Struggling with Java 8 Lambda Expressions? Here’s Help!
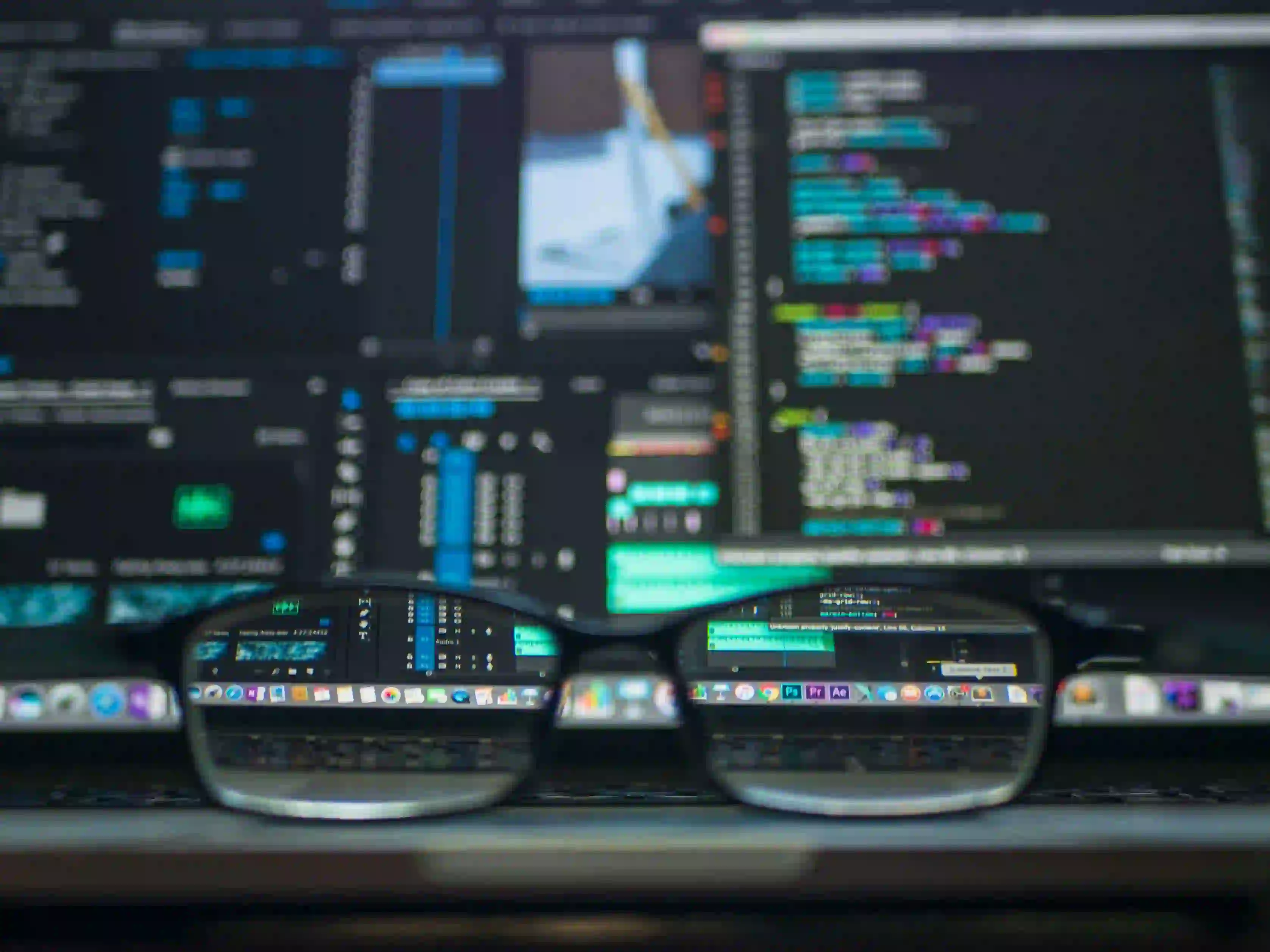
Struggling with Java 8 Lambda Expressions? Here’s Help!
Java 8 introduced a revolutionary feature known as Lambda Expressions that changed the way we write code in Java. Lambda expressions allow you to treat functionality as a method argument, or to create a concise syntax for writing anonymous methods.
