Common Pitfalls When Using CGLIB to Create Proxy Objects
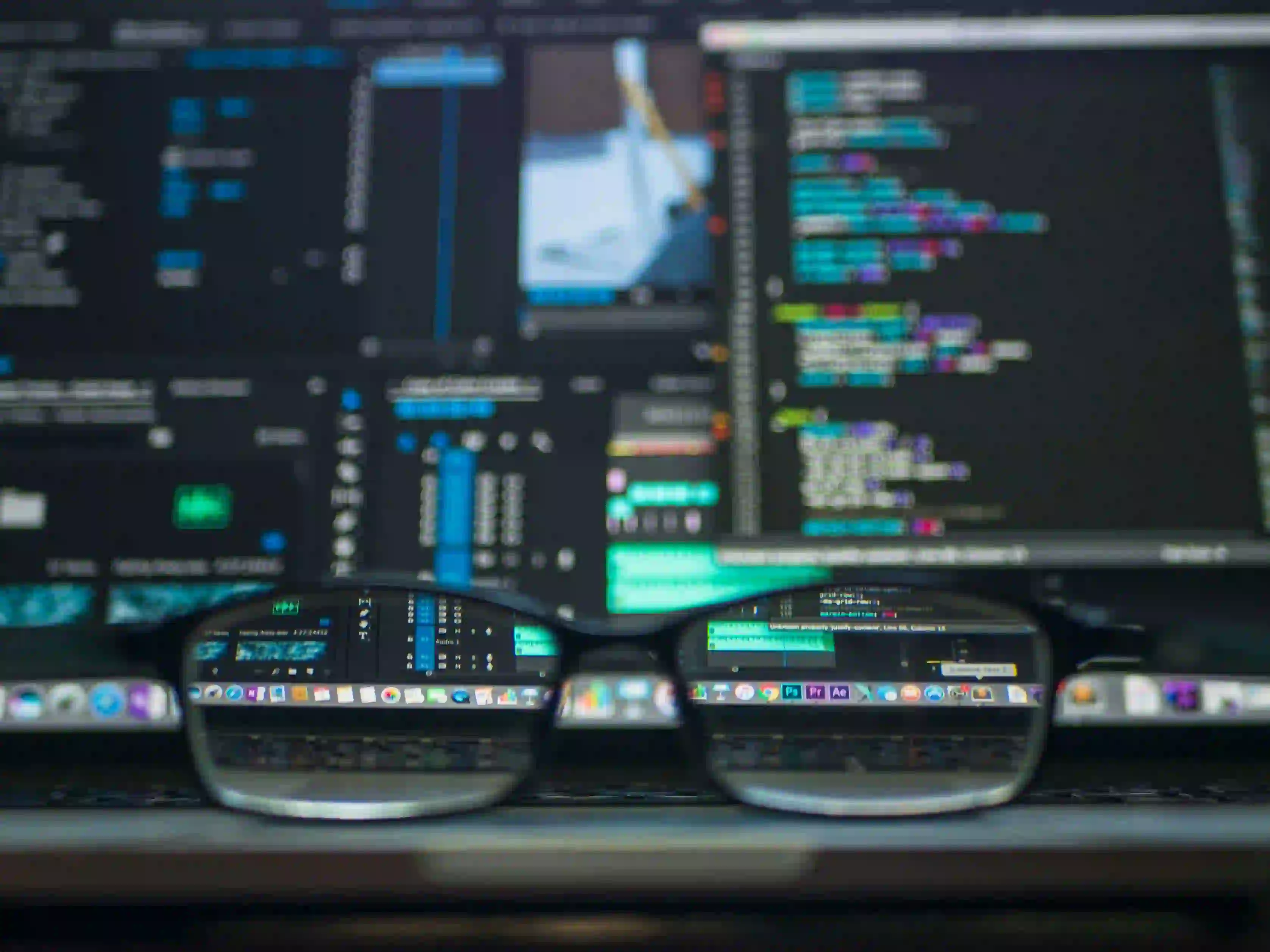
Common Pitfalls When Using CGLIB to Create Proxy Objects
CGLIB (Code Generation Library) is a powerful tool in the Java ecosystem, mainly used for creating dynamic proxy objects. Unlike Java's inherent proxy mechanism, CGLIB creates proxies by subclassing the target class. This opens up numerous possibilities, particularly in frameworks like Spring, but it also comes with its share of pitfalls.
In this post, we will explore common pitfalls when using CGLIB to create proxy objects, how to avoid them, and best practices to ensure smooth development.
