Optimize Your WAR Files: Manage Dependencies Efficiently
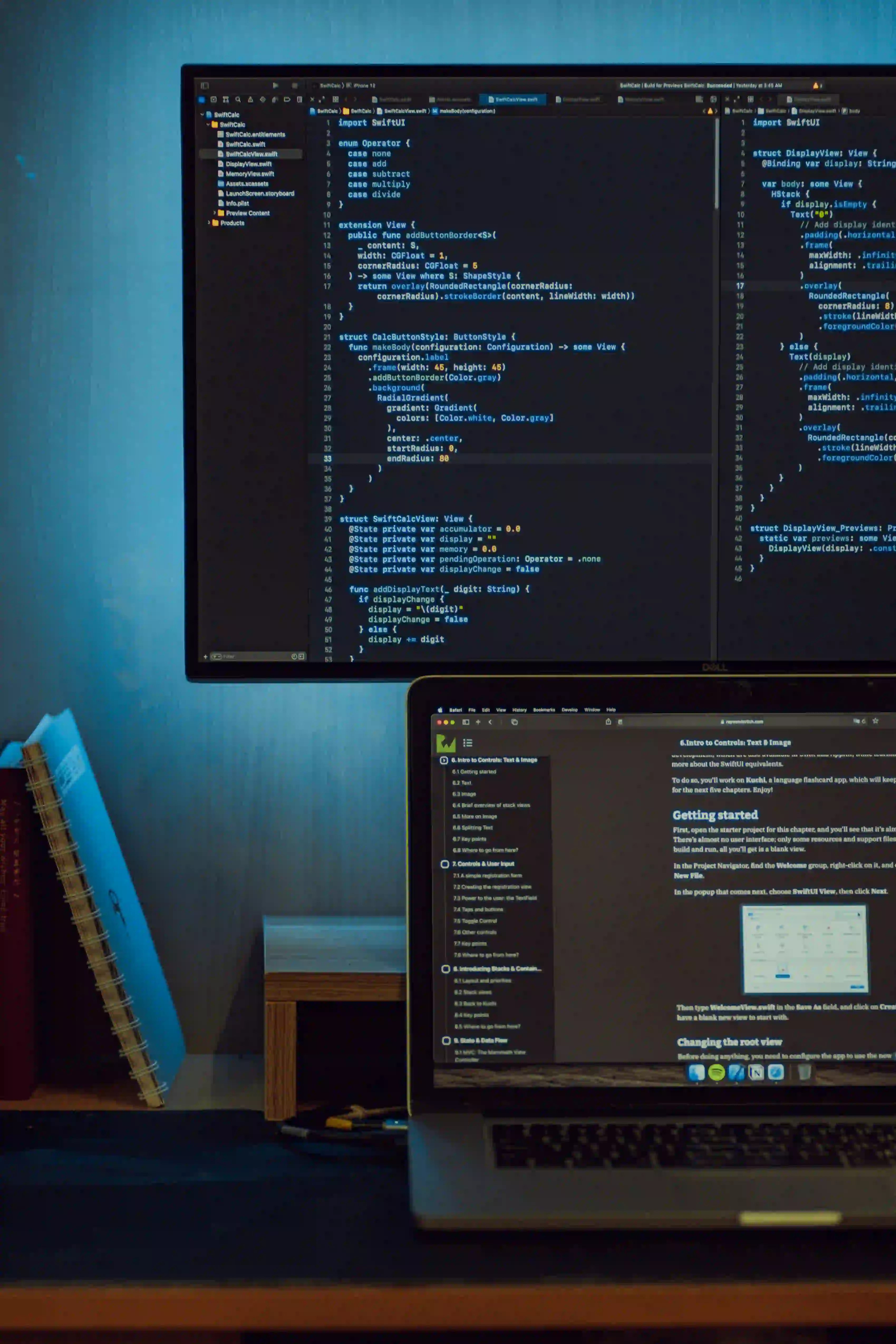
Optimize Your WAR Files: Manage Dependencies Efficiently
When developing Java web applications, deploying your application as a Web Application Archive (WAR) file is a common practice. However, the way you manage dependencies can significantly affect the size and performance of your WAR files. In this blog post, we’ll explore how to efficiently manage dependencies, providing clear code snippets that illustrate the concepts.
