Mastering Exception Logging in Spring Boot: Common Pitfalls
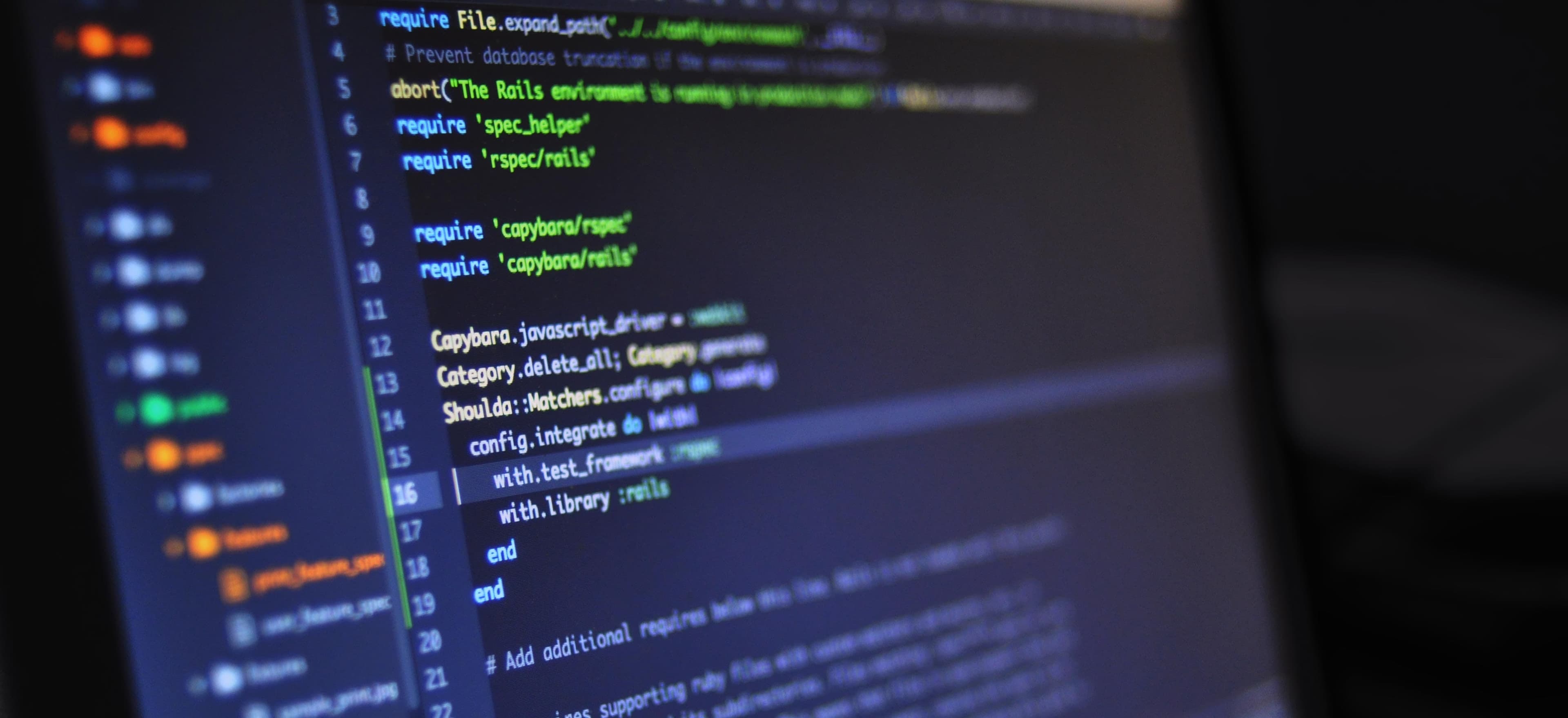
- Published on
Mastering Exception Logging in Spring Boot: Common Pitfalls
Exception logging is a vital part of any application. In Spring Boot, this is no different. Whether you are building a RESTful web service, a microservice, or any type of application, knowing how to properly log exceptions can help you troubleshoot and maintain your application effectively. In this blog post, we will delve into common pitfalls in exception logging using Spring Boot and how to master them for robust application performance.
