Common Thymeleaf Validation Mistakes in Spring Applications
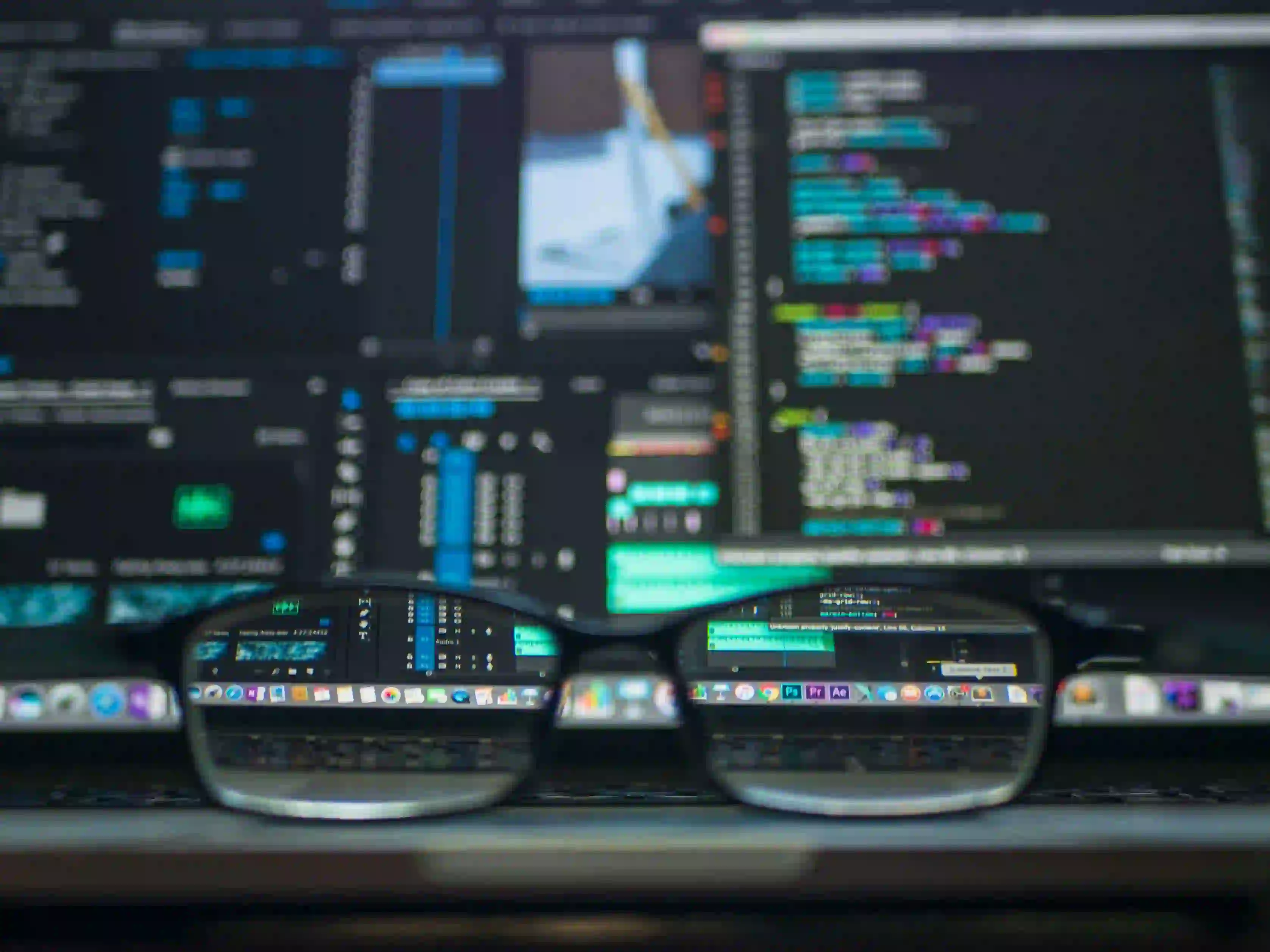
Common Thymeleaf Validation Mistakes in Spring Applications
Thymeleaf is an incredibly powerful template engine that integrates seamlessly with Spring applications. It allows developers to create dynamic web applications while keeping code clean and maintainable. However, when it comes to validation, there are several common mistakes that can hinder the effectiveness of your Thymeleaf views. In this blog post, we will discuss these pitfalls, provide practical solutions, and improve your understanding of Thymeleaf validation in Spring applications.
Understanding Thymeleaf and Spring Validation
Before delving into the common mistakes, let's establish why using Thymeleaf with Spring for validation is beneficial. Spring provides an effective validation framework that can be combined with Thymeleaf to enforce business rules within your application’s data models. Using annotations such as @Valid
or the @Validated
annotation can streamline your validation logic and ensure data integrity.
