Understanding the Pitfalls of Pseudorandomness in Cryptography
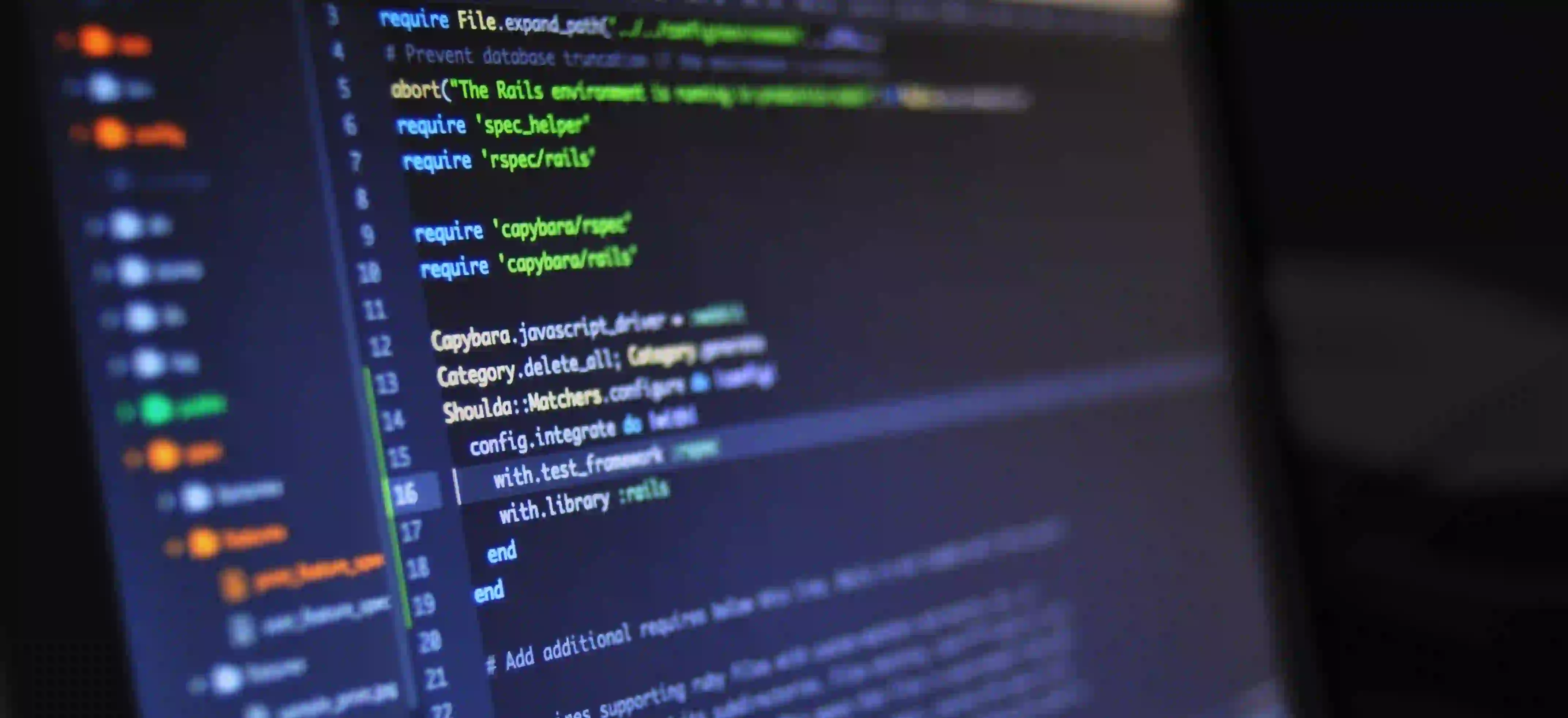
Understanding the Pitfalls of Pseudorandomness in Cryptography
In the world of cryptography, randomness plays a pivotal role. From key generation to secure protocol design, randomness is foundational. However, not all randomness is created equal, and this brings us to the concept of pseudorandomness. In this article, we will explore what pseudorandomness is, the potential pitfalls associated with its use, and how to mitigate these issues through best practices.
What is Pseudorandomness?
Pseudorandomness refers to sequences of numbers that appear random but are generated by deterministic processes. In cryptography, pseudorandom number generators (PRNGs) are algorithms that produce a sequence of numbers that pass various statistical tests for randomness. However, since these algorithms are deterministic, the sequences they produce can be predicted if the algorithm and its internal state are known.
