Struggling with Moxy in JAX-RS? Simplify JSON Binding!
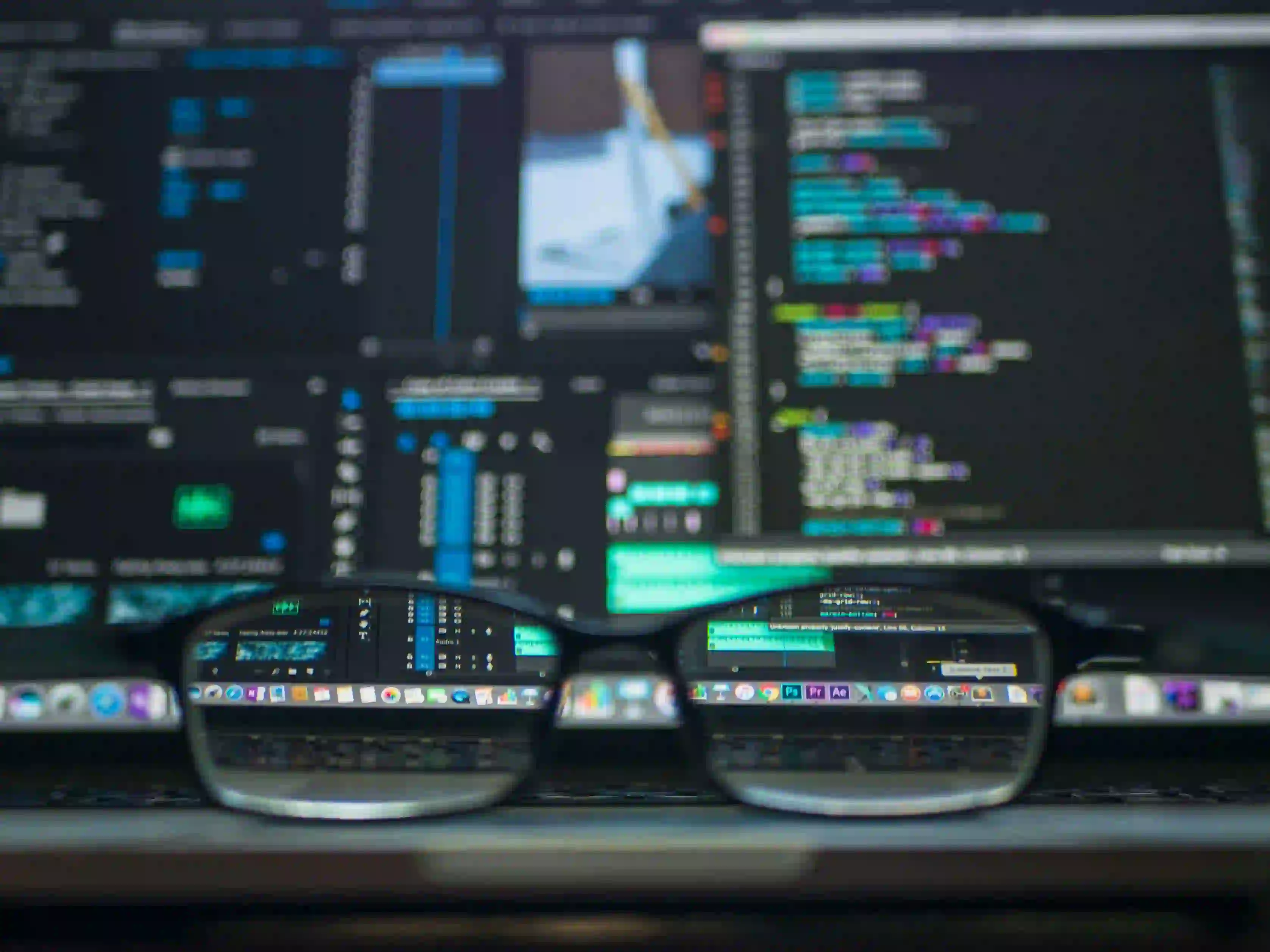
Struggling with Moxy in JAX-RS? Simplify JSON Binding!
When it comes to developing RESTful web services in Java, JAX-RS stands as a powerful and flexible framework. One of the common tasks that developers face in these services is JSON binding. MOXy (EclipseLink MOXy) offers a solution that simplifies and enhances JSON binding in JAX-RS applications.
In this post, we will explore how to effectively use MOXy for JSON binding in JAX-RS, enabling seamless serialization and deserialization of your Java objects. We will walk through the steps to set it up, provide examples, and illustrate best practices along the way.
What is JAX-RS?
