, let’s establish how to perform validation in Java. Here’s a look at a simple Java form validation approach using JavaBeans Validation (JSR 380).
Setting Up the Validation
- Ensure you have the Hibernate Validator dependency in your Maven project:
<dependency>
<groupId>org.hibernate.validator</groupId>
<artifactId>hibernate-validator</artifactId>
<version>6.2.0.Final</version>
</dependency>
- Create a JavaBean with validation annotations:
import javax.validation.constraints.Email;
import javax.validation.constraints.NotEmpty;
import javax.validation.constraints.Size;
public class User {
@NotEmpty(message = "Username cannot be empty")
private String username;
@NotEmpty(message = "Email cannot be empty")
@Email(message = "Email should be valid")
private String email;
@Size(min = 6, message = "Password should have at least 6 characters")
private String password;
// Getters and Setters
}
Why This Matters: By utilizing annotations like @NotEmpty
, @Email
, and @Size
, you enforce rules directly on your properties. This approach simplifies your validation logic and keeps your code clean.
Validating the Bean
You would typically validate the Bean within your service or controller:
import javax.validation.ConstraintViolation;
import javax.validation.Validation;
import javax.validation.Validator;
import javax.validation.ValidatorFactory;
import java.util.Set;
public class UserService {
private Validator validator;
public UserService() {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
validator = factory.getValidator();
}
public void registerUser(User user) {
Set<ConstraintViolation<User>> violations = validator.validate(user);
if (!violations.isEmpty()) {
for (ConstraintViolation<User> violation : violations) {
System.out.println(violation.getMessage());
}
} else {
// Proceed with user registration
System.out.println("User registered successfully!");
}
}
}
Commentary: Here we initialize a Validator and use it in the registerUser
method to check for validation rules. The use of ConstraintViolation
enables us to retrieve specific feedback for the user.
Combining Functionality with Stylish Presentation
Now that we have a functional validation setup, let’s discuss how to present this data stylishly.
The Role of CSS
A clean CSS framework can drastically improve the styling of form inputs. Here we will utilize a simple CSS setup.
input {
border: 2px solid #ccc;
padding: 10px;
margin: 5px 0;
width: 100%;
box-sizing: border-box;
}
input:focus {
border-color: #007bff;
}
.error {
color: red;
font-size: 0.9rem;
}
Why CSS Matters: The above CSS creates an engaging user experience. The border changes color on focus, drawing the user’s attention to the input. Additionally, error messages are styled distinctly, thereby alerting users immediately to any issues.
Enhancing with JavaScript for Real-Time Feedback
We can further couple our Java validation with client-side feedback using JavaScript.
<script>
function validateForm() {
const username = document.getElementById("username").value;
const email = document.getElementById("email").value;
const password = document.getElementById("password").value;
if (!username) {
showError("Username cannot be empty");
return false;
}
if (!validateEmail(email)) {
showError("Email should be valid");
return false;
}
if (password.length < 6) {
showError("Password should have at least 6 characters");
return false;
}
return true;
}
function showError(message) {
const errorDiv = document.getElementById("error");
errorDiv.innerText = message;
errorDiv.classList.add("error");
}
function validateEmail(email) {
const re = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return re.test(email);
}
</script>
What to Note: By providing JavaScript for preliminary checks before form submission, we save server resources and enhance user experience. Immediate feedback helps users correct mistakes on-the-go.
Wrapping It All Up
In conclusion, Java form validation can be both functional and visually appealing. By leveraging validation annotations, CSS styling, and JavaScript, we can create forms that not only perform well but also look good.
For a more comprehensive understanding of how to style your forms effectively, revisit the article Overcoming Styling Challenges for Form Inputs. It provides invaluable insights into tackling styling hurdles in web form development.
Key Takeaways
- Validation is critical for data integrity and user experience.
- Java provides robust options for form validation through annotations.
- CSS and JavaScript can significantly enhance the usability and aesthetic of your forms.
- Striking the right balance between functionality and style is essential for modern web applications.
By paying attention to both validation and presentation, you elevate your web application beyond basic functionality to a user-friendly interface that your users will appreciate.
Happy coding!