In this blog post, we will go deep into the world of Lambda Expressions in Java 8, explain their usefulness, provide code examples, and clarify any uncertainties you may have regarding this feature.
What is a Lambda Expression?
A lambda expression is essentially a block of code that you can pass around as if it were an object. It represents a parameterized interface, particularly the functional interfaces.
A functional interface is defined as an interface that consists of a single abstract method. A lambda expression is composed of three key parts:
- Parameters - If the functional interface has a method that takes parameters, specify them.
- Arrow Operator - This separates the parameters from the body of the implementation.
- Body - This contains the actual implementation.
Example of a Lambda Expression
Here’s a simple example to illustrate a lambda expression in action. Let's say we want to create a thread that prints numbers from 1 to 5.
Runnable runnable = () -> {
for (int i = 1; i <= 5; i++) {
System.out.println(i);
}
};
new Thread(runnable).start();
Why This Code is Useful:
- The
Runnable
interface is a functional interface with a single abstract methodrun
. - The lambda expression simplifies the creation of an instance of
Runnable
. - This syntax is more concise and readable than creating an anonymous class to implement
Runnable
.
Why Use Lambda Expressions?
1. More Concise Code
Lambda expressions allow you to reduce boilerplate code. That means less code to write, read, and maintain.
Before Java 8:
new Thread(new Runnable() {
public void run() {
System.out.println("Hello from a thread!");
}
}).start();
After Java 8:
new Thread(() -> System.out.println("Hello from a thread!")).start();
2. Improved Readability
Developers can easily understand what the code is doing, thanks to the reduced clutter. The meaning of the code can be derived directly from its structure, which is straightforward.
3. Scope and Closure
Lambda expressions can access variables from their enclosing scope, also known as closure. This can be quite useful.
String message = "Hello, World!";
Runnable runnable = () -> System.out.println(message);
new Thread(runnable).start();
In the code snippet above, the lambda expression captures the message
variable. This is powerful as it allows you to pass context with your expressions easily.
Common Use Cases of Lambda Expressions
Streaming APIs
One of the most profound uses of lambda expressions is with the Stream API, which allows functional-style operations on streams of elements.
Let's say you have a list of integers and you want to filter out the odd numbers and double them.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
List<Integer> doubledEvens = numbers.stream()
.filter(n -> n % 2 == 0)
.map(n -> n * 2)
.collect(Collectors.toList());
System.out.println(doubledEvens);
Why This Matters:
- The above code creates a pipeline for processing data using methods like
filter
andmap
, significantly improving the expressiveness of your code. - Lambda expressions enhance readability and allow chaining operations, making data processing easier to grasp and maintain.
Event Handling in GUI Applications
Lambda expressions can simplify code in GUI applications. For instance, if you are handling button clicks in a JavaFX application, using a lambda can eliminate the need for an entire anonymous class.
button.setOnAction(e -> System.out.println("Button clicked!"));
Best Practices with Lambda Expressions
-
Keep it Simple: Lambda expressions should remain simple. If you're writing a complex expression, consider using regular methods instead.
-
Prefer Method References: If the lambda expression is merely calling a method, prefer using method references for improved readability.
// Using method reference
List<String> names = Arrays.asList("John", "Jane", "Jack");
names.forEach(System.out::println);
- Type Inference: You often do not need to specify types for parameters explicitly; the compiler can infer them, so avoid redundancy.
Challenges with Lambda Expressions
While lambda expressions can simplify code, they can also lead to challenges:
-
Debugging: Since lambda expressions are essentially anonymous, debugging may become complicated without clear stack traces.
-
Over-usage: Overuse may lead to scattered code that could confuse readability instead of improving it. Use them where logical and semantically appropriate.
Final Considerations
Lambda expressions are a valuable addition to Java 8, promoting clean code and functional programming paradigms. If you haven't started using them yet, it’s time to explore this powerful feature.
For further reading and exploration, you may consider these resources for deep dives into Java and Lambda expressions:
By embracing lambda expressions, you can write cleaner, more efficient Java code that not only enhances your productivity but also makes your codebase far easier to understand and maintain. Happy coding!