What is CGLIB?
CGLIB is a code generation library that extends Java classes and implements interfaces at runtime. It is especially valuable for creating proxies of classes that do not implement interfaces. For an in-depth understanding of CGLIB, you can check the official CGLIB documentation.
How Does CGLIB Work?
CGLIB operates by extending classes and overriding methods in order to add behavior. This allows you to intersperse cross-cutting concerns, such as transaction handling or logging, with minimal boilerplate code.
Below is a simple example showing how to create a proxy using CGLIB:
import net.sf.cglib.proxy.Enhancer;
import net.sf.cglib.proxy.MethodInterceptor;
import net.sf.cglib.proxy.MethodProxy;
class UserService {
public void performAction() {
System.out.println("Action performed");
}
}
class UserServiceInterceptor implements MethodInterceptor {
@Override
public Object intercept(Object obj, java.lang.reflect.Method method, Object[] args, MethodProxy proxy) throws Throwable {
System.out.println("Before method " + method.getName());
Object result = proxy.invokeSuper(obj, args); // Invoking the actual method
System.out.println("After method " + method.getName());
return result;
}
}
public class CGLIBExample {
public static void main(String[] args) {
Enhancer enhancer = new Enhancer();
enhancer.setSuperclass(UserService.class);
enhancer.setCallback(new UserServiceInterceptor());
UserService proxy = (UserService) enhancer.create();
proxy.performAction(); // Proxy method
}
}
In this code snippet, UserService
is a simple class we want to enhance. The UserServiceInterceptor
implements the MethodInterceptor
interface, providing hooks before and after method invocations.
Common Pitfalls of Using CGLIB
1. Final Classes and Methods
Problem: CGLIB cannot create proxies for final classes or methods. If you try to enhance a final class, it will throw a Throwable
.
Solution: To avoid this, ensure that the classes and methods you want to proxy are not marked as final. Use designs that favor abstraction over concrete implementations, enabling flexibility.
// This class cannot be proxied with CGLIB
final class FinalService {
public void execute() {
System.out.println("Executing...");
}
}
// Change to a non-final class
class BaseService {
public void execute() {
System.out.println("Executing...");
}
}
2. Constructor Overloading
Problem: CGLIB generates a subclass at runtime, and if your class includes overloaded constructors, it will only invoke the default constructor. Thus, any initialization performed in the other constructors will be missed.
Solution: Stick to using the default constructor for classes that you plan to proxy with CGLIB. If specific initialization is necessary, consider using another method explicitly.
class User {
private String name;
// Overloaded constructors will not be called
public User() {
this.name = "Default User";
}
public User(String name) {
this.name = name;
}
}
3. Static Methods
Problem: CGLIB cannot proxy static methods since they belong to the class rather than an instance.
Solution: If you need to intercept method calls, consider using either instance methods or refactor the static methods into instance methods, or use Java's built-in proxy mechanism, which can work for interfaces.
class StaticUser {
public static void staticMethod() {
System.out.println("Static method");
}
}
// Cannot proxy the static method
4. Implicit Dependency on ClassLoader
Problem: Instances of CGLIB proxies are dependent on the ClassLoader of the target class. Different class loaders may lead to ClassCastException
.
Solution: Be cautious about class loading, especially in complex environments such as application servers. Use a consistent class loader across your modules.
5. Performance Overhead
Problem: Creating proxies dynamically incurs some performance overhead. If excessively used, this can degrade application performance.
Solution: Use CGLIB proxies judiciously. Measure performance impacts using profiling tools, and consider caching the proxies where applicable.
6. Thread Safety
Problem: CGLIB proxies do not handle thread safety automatically. If your proxied class modifies shared state, you may encounter concurrency issues.
Solution: Ensure that your instantiation and access patterns abate concurrency issues. Use synchronized blocks or concurrent collections to manage shared resources.
Wrapping Up
CGLIB is a versatile tool perfect for enhancing object behavior through proxies. However, it also comes with inherent pitfalls that can introduce bugs, performance issues, or instability if not handled carefully.
By understanding these common pitfalls—such as limitations with final classes, constructor overloading, static methods, thread safety, and performance overhead—you can effectively leverage CGLIB while avoiding potential disasters in your Java projects.
For further exploration of proxy mechanics, consider looking into the Spring documentation on AOP (Aspect Oriented Programming).
Further Reading
By using these insights and considerations, you can take full advantage of what CGLIB offers in your development toolkit while ensuring a robust and maintainable codebase. Happy coding!