Mastering State Design Pattern: Common Implementation Pitfalls
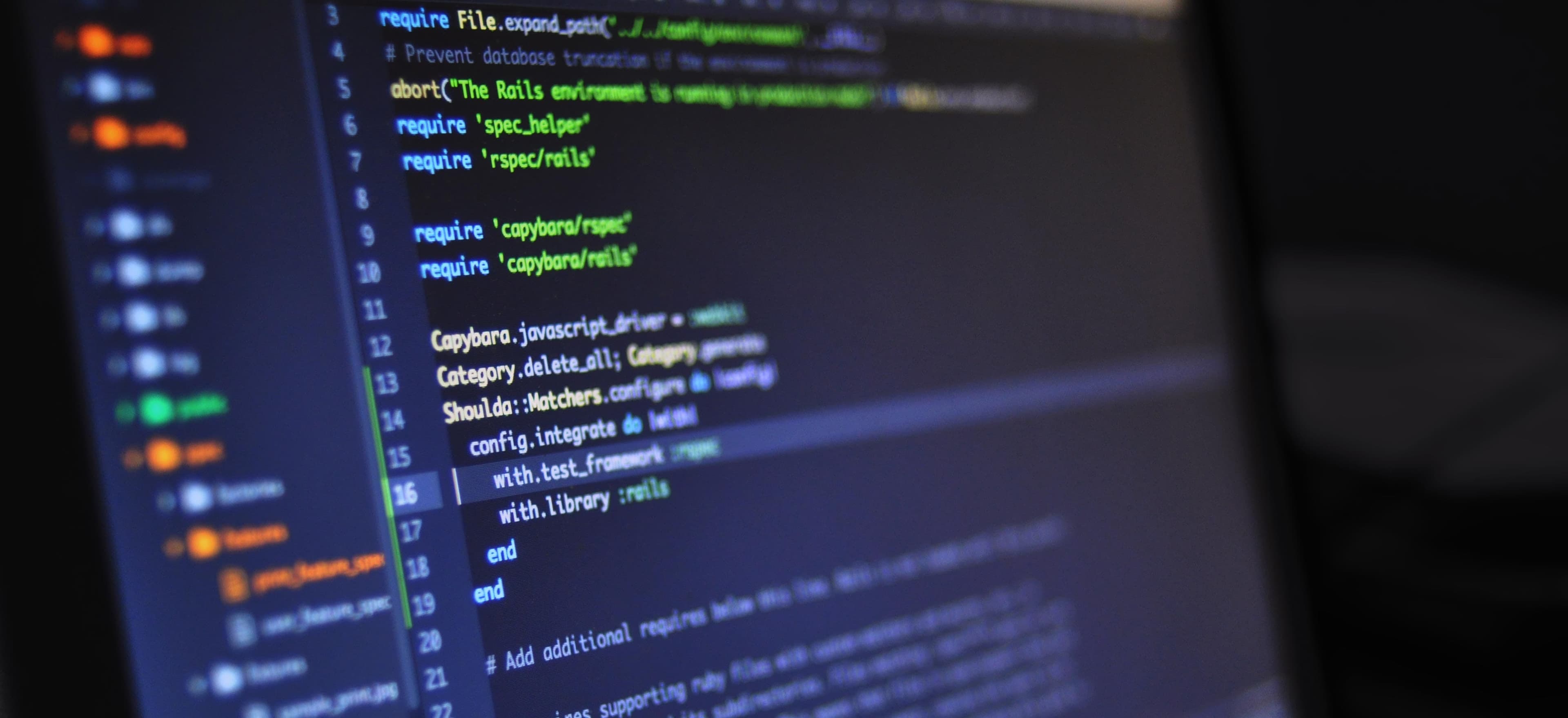
- Published on
Mastering the State Design Pattern: Common Implementation Pitfalls
The State Design Pattern is an essential tool for developers who want to manage an object's state effectively. It allows an object to change its behavior when its internal state changes. In other words, the object appears to change its class. Although this pattern can greatly enhance your code's flexibility and maintainability, there are common pitfalls that developers may encounter during implementation.
This article will delve into the State Design Pattern, explore its benefits, and discuss typical mistakes that can arise. We will also provide code snippets to showcase best practices, clarify concept intricacies, and improve your understanding of this pattern.
What is the State Design Pattern?
The State Pattern is a behavioral design pattern that enables an object to alter its behavior when its internal state changes. This is particularly useful when dealing with complex state-dependent behavior. Instead of extensive conditional statements, the pattern encapsulates state-specific behaviors in separate classes, which increases code clarity and maintainability.
