Top 5 Spring MVC Mistakes That Beginners Make
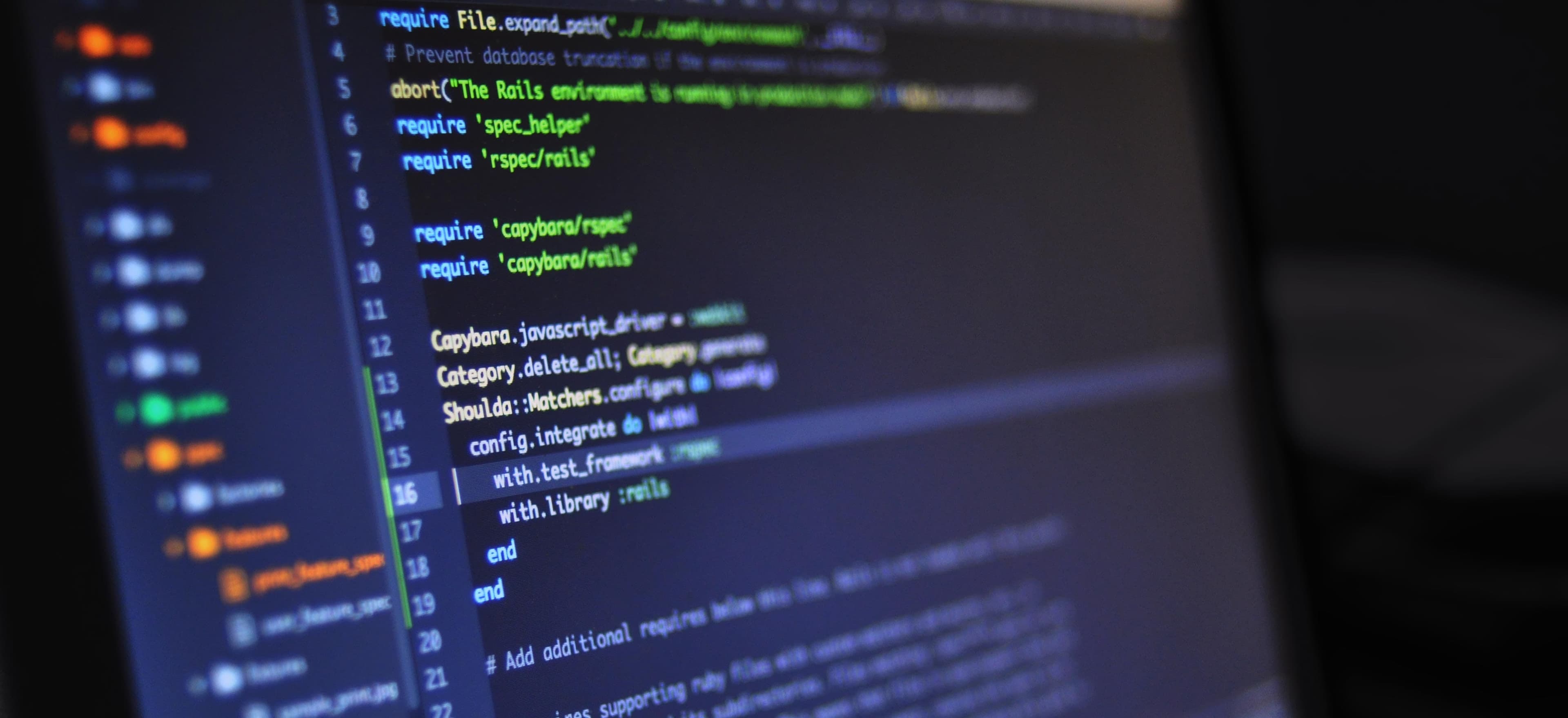
- Published on
Top 5 Spring MVC Mistakes That Beginners Make
Spring MVC is a robust framework that simplifies the development of Java web applications. While it offers powerful features and ease of use, beginners often fall into several common pitfalls that can thwart their projects. This blog post highlights the top five mistakes that beginners make with Spring MVC, providing insights into how to avoid them effectively.
1. Ignoring the DispatcherServlet Configuration
One of the first mistakes that beginners make is neglecting the proper configuration of the DispatcherServlet. The DispatcherServlet is the heart of the Spring MVC framework, responsible for routing requests to the appropriate controllers.
Why This Matters
A poorly configured DispatcherServlet can lead to issues like HTTP 404 errors, where the application cannot find the requested resource.
