Debugging JVM CI: Navigating Heap Instantiation Issues
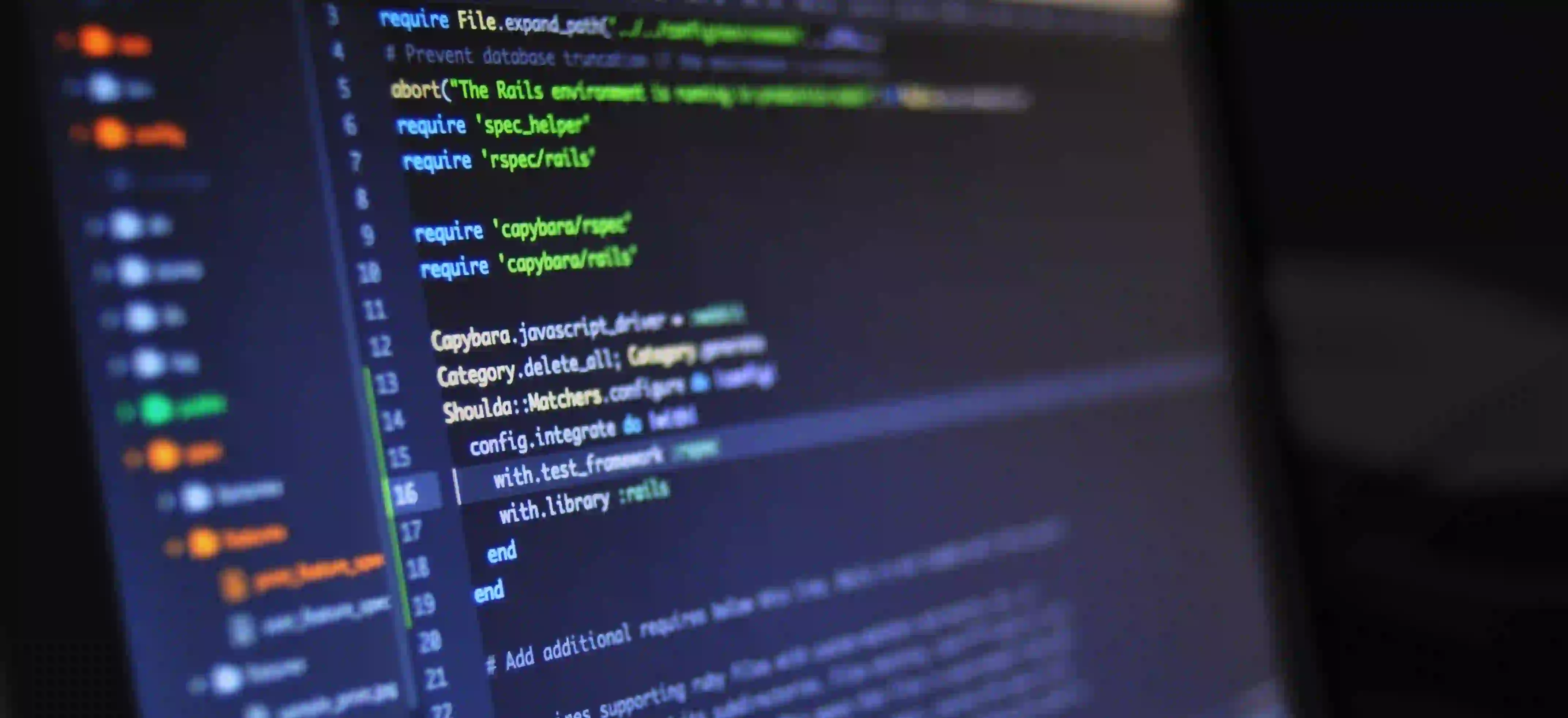
Debugging JVM CI: Navigating Heap Instantiation Issues
Debugging Java applications often uncovers various challenges, especially when running on the Java Virtual Machine (JVM). One recurring obstacle developers encounter is heap instantiation issues. These can manifest as memory leaks, OutOfMemoryErrors, or performance bottlenecks. In this blog post, we will explore how to diagnose and resolve heap instantiation issues in the JVM while offering code snippets to illustrate key concepts.