Why Pseudorandomness Matters
Pseudorandomness is crucial in cryptography because it helps create:
- Keys: Secure cryptographic keys must be unpredictable.
- Nonces: Unique numbers used only once can protect against replay attacks.
- Initialization Vectors: These ensure the same plaintext encrypted multiple times produces different ciphertexts.
Therefore, understanding the implications of pseudorandomness is essential for effective cryptographic design.
Common Pitfalls of Pseudorandomness
1. Predictability
One of the most significant concerns when it comes to pseudorandomness is predictability. If an attacker can determine the state of the PRNG, they can predict future outputs, compromising the security of the system.
Example:
Let's look at a basic example using a simple PRNG in Java.
import java.util.Random;
public class PredictablePRNG {
public static void main(String[] args) {
Random rand = new Random(12345); // Seeded with a fixed value
for (int i = 0; i < 10; i++) {
System.out.println(rand.nextInt(100));
}
}
}
Why This is Problematic: In this example, the Random object is seeded with a fixed value (12345). Consequently, every time this program is run, it produces the same sequence of numbers. An attacker who understands this can easily predict the output.
2. Insufficient Entropy
Entropy is a measure of randomness. A PRNG that lacks sufficient entropy will yield predictable outputs. In many systems, highlighted bits of entropy come from unpredictable external sources, such as mouse movements or system events. If these sources are weak or insufficient, the overall randomness diminishes.
import java.security.SecureRandom;
public class SecurePRNG {
public static void main(String[] args) {
SecureRandom secureRandom = new SecureRandom();
for (int i = 0; i < 10; i++) {
System.out.println(secureRandom.nextInt(100));
}
}
}
Why This is Better: The SecureRandom
class in Java draws from a more unpredictable source, making the generated numbers much harder to predict compared to a standard Random
class. Always prefer cryptographic secure sources for randomness in secret and sensitive applications.
3. Seeding Issues
The way a PRNG is seeded can greatly affect its security. For instance, using predictable values for seeding lowers the quality of randomness. This could stem from user input or system time, which can often be anticipated.
public class RandomSeeding {
public static void main(String[] args) {
long currentTime = System.currentTimeMillis(); // Time-based seed
Random rand = new Random(currentTime);
for (int i = 0; i < 10; i++) {
System.out.println(rand.nextInt(100));
}
}
}
Why This is Insecure: Using the current system time as a seed can be easily predicted. An attacker can match the system time with the generated random output.
How to Mitigate Pseudorandomness Risks
Employ Secure PRNGs
Whenever randomness is required in cryptographic contexts, leverage a cryptographically secure PRNG. In Java, the SecureRandom
class is designed specifically for this purpose.
Incorporate Cryptographic Best Practices
- Avoid Fixed Seeds: Always use unpredictable and high-entropy sources for seeding. If possible, use
SecureRandom
to handle the seeding automatically. - Regularly Reseed the PRNG: Implement periodic reseeding mechanisms to ensure the internal state remains unpredictable.
import java.security.SecureRandom;
public class ReseedExample {
private SecureRandom secureRandom;
public ReseedExample() {
this.secureRandom = new SecureRandom();
this.secureRandom.setSeed(System.nanoTime()); // Better seeding
}
public int getRandomNumber() {
return secureRandom.nextInt(100);
}
// Call this method to reseed at strategic times
public void reseed() {
byte[] seed = new byte[16];
secureRandom.nextBytes(seed);
secureRandom.setSeed(seed);
}
}
Conduct Regular Security Audits
Finally, performing regular audits of your randomness generation strategy and its implementation can help identify weaknesses and ensure compliance with the latest cryptographic standards.
The Bottom Line
Pseudorandomness is a double-edged sword in cryptography. While it is an essential tool, misusing it can lead to dire security vulnerabilities. By understanding the pitfalls of pseudorandomness and adhering to best practices in PRNG implementation, you can safeguard your applications against predictable attacks and reinforce your security posture.
For further reading on secure key management, you might find NIST SP 800-90A insightful. Ensuring that your cryptographic systems are secure is an ongoing process—stay educated, stay secure.
References:
- Java SecureRandom Documentation
- Random Number Generation in Cryptography
- NIST SP 800 Series Publications
In the realm of cryptography, staying informed is your best defense against emerging vulnerabilities. Embrace constant learning!