Mastering Java Collections: Common Interview Pitfalls
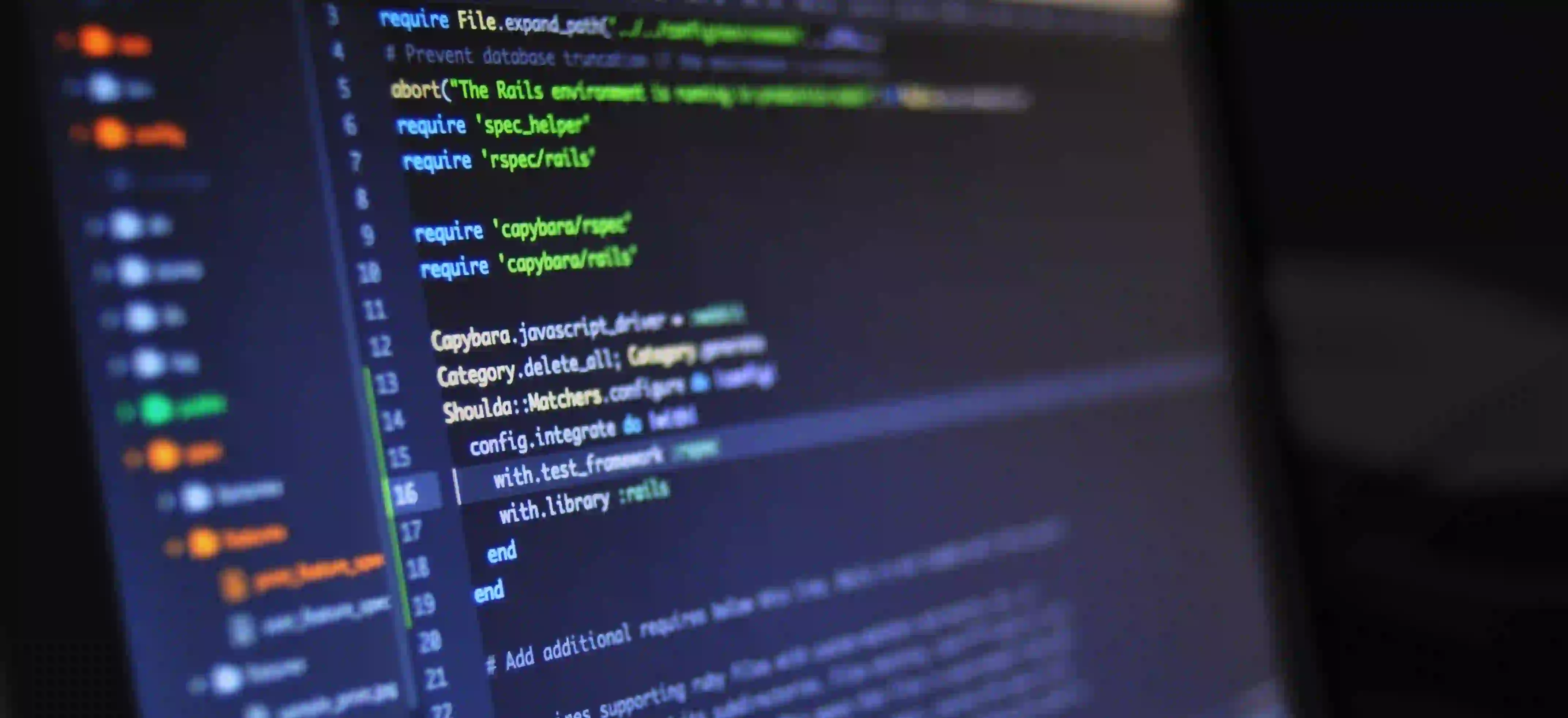
Mastering Java Collections: Common Interview Pitfalls
Java Collections are vital for any software developer working with Java. They enable developers to handle groups of objects effectively, offering powerful tools for data manipulation. However, while they can significantly simplify coding tasks, candidates often stumble over common interview questions and practical tests related to collections. This post aims to empower you to master Java Collections by addressing these pitfalls and providing practical code examples to enhance your understanding.
Understanding Java Collections
The Java Collections Framework (JCF) consists of various classes and interfaces for handling collections of objects. The primary interfaces include:
- List: An ordered collection (also known as a sequence) that may contain duplicates. Implementations include
ArrayList
,LinkedList
, andVector
. - Set: A collection that cannot contain duplicates. Implementations include
HashSet
,LinkedHashSet
, andTreeSet
. - Map: A collection of key-value pairs, with each key being unique. Implementations include
HashMap
,LinkedHashMap
, andTreeMap
.
