Common Pitfalls in JBoss Drools: Avoiding Rule Conflicts
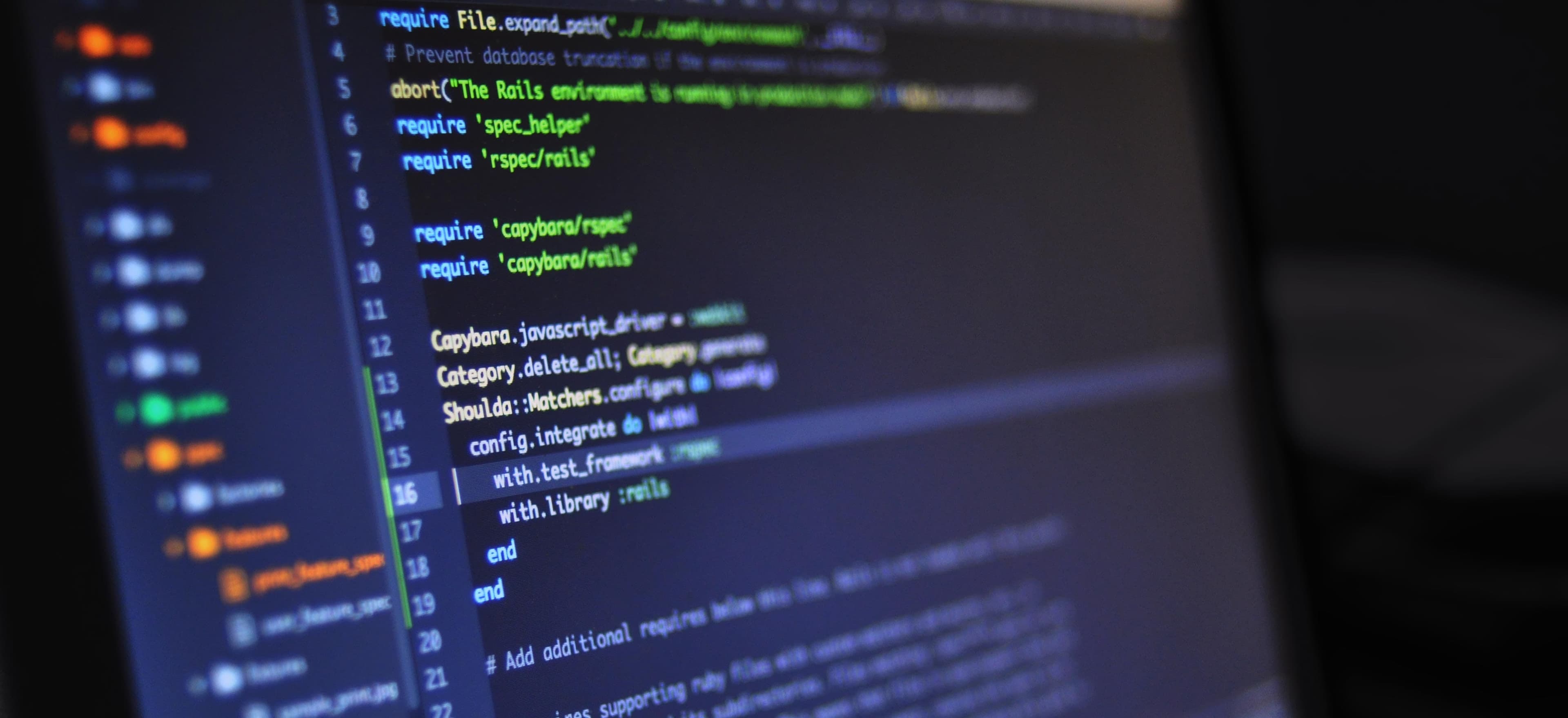
- Published on
Common Pitfalls in JBoss Drools: Avoiding Rule Conflicts
Drools, part of the JBoss family, is a powerful Rule Engine used for business rule management. While it is an excellent tool for automating decisions, developers must navigate several pitfalls to maximize its effectiveness. One of the most pressing issues developers face is rule conflicts. In this blog post, we will explore what rule conflicts are, how they arise, and steps you can take to avoid them. We will also provide exemplary code snippets along with strategic insights that will help you understand best practices while using JBoss Drools.
What Are Rule Conflicts?
Rule conflicts arise when two or more rules attempt to modify the same object or produce conflicting results for the same condition. This situation can lead to unpredictable behavior in your business logic. Typically, conflicts manifest in these ways:
- Duplicate Conditions: Two rules evaluate the same conditions and lead to different outcomes.