Example
Here's how you might configure the DispatcherServlet in your web.xml:
<servlet>
<servlet-name>dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dispatcher</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
Commentary
In this setup, we declare the DispatcherServlet and map it to the root URL pattern. This configuration tells Spring to handle all incoming requests. Failing to set this up correctly will result in unhandled requests, disrupting the user experience.
For more in-depth information on configuring the DispatcherServlet, check out the Spring MVC documentation.
2. Not Leveraging Annotations
Many beginners stick to XML configuration, even in projects where annotations are available. This can make your code cluttered and harder to maintain.
Why This Matters
Annotations provide a more concise and manageable way to configure your application. Using them leads to cleaner code and faster development.
Example
Instead of configuring controllers in XML, you can use annotations like @Controller
and @RequestMapping
:
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class HelloController {
@RequestMapping("/hello")
@ResponseBody
public String sayHello() {
return "Hello, World!";
}
}
Commentary
In this example, the @Controller
annotation indicates that this class serves as a controller for handling web requests. The @RequestMapping
annotation maps the /hello
URL to the sayHello
method. This reduces boilerplate code and improves readability.
3. Misunderstanding the Model-View-Controller Pattern
A fundamental mistake is misinterpreting how the Model-View-Controller (MVC) pattern works in Spring. Beginners often confuse the roles of these components.
Why This Matters
Understanding the distinctions between Model, View, and Controller is crucial for proper application architecture and development.
Explanation
-
Model: Represents the data layer. It is where you typically integrate with your database (e.g., using Spring Data JPA).
-
View: The user interface that presents data to the user. Use technologies like JSP, Thymeleaf, or any other view technology to design your views.
-
Controller: Acts as a bridge between Model and View. It processes user inputs and returns the appropriate response.
Example Scenario
Think of a simple scenario where we render a user profile. Here is how to separate concerns:
Controller
@Controller
public class UserController {
private final UserService userService;
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/user/{id}")
public String getUserProfile(@PathVariable Long id, Model model) {
User user = userService.getUserById(id);
model.addAttribute("user", user);
return "userProfile";
}
}
View (userProfile.html using Thymeleaf)
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>User Profile</title>
</head>
<body>
<h1 th:text="${user.name}">User Name</h1>
<p th:text="${user.email}">User Email</p>
</body>
</html>
Commentary
Each component serves its purpose in the MVC architecture. The controller fetches user data from the service and adds it to the model. The view then presents that model data to the user. By adhering to the MVC pattern, we ensure better separation of concerns and code maintainability.
4. Poor Exception Handling
Another common mistake that novices face is handling exceptions ineffectively. Beginners often overlook this critical aspect, leading to error-prone applications.
Why This Matters
Proper exception handling improves user experiences by providing meaningful messages instead of generic errors, such as HTTP 500 errors.
Example
Here’s how to implement global exception handling using @ControllerAdvice
:
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseStatus;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(UserNotFoundException.class)
@ResponseStatus(HttpStatus.NOT_FOUND)
public String handleUserNotFound(UserNotFoundException e) {
return "error/user-not-found";
}
}
Commentary
In this example, the GlobalExceptionHandler
class intercepts UserNotFoundException
and returns a custom error page. This approach centralizes error handling, allowing for easier maintenance and a consistent user experience.
5. Neglecting to Utilize Spring Boot Features
With the introduction of Spring Boot, developers have access to numerous features that make Spring MVC development straightforward. However, beginners often ignore these powerful tools.
Why This Matters
Spring Boot automates many configurations that can save you time and help avoid common pitfalls.
Example
Using Spring Boot, you can significantly reduce boilerplate code by relying on the auto-configuration feature. Here's how a simple application might look:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
Commentary
In this setup, the @SpringBootApplication
annotation encompasses three annotations: @Configuration
, @EnableAutoConfiguration
, and @ComponentScan
. This simplifies application setup, letting you focus on building features rather than worrying about tedious configurations.
The Closing Argument
The road to mastering Spring MVC is riddled with potential missteps. By understanding and avoiding these common mistakes – inadequate configuration of the DispatcherServlet, misunderstanding MVC principles, ineffective exception handling, and ignoring Spring Boot – you can create more robust and maintainable applications.
Understanding the intricacies of Spring MVC not only enhances your development capabilities but also enriches your application's overall user experience. Kindly explore the Spring MVC Documentation for further insights, and stay updated on best practices in Java development.
By correcting these common pitfalls early in your development journey, you can compose a solid foundation for your Java web applications, setting yourself on a path toward greater success and satisfaction. Happy coding!