Mastering Pagination: Common Pitfalls to Avoid
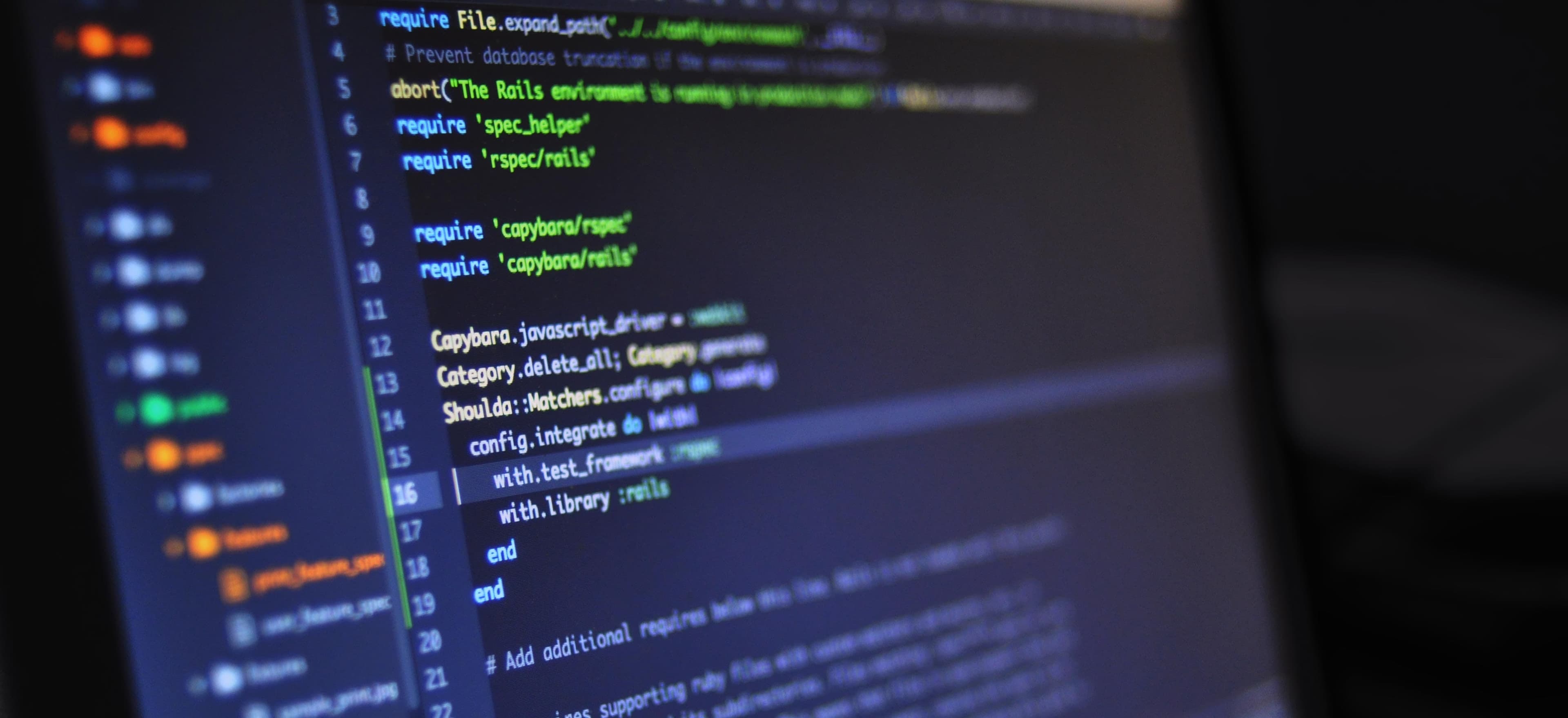
- Published on
Mastering Pagination: Common Pitfalls to Avoid
Pagination is a crucial aspect of web development and user experience. It allows the user to navigate through large sets of data without overwhelming them. However, implementing pagination isn’t as straightforward as it seems. In this blog post, we'll explore common pitfalls to avoid while mastering pagination in Java applications, enhancing both performance and user satisfaction.
What is Pagination?
Pagination is a method that divides content into discrete pages. This technique is widespread across various applications, from displaying search results to breaking down long articles. It is essential in improving user engagement and facilitating easier navigation.
Why is Pagination Important?
Pagination helps in:
- Improved User Experience: Users can easily navigate through a set of data.