The Importance of Exception Logging
Logging exceptions aids in the identification and rectification of issues during development and post-deployment. Proper logs provide insights into:
- Error Diagnosis: Quickly understand what went wrong.
- Performance Monitoring: Analyze the behavior of your application under load.
- Security Auditing: Track anomalies that may indicate vulnerabilities.
To ensure effective logging, it's essential to avoid pitfalls that can obscure error information or overwhelm the log files.
Common Pitfalls in Exception Logging
1. Not Using a Consistent Logging Framework
Using different logging frameworks across various parts of your application can lead to inconsistencies. Spring Boot supports popular logging frameworks like Logback, Log4j2, and SLF4J by default.
To ensure a consistent logging approach, choose one logging framework to use across your application. Here’s how you can configure Logback in your Spring Boot application:
<!-- In your pom.xml -->
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
</dependency>
2. Insufficient Logging Levels
Many developers get overwhelmed with logging levels such as DEBUG, INFO, WARN, ERROR, etc. Choosing the wrong logging level can lead to critical information being missed or irrelevant details cluttering the logs.
Be intentional about your log levels:
- DEBUG: Use for detailed troubleshooting during development.
- INFO: For actionable messages that show the progress of your application.
- WARN: For potentially harmful situations.
- ERROR: For when an error prevents the application from performing a specific task.
Here’s an example:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyService {
private static final Logger logger = LoggerFactory.getLogger(MyService.class);
public void process() {
logger.info("Starting process");
try {
// Some processing logic
} catch (MySpecificException e) {
logger.error("Error occurred while processing: {}", e.getMessage());
}
}
}
3. Not Logging Enough Context
When exceptions occur, it’s essential to log contextual information, such as:
- User ID
- Session ID
- Request Parameters
Failing to do so can make diagnosing issues nearly impossible. For example:
public void handleRequest(Request request) {
logger.info("Handling request for user ID: {}", request.getUserId());
try {
// processing logic
} catch (Exception e) {
logger.error("Exception occurred for user ID: {}. Error: {}", request.getUserId(), e.getMessage());
}
}
4. Ignoring Exception Stack Traces
When logging exceptions, it’s important to log the full stack trace in order to analyze what led to the error. Logging only the message without the stack trace can lead to loss of critical debugging information.
You can log exceptions in Spring Boot like this:
try {
// Some logic that may throw an exception
} catch (Exception e) {
logger.error("An error occurred: ", e);
}
This will capture the complete stack trace in the logs, providing thorough context for future debugging.
5. Over-Logging
While logging is critical, it’s easy to fall into the trap of logging too many details. Producing excessive logs can cause performance bottlenecks and make analysis cumbersome.
Check logs periodically to ensure they remain relevant. Focus on what’s essential and omit duplicative or trivial logs. You can maintain the quality of logs by setting appropriate filtering in your logging configuration.
This snippet illustrates filtering to keep logs manageable:
# In application.yml
logging:
level:
root: WARN
com.example: DEBUG
6. Failing to Handle Asynchronous Logging
In a Spring Boot application, asynchronous processing can lead to lost logs. To mitigate this, consider configuring your logging to capture asynchronous log messages reliably. This can be accomplished via specialized logging appenders.
Example configuration can help:
<appender name="ASYNC" class="ch.qos.logback.classic.AsyncAppender">
<appender-ref ref="CONSOLE"/>
</appender>
7. Neglecting External Logger Management Tools
Every Spring Boot application benefits from centralized logging solutions like ELK Stack (Elasticsearch, Logstash, Kibana) or Grafana Loki. Relying solely on local logs can lead to missed insights.
Consider integrating tools like:
These tools enable you to visualize logs and analyze trends over time.
Key Takeaways
Mastering exception logging in Spring Boot is instrumental for robust application performance. By avoiding common pitfalls such as inconsistent logging frameworks, insufficient logging levels, and neglecting context, you can significantly enhance your error-tracking capabilities.
Adopt best practices to ensure that your logging serves as an asset rather than a burden. With consistent application and the right tools, you will transform your Spring Boot application into a well-oiled machine that can efficiently tackle exceptions without losing sight of the bigger picture.
For further reading on logging in Spring Boot, check out the Spring Boot Reference Documentation.
Feel free to share your thoughts or questions in the comments section below!
Checkout our other articles