Understanding these interfaces and their key characteristics is fundamental for coding proficiency and interview success.
Common Pitfalls in Java Collections
1. Confusing Collections with Arrays
Many candidates confuse collections with arrays. Arrays have a fixed size, meaning once they're created, their size cannot change. In contrast, collections can dynamically resize.
Example: Dynamic Sizing in ArrayList
import java.util.ArrayList;
public class DynamicArrayExample {
public static void main(String[] args) {
ArrayList<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
// The list can grow dynamically as we add more elements
names.add("Charlie");
System.out.println("Names: " + names);
}
}
Here, we use ArrayList
, which allows us to add names without worrying about its size. This flexibility is a crucial advantage.
2. Not Understanding the Differences in HashMap and TreeMap
Candidates often fail to recognize when to use HashMap
versus TreeMap
. A HashMap
offers constant-time average performance for key lookups, while a TreeMap
maintains an order based on the natural ordering of its keys or a provided comparator.
Example: Performance Differences
import java.util.HashMap;
import java.util.TreeMap;
public class MapComparisonExample {
public static void main(String[] args) {
HashMap<String, Integer> hashMap = new HashMap<>();
TreeMap<String, Integer> treeMap = new TreeMap<>();
hashMap.put("Tom", 40);
hashMap.put("Jerry", 10);
treeMap.put("Tom", 40);
treeMap.put("Jerry", 10);
System.out.println("HashMap: " + hashMap);
System.out.println("TreeMap (sorted): " + treeMap);
}
}
Choosing HashMap
for speed and TreeMap
for sorted order is essential for optimized performance in your applications.
3. Misunderstanding Null Values in Collections
Java collections handle null values differently. For example, HashSet
and HashMap
allow null elements, while TreeMap
does not allow null keys. Interviewers may evaluate your understanding of these constraints.
Example: Null Handling in HashSet and TreeSet
import java.util.HashSet;
import java.util.TreeSet;
public class NullValueExample {
public static void main(String[] args) {
HashSet<String> hashSet = new HashSet<>();
TreeSet<String> treeSet = new TreeSet<>();
hashSet.add(null); // Allowed
// treeSet.add(null); // Throws NullPointerException
System.out.println("HashSet allows null: " + hashSet);
}
}
Recognizing these nuances can help prevent time-consuming bugs in production.
4. Ignoring Thread-Safety
When dealing with a multi-threaded environment, standard collections are not thread-safe. Candidates often overlook the need for concurrency, leading to potential race conditions in their applications.
Example: Using Concurrent Collections
import java.util.concurrent.ConcurrentHashMap;
public class ThreadSafeMapExample {
public static void main(String[] args) {
ConcurrentHashMap<String, Integer> concurrentMap = new ConcurrentHashMap<>();
// Simulating concurrent modification
concurrentMap.putIfAbsent("key1", 1);
System.out.println("ConcurrentMap: " + concurrentMap);
}
}
Utilizing ConcurrentHashMap
ensures that operations are thread-safe without requiring external synchronization.
5. Inefficient Iteration
Interviews often include questions on iterating through collections. Using an iterator can yield better performance and avoid concurrent modification issues. Yet, many candidates still opt for the traditional for-loop.
Example: Iterating with Iterators
import java.util.ArrayList;
import java.util.Iterator;
public class IteratorExample {
public static void main(String[] args) {
ArrayList<String> colors = new ArrayList<>();
colors.add("Red");
colors.add("Green");
colors.add("Blue");
Iterator<String> iterator = colors.iterator();
while (iterator.hasNext()) {
String color = iterator.next();
System.out.println("Color: " + color);
}
}
}
Using an iterator prevents common pitfalls associated with directly modifying collections during iteration.
6. Underestimating Collections API
Java offers a rich set of methods in the Collections framework, such as sorting, searching, and shuffling. Interviewees often don't exploit these functions.
Example: Sorting a List
import java.util.ArrayList;
import java.util.Collections;
public class SortingExample {
public static void main(String[] args) {
ArrayList<String> fruits = new ArrayList<>();
fruits.add("Banana");
fruits.add("Apple");
fruits.add("Cherry");
Collections.sort(fruits);
System.out.println("Sorted Fruits: " + fruits);
}
}
Understanding and using the Collections API is key to enhancing performance and maintainability.
The Last Word
The Java Collections Framework is indispensable for efficient programming, but mastering it requires avoiding common pitfalls. Familiarizing yourself with the nuances of different collections, understanding when to apply each, and exploiting the capabilities of the framework is crucial.
Additional Resources
To further solidify your understanding, consider reading:
Mastering these key concepts and practical applications will not only prepare you for interviews but also enhance your day-to-day coding in Java. Stay curious and keep coding!