- Optimized Performance: It prevents the loading of extensive data sets at once, which can consume too much bandwidth and lead to slower response times.
- SEO Benefits: Properly implemented pagination allows search engines to index your content more effectively.
Understanding the Architecture of Pagination
Before diving into pitfalls, let's recap the fundamental components of pagination.
Key Components
- Current Page: Represents the page the user is currently on.
- Total Pages: Total number of pages calculated based on the total number of items and items per page.
- Items per Page: This defines how many items will be displayed on a single page.
- Offset: The position of the first item on the current page.
Sample Java Pagination Code
Here’s a simple implementation of pagination in Java:
import java.util.List;
public class Paginator<T> {
private List<T> items;
private int itemsPerPage;
public Paginator(List<T> items, int itemsPerPage) {
this.items = items;
this.itemsPerPage = itemsPerPage;
}
public List<T> getPage(int pageNumber) {
int fromIndex = (pageNumber - 1) * itemsPerPage;
if (fromIndex >= items.size()) {
return List.of(); // Return an empty list if the page number exceeds total pages.
}
int toIndex = Math.min(fromIndex + itemsPerPage, items.size());
return items.subList(fromIndex, toIndex);
}
}
Why Use Paginator Class?
- The
Paginator
class provides a clean interface to handle pagination. - It uses generics (
<T>
) to be applied to any list type, making the paginator reusable.
Common Pitfalls in Pagination
1. Not Handling Edge Cases
When implementing pagination, it is crucial to account for edge cases.
Example Pitfall:
Failing to handle a request for a page number that exceeds the total number of pages can result in runtime errors or unexpected behavior.
Fix:
Adjust the getPage
method to handle such requests. This is already demonstrated in the code above by returning an empty list when the page number exceeds total pages.
2. Poorly Implemented Logic on the Front-End
Pagination is not only a back-end issue. The front-end must also ensure users can smoothly navigate through pages.
Example Pitfall:
Having previous and next buttons that are always enabled, regardless of the current page, can confuse users.
Fix:
Implement logic to disable these buttons appropriately. For instance:
<button id="prevBtn" disabled="true">Previous</button>
<button id="nextBtn" disabled="false">Next</button>
This provides a clear indication of which actions the user can take at any time.
3. Inconsistent API Implementation
If you are fetching data from an API, inconsistent pagination can lead to confusion.
Example Pitfall:
An API that uses different page numbering conventions (e.g., 1-based vs. 0-based indexing) can confuse developers and lead to unexpected results.
Fix:
Always document the pagination approach clearly, and follow a consistent pattern throughout your application.
4. Lack of Caching
Having to retrieve data from a database every time a user requests a page can lead to performance bottlenecks.
Example Pitfall:
High latency in data retrieval when paginating can cause a poor user experience.
Fix:
Implement search result caching with technologies like Redis or similar solutions, ensuring that repeating queries do not hit the database.
5. Not Considering SEO
When displaying paginated details, failing to provide proper SEO attributes can hurt your rankings.
Example Pitfall:
Not utilizing rel="next"
and rel="prev"
in your HTML can prevent search engines from effectively crawling your paginated content.
Fix:
Add the following attributes to your head section:
<link rel="next" href="http://example.com/items?page=2" />
<link rel="prev" href="http://example.com/items?page=1" />
This helps search engines understand how to navigate through paginated content effectively.
6. No User Control Over Pagination
Limiting users to a fixed number of items per page can lead to frustration.
Example Pitfall:
Users should be allowed to select how many items they want to see per page.
Fix:
Provide options like "Show 10, 20, 50 items per page."
7. Poor A/B Testing
Conducting various testing methods to optimize user interaction with pagination is often overlooked.
Example Pitfall:
Not examining how users are interacting with pagination can lead to perpetuating inefficiencies.
Fix:
Utilize tools like Google Analytics to analyze user behavior and test different pagination methods.
Closing Remarks
Pagination is more than just splitting content into pages. It requires a balanced approach across both front-end and back-end to improve user experience and system performance. By avoiding common pitfalls and implementing an efficient pagination system, you can significantly enhance the usability of your application.
To dive deeper into pagination strategies and best practices, feel free to check out these resources: MDN Web Docs on Pagination and Google Search Console on Pagination.
As you continue to refine your pagination techniques, remember to keep your users in mind, ensuring their experience remains smooth and enjoyable.
Happy coding!