- Aggressive Rule Prioritization: Rules that are given higher priorities may override those that deserve precedence based on business logic.
- Lack of Clear Activation Groups: When several rules need to be activated or inactivated together, failure to use activation groups can lead to chaos.
To minimize these conflicts, it is essential to have a grasp of the kinds of situations that can lead to rule conflicts and understand how Drools resolves these issues.
Understanding the Basics of Rule Declaration
Before we dive deeper, let’s establish a basic structure of a Drools rule. Here’s a simple example:
rule "Calculate Discount"
when
Customer(eligibleForDiscount == true)
then
// Logic to apply discount
System.out.println("Discount applied to customer");
end
This rule checks if a customer is eligible for a discount and processes it accordingly. However, if you were to create another rule that modifies the customer's discount simultaneously, you might encounter a conflict.
Identifying Rule Conflicts
Many conflicts may arise unnoticed. Here are some techniques to identify these issues efficiently:
-
Rule Prioritization: Ensure that rules have been assigned the correct priority. Use the
salience
keyword to manage which rule should fire first.rule "High Priority Discount" salience 10 when Customer(eligibleForHighDiscount == true) then // Logic System.out.println("High discount applied."); end
-
Understand Rule Flow: Utilize the Drools rule flow diagram feature. This diagram visually represents how rules interact, helping identify potential conflicts before runtime.
-
Testing Rules: Collaboratively develop unit tests that cover different scenarios, ensuring that rule conditions do not cause unintended behavior.
Avoiding Common Rule Pitfalls
The following are strategies to help developers avoid conflicts in Drools.
1. Use Activation Groups
Activation groups group rules that are related to the same condition. When one rule in an activation group is activated, the others are deactivated. This is particularly beneficial for avoiding direct conflicts. Here’s an example:
rule "Discount for Loyal Customers"
activation-group "discount-rule-group"
when
Customer(loyaltyPoints > 100)
then
System.out.println("Loyal customer discount applied.");
end
rule "Discount for New Customers"
activation-group "discount-rule-group"
when
Customer(tenure < 1)
then
System.out.println("New customer discount applied.");
end
In this case, only one rule from the group can be activated, thereby reducing the risk of conflicting discounts being applied.
2. Refactor Code for Clarity
Sometimes conflicts arise from complex or poorly structured rules. Aim to break down large rules into smaller, more specific rules. This practice not only prevents errors but also enhances readability, making it easier for team members to understand the intent of each rule.
3. Ensure Consistent Fact Updates
Drools operates on facts, which are the foundational objects on which rules operate. Always ensure that facts are updated consistently before rules are fired. Utilize the following code structure for clarity:
rule "Update Customer"
when
Customer(eligibleForDiscount == true)
then
customer.setDiscountApplied(true);
update(customer);
end
By using the update()
command, you ensure that other rules will have the most current state of the facts when they evaluate.
4. Implement Testing Practices
Utilize JUnit for testing your Drools rules. This practice helps ensure that rules work as expected and handle conflicts where they're managed. Here’s a basic unit test framework to consider:
import org.kie.api.runtime.KieSession;
import org.kie.api.KieServices;
import org.kie.api.runtime.KieContainer;
public class RuleTest {
@Test
public void testCustomerDiscountEligibility() {
KieServices ks = KieServices.Factory.get();
KieContainer kc = ks.getKieClasspathContainer();
KieSession kSession = kc.newKieSession("ksession-rules");
Customer customer = new Customer();
customer.setEligibleForDiscount(true);
kSession.insert(customer);
kSession.fireAllRules();
assertTrue(customer.isDiscountApplied());
}
}
This unit test will verify that the discount is applied under the expected conditions.
Key Takeaways
Drools is an incredibly useful tool for implementing business rules but comes with its own set of challenges, particularly regarding rule conflicts. By being aware of potential pitfalls and employing strategies like using activation groups, refactoring for clarity, ensuring consistent fact updates, and implementing solid testing practices, developers can significantly mitigate the risk of rule conflicts.
For more in-depth insights and documentation about managing rules effectively in JBoss Drools, you can check out the Drools Documentation and the JBoss Community.
By embracing these practices, you can ensure that your Drools implementations will be effective, reliable, and maintainable, allowing you to harness its full potential without the headaches of rule conflicts. Happy coding!
Checkout our other articles