For further reading on Thymeleaf's integration with Spring, you can refer to the official Thymeleaf documentation.
Common Thymeleaf Validation Mistakes
1. Not Binding the Form Object Properly
One frequent mistake is not binding the form object to the model correctly. Failing to do so means that the Thymeleaf template cannot access the object’s properties, leading to validation errors that are hard to track.
Solution
Ensure that you are adding the form model attribute to the model correctly in your controller. Here is an example:
@Controller
@RequestMapping("/user")
public class UserController {
@GetMapping("/register")
public String showRegistrationForm(Model model) {
model.addAttribute("user", new User());
return "registration";
}
}
In your Thymeleaf template, use the model attribute correctly:
<form th:action="@{/user/register}" th:object="${user}" method="post">
<input type="text" th:field="*{username}" placeholder="Username" />
<input type="submit" value="Register" />
</form>
2. Ignoring Validation Annotations
Using Java validation annotations, such as @NotNull
or @Size
, is crucial for data validation. However, ignoring them — whether by accident or design — can lead to unvalidated input and potential errors.
Solution
Be sure to incorporate validation annotations on your form backing object. Here is an example:
public class User {
@NotNull(message = "Username cannot be empty")
@Size(min = 3, max = 15, message = "Username must be between 3 to 15 characters")
private String username;
// Getters and Setters
}
Using these annotations immediately informs your application of business rules regarding data entry.
3. Not Handling Validation Errors Properly
Another common mistake developers can make is not properly handling validation errors in the view. If validation fails and you don't show appropriate error messages, users may be left confused as to why their input was rejected.
Solution
In your controller, make sure to add the BindingResult
parameter to check for errors. For instance:
@PostMapping("/register")
public String registerUser(@Valid @ModelAttribute("user") User user, BindingResult result) {
if (result.hasErrors()) {
return "registration";
}
// Save user and redirect
return "redirect:/success";
}
In the Thymeleaf template, display errors using the following snippet:
<div th:if="${#fields.hasErrors('username')}" th:errors="*{username}">
</div>
4. Misconfiguring Validator Beans
When using custom validation, misconfiguration of Validator beans could lead to unexpected behavior. If your custom validator is not registered or annotated appropriately, it will not work with the @Valid
or @Validated
annotations.
Solution
Ensure that your validator is correctly registered as a Spring bean. For example:
@Component
public class CustomUserValidator implements Validator {
@Override
public boolean supports(Class<?> clazz) {
return User.class.equals(clazz);
}
@Override
public void validate(Object target, Errors errors) {
User user = (User) target;
// Custom validation logic
}
}
Don’t forget to register it in your controller:
@Autowired
private CustomUserValidator customUserValidator;
@PostMapping("/register")
public String registerUser(@Valid @ModelAttribute("user") User user,
BindingResult bindingResult) {
customUserValidator.validate(user, bindingResult);
if (bindingResult.hasErrors()) {
return "registration";
}
// Save user and redirect
return "redirect:/success";
}
5. Lack of Client-Side Validation
While server-side validation is essential, relying solely on it can lead to a poor user experience. Client-side validation using JavaScript gives instant feedback to users before the form even reaches the server.
Solution
Integrate client-side validation libraries like jQuery Validate. Here's an example:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://cdn.jsdelivr.net/jquery.validation/1.19.3/jquery.validate.min.js"></script>
<script>
$(document).ready(function() {
$("form").validate({
rules: {
username: {
required: true,
minlength: 3,
maxlength: 15
}
},
messages: {
username: {
required: "Please enter a username",
minlength: "Username must be at least 3 characters long"
}
}
});
});
</script>
6. Failing to Localize Validation Messages
Users appreciate when validation messages are presented in their native languages. Ignoring localization can lead to confusion, especially in multicultural applications.
Solution
Use messages.properties files in your Spring application to provide localized messages:
# messages.properties
username.empty=Username cannot be empty
username.size=Username must be between 3 to 15 characters
You would then access them in your validation annotations:
@NotNull(message = "{username.empty}")
@Size(min = 3, max = 15, message = "{username.size}")
private String username;
The Bottom Line
Understanding the common mistakes associated with Thymeleaf validation in Spring applications is crucial for any developer seeking to implement secure and effective data validation. By addressing issues such as binding errors, misconfigured validators, and validation message localization, you can significantly improve user experience and application reliability.
To deepen your understanding, consider reviewing Spring's validation documentation. With these best practices in mind, you can confidently build robust and user-friendly applications.
Now, go forth and code with confidence!