JAX-RS, short for Java API for RESTful Web Services, is a set of APIs to create REST web services in Java. It offers tools and annotations to simplify the development process. With JAX-RS, developers can easily create endpoints, handle HTTP requests, and return responses in a structured way.
For detailed documentation, you can check Oracle's official JAX-RS documentation.
Let us Begin to MOXy
MOXy is EclipseLink's implementation of the JAXB (Java Architecture for XML Binding) specification, but it also provides support for JSON binding. It allows Java objects to be converted to and from JSON seamlessly.
Using MOXy can be beneficial because:
- It offers advanced JSON capabilities, such as JSON views and binding to XML.
- You can control the serialization and deserialization process through annotations.
Setting Up MOXy with JAX-RS
To get started with MOXy, you will need to include the necessary dependencies in your project. If you're using Maven, add the following to your pom.xml
:
<dependency>
<groupId>org.eclipse.persistence</groupId>
<artifactId>org.eclipse.persistence.moxy</artifactId>
<version>2.7.7</version> <!-- Check for the latest version -->
</dependency>
<dependency>
<groupId>javax.ws.rs</groupId>
<artifactId>javax.ws.rs-api</artifactId>
<version>2.1</version>
</dependency>
Configuring MOXy as Your JSON Provider
Once you have the dependencies set up, the next step is to configure MOXy as the JSON provider. This can be done in your JAX-RS configuration class as follows:
import org.eclipse.persistence.jaxb.JAXBContextFactory;
import org.glassfish.jersey.jackson.JacksonFeature;
import org.glassfish.jersey.server.ResourceConfig;
public class AppConfig extends ResourceConfig {
public AppConfig() {
packages("com.example"); // Specify your package
register(JacksonFeature.class); // Register Jackson feature if you're using it too
property("javax.ws.rs.ext.RuntimeType", "MOXy");
}
}
This class ensures that MOXy is used to handle JSON content effectively. It’s also wise to register Jackson if you want to support both serializers.
Example: JSON Binding with MOXy
Now, let’s dive into an example to understand how MOXy simplifies JSON binding.
First, define a simple Java class that represents a user:
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement
public class User {
private String name;
private int age;
public User() {
}
public User(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
Here, we’ve annotated the User
class with @XmlRootElement
, allowing it to be serialized into JSON format easily.
Next, create a resource class that exposes a REST API:
import javax.ws.rs.*;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
@Path("/users")
public class UserResource {
@POST
@Consumes(MediaType.APPLICATION_JSON)
@Produces(MediaType.APPLICATION_JSON)
public Response createUser(User user) {
// Logic to save user (omitted for brevity)
return Response.status(Response.Status.CREATED).entity(user).build();
}
@GET
@Produces(MediaType.APPLICATION_JSON)
public Response getUser() {
User user = new User("John Doe", 30);
return Response.ok(user).build();
}
}
With this setup, MOXy handles the conversion of User
objects to JSON automatically during the response phase and vice versa during the request phase.
Testing Your API
To test the API, you could use tools like Postman or curl.
-
POST Request: To create a new user:
🔧snippet.shcurl -X POST -H "Content-Type: application/json" -d '{"name": "Jane Doe", "age": 25}' http://localhost:8080/yourapp/users
-
GET Request: To retrieve a user:
🔧snippet.shcurl -X GET http://localhost:8080/yourapp/users
You should see a JSON representation of the user response.
Advanced Annotations
MOXy also provides additional annotations that can help control the serialization process. Some notable ones include:
@XmlProperty
: To rename fields in the JSON output.@XmlPath
: To specify the JSON path to bind a field.@XmlAccessorType
: To redefine access control for properties.
Example with Annotations
Here is a modified version of the User
class with annotations:
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement(name = "user")
@XmlAccessorType(XmlAccessType.FIELD)
public class User {
@XmlAttribute
private String name;
@XmlAttribute
private int age;
// Constructors, getters, and setters
}
In this example, @XmlAccessorType(XmlAccessType.FIELD)
enables field-level access, and @XmlAttribute
marks the name and age fields as XML attributes, affecting the JSON output.
My Closing Thoughts on the Matter
Incorporating MOXy into your JAX-RS applications can significantly simplify the JSON binding process. It provides powerful features to efficiently serialize and deserialize Java objects.
By leveraging the annotations and capabilities MOXy offers, developers can maintain control over the generated JSON format while still enjoying the convenience of automatic binding. Whether you are creating new web services or enhancing existing ones, MOXy provides a robust solution for your JSON binding needs.
For more in-depth information about configuring and using MOXy, check out the EclipseLink MOXy documentation.
Further Reading
Feel free to dive deeper into these resources and explore the capabilities that MOXy can bring to your JAX-RS applications!