Benefits of the State Design Pattern
-
Improved Maintainability: By breaking state-dependent behavior into distinct classes, your code becomes easier to understand and maintain.
-
Increased Flexibility: New states can be added without modifying existing code, adhering to the Open/Closed Principle of SOLID design principles.
-
Clearer Code: Each state's behavior is self-contained, which reduces the complexity often associated with large if-else conditionals.
Basic Structure of the State Design Pattern
Before we proceed to common pitfalls, let's outline the basic structure of the State Design Pattern. Here's a typical UML representation:
+---------+
| Context |
+---------+
|
| 1
|
| 1..*
+---------+
| State |
+---------+
/ \
+---------+ +---------+
| Concrete State A | Concrete State B |
+---------+ +---------+
Key Components
-
Context: This is the class that maintains a reference to the current state of the object.
-
State: This is an interface that defines the methods that should be implemented by concrete states.
-
Concrete States: These classes implement the state-specific behavior.
Implementation Example
Let's illustrate the State Design Pattern with a simple example of a Document that can be in different states: Draft, Moderation, and Published.
Interface and Context Class
// The State interface
public interface DocumentState {
void publish(Document context);
void moderate(Document context);
}
// The Context class
public class Document {
private DocumentState state;
public Document() {
this.state = new DraftState(); // Default state
}
public void setState(DocumentState state) {
this.state = state;
}
public void publish() {
state.publish(this);
}
public void moderate() {
state.moderate(this);
}
}
Concrete State Classes
// Concrete state for Draft
public class DraftState implements DocumentState {
@Override
public void publish(Document context) {
System.out.println("Publishing the document.");
context.setState(new PublishedState());
}
@Override
public void moderate(Document context) {
System.out.println("Moderating the document.");
context.setState(new ModerationState());
}
}
// Concrete state for Moderation
public class ModerationState implements DocumentState {
@Override
public void publish(Document context) {
System.out.println("Document is approved; publishing.");
context.setState(new PublishedState());
}
@Override
public void moderate(Document context) {
System.out.println("Document is already under moderation.");
}
}
// Concrete state for Published
public class PublishedState implements DocumentState {
@Override
public void publish(Document context) {
System.out.println("Document is already published.");
}
@Override
public void moderate(Document context) {
System.out.println("Cannot moderate a published document.");
}
}
Usage
Here's how you would use the Document
with the various states:
public class Client {
public static void main(String[] args) {
Document document = new Document();
// Publish the document
document.publish(); // Outputs: Publishing the document.
// Try to moderate the published document
document.moderate(); // Outputs: Cannot moderate a published document.
// Moderate the document while in Draft
document.setState(new DraftState());
document.moderate(); // Outputs: Moderating the document.
}
}
Common Implementation Pitfalls
1. Avoiding Circular State Transitions
When defining transitions between states, be cautious to avoid circular transitions that can lead to infinite loops. Ensure each state logically leads to another and that it allows for eventual completion of the lifecycle.
2. Overcomplicating State Behavior
Another common mistake is introducing unnecessary complexity in state behavior. Each state should have clear responsibilities. If a state is becoming too complex, it might be a sign that your design needs to be re-evaluated.
3. Not Handling State Transitions Properly
Failing to properly handle state transitions can lead to unexpected behavior. For instance, if a state allows transitioning back to another state that should only be reached in particular conditions, this can create logic errors.
4. Ignoring the Context State
Developers sometimes forget to update the Context object’s state, leading to scenarios where the expected state and the actual state are out of sync. Always ensure that you set the new state when transitioning to avoid such discrepancies.
5. Lack of Testing for Edge Cases
Make sure to thoroughly test your implementation, especially for edge cases, such as trying to transition states that shouldn't happen together. Regular testing can help catch these issues early.
Final Thoughts
The State Design Pattern is a powerful tool for managing state-specific behavior while maintaining clean and readable code. However, as we've seen, there are significant pitfalls to avoid.
By understanding the structure of the pattern and remaining conscious of common implementation errors, you can effectively use this pattern to improve your software design. Always remember the principles of maintainability, clarity, and proper handling of transitions.
For further reading and exploration, check out other resources on design patterns available at the links provided: Refactoring.Guru and SourceMaking.
By mastering the State Design Pattern and avoiding these pitfalls, you will elevate your Java programming skills and produce code that is both robust and elegant.
Feel free to ask if you have further questions on this topic! Happy coding!
Checkout our other articles