Understanding WAR Files
A WAR file is essentially a compressed archive that houses all the files needed for a web application, including Java classes, libraries, JSPs, HTML, CSS, and JavaScript files. By default, a WAR file can accumulate a lot of redundant libraries, leading to bloated applications that take longer to load and consume more memory.
Why Optimize WAR Files?
- Faster Deployment: Smaller WAR files deploy more quickly to the server.
- Reduced Memory Usage: Less overhead translates to better memory management.
- Improved Load Times: Efficient applications boost user experience through quicker load times.
- Easier Maintenance: A streamlined application simplifies updates and debugging.
To achieve these benefits, efficient dependency management becomes paramount.
Effective Dependency Management Strategies
1. Use Dependency Management Tools
Using tools like Maven or Gradle simplifies the complexity of managing dependencies. These tools can help identify and resolve transitive dependencies—dependencies that your dependencies rely on.
Maven Example
The following snippet shows a basic Maven pom.xml
file with dependency management:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-webapp</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>war</packaging>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.3.8</version>
</dependency>
<!-- Avoid unnecessary libraries -->
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-simple</artifactId>
<version>1.7.32</version>
<scope>runtime</scope>
</dependency>
</dependencies>
</project>
The key point here is the scope
attribute. Setting a dependency to runtime
ensures it's included in the compiled library but not during compile time, reducing the bloat in your WAR file.
2. Exclude Unnecessary Transitive Dependencies
Often, libraries bring in additional dependencies that your application may not need. It's essential to exclude these unnecessary libraries using the <exclusions>
tag in Maven.
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>30.1-jre</version>
<exclusions>
<exclusion>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</exclusion>
</exclusions>
</dependency>
In this example, we exclude the httpclient
dependency because it's not required in our project. This practice keeps our WAR file size minimized.
3. Leverage the “provided” Scope
When deploying to a server that already includes certain libraries (e.g., a servlet API), mark these dependencies with the provided
scope in Maven.
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
<scope>provided</scope>
</dependency>
This prevents the servlet API from being packaged inside the WAR, effectively reducing the file size.
4. Use the Maven Assembly Plugin
For final packaging, use the Maven Assembly Plugin to create a clean, slim WAR file without unnecessary files.
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-assembly-plugin</artifactId>
<version>3.3.0</version>
<configuration>
<descriptorRefs>
<descriptorRef>war</descriptorRef>
</descriptorRefs>
<finalName>${project.artifactId}-${project.version}</finalName>
</configuration>
<executions>
<execution>
<id>make-assembly</id>
<phase>package</phase>
<goals>
<goal>single</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
This snippet configures Maven to create a slim WAR file during the package phase by utilizing a predefined distribution layout.
5. Monitor and Analyze Dependencies
Finally, monitoring and analyzing your dependencies is vital. Tools like Maven Dependency Plugin can help identify the size and scope of each dependency in your project.
mvn dependency:tree
The command above returns a tree view of your dependencies, allowing you to assess what's necessary and what can be removed or excluded.
In Conclusion, Here is What Matters
Effective dependency management is crucial for optimizing your WAR files. By employing tools like Maven or Gradle, excluding unnecessary dependencies, leveraging scopes appropriately, and using assembly plugins, you can create streamlined, efficient web applications.
Next Steps
- Conduct a detailed analysis of your current dependencies.
- Start implementing the strategies discussed above.
- Monitor the WAR file size after adjustments to evaluate the impact.
By following these practices, you will not only enhance the performance of your Java web application but also ensure a smoother and more efficient deployment and maintenance cycle. As you improve your dependency management skills, the quality of your applications will surely shine through.
For more insights on Java development and optimization, check out Baeldung and Java Code Geeks. Happy coding!
This blog post contains actionable insights and code snippets tailored for Java developers. Optimize your WAR files today and experience the benefits immediately.