Understanding the JVM Heap
The JVM heap is a memory area where Java objects are allocated. It is created at the Java Virtual Machine startup and can be dynamically resized, though the upper limit can be set using flags like -Xmx
. There are two main areas of the heap:
- Young Generation: Newly created objects reside here first. If they survive garbage collection, they are promoted to the Old Generation.
- Old Generation: Objects that have survived multiple garbage collections are stored in this region.
The balance and management of these areas are crucial for performance. Inadequately tuned heap sizes could lead to either frequent garbage collections or exhaustion of memory.
Common Heap Instantiation Issues
-
OutOfMemoryError: When the JVM cannot allocate an object because it has run out of memory, it throws this error. Understanding your application's memory usage is crucial.
-
Memory Leaks: These occur when the application holds onto objects that are no longer needed, preventing them from being garbage collected.
-
Too Frequent Garbage Collections: If your heap is too small, the garbage collector will run too often, leading to performance issues.
Diagnosing the Problem
To effectively tackle heap instantiation issues, you first need to identify the root of the problem. Here are several debugging techniques.
1. Monitoring with JVM Options
To diagnose heap issues, start your JVM with monitoring options. The following flags provide insightful metrics:
java -Xms512m -Xmx2048m -XX:+PrintGCDetails -XX:+PrintGCTimeStamps -Xloggc:gc.log -jar your-application.jar
- Xms: Initial heap size.
- Xmx: Maximum heap size.
- XX:+PrintGCDetails: Provides detailed garbage collection logs.
- XX:+PrintGCTimeStamps: Logs timestamps for GC events.
- Xloggc: Specifies the log file for GC logs.
2. Visualizing Heap Usage
Using tools like VisualVM or JConsole allows you to visualize heap dumps. These tools provide a real-time overview of memory usage and can help identify potential memory leaks or improper memory usage.
Example of VisualVM Usage
-
Launch your Java application with remote monitoring enabled:
🔧snippet.shjava -Dcom.sun.management.jmxremote -jar your-application.jar
-
Open VisualVM and connect to your application to inspect heap usage.
3. Heap Dump Analysis
Generating a heap dump at the point of failure provides insight into current memory allocation states. You can create a heap dump automatically when you get an OutOfMemoryError by adding the following flag:
-XX:+HeapDumpOnOutOfMemoryError
Heap dumps can be analyzed using tools like Eclipse MAT (Memory Analyzer Tool).
Example Code to Trigger OutOfMemoryError
To help you see how heap instantiation issues can arise, consider the following Java snippet that intentionally causes an OutOfMemoryError
:
import java.util.ArrayList;
import java.util.List;
public class OutOfMemoryExample {
public static void main(String[] args) {
List<Object> memoryLeakList = new ArrayList<>();
while (true) {
memoryLeakList.add(new Object()); // Continually adds objects, causing memory to fill up
}
}
}
Commentary: This code creates a list that grows indefinitely. Each new object added to the list occupies space in the heap. Eventually, it will exhaust the available memory, demonstrating a memory leak scenario clearly.
4. Analyzing Application Code
Review the code for potential factors contributing to heap issues:
- Unclosed Resources: Always close file streams, database connections, and other resources.
- Static References: Static variables hold references for the life of the application. Use them judiciously.
- Collections: Unbounded collections can quickly fill the heap. Set capacity constraints when feasible.
Example: Fixing Static Reference Leaks
Here is an example of improper static reference usage:
public class Cache {
private static List<User> users = new ArrayList<>();
public static void addUser(User user) {
users.add(user); // Holds reference indefinitely, causing potential memory leak
}
}
Instead, consider weakening the reference:
public class Cache {
private static final Map<Integer, WeakReference<User>> users = new HashMap<>();
public static void addUser(Integer id, User user) {
users.put(id, new WeakReference<>(user)); // Allows garbage collection when no strong reference exists
}
}
Commentary: Using WeakReference
allows the User
objects to be reclaimed by the garbage collector when they are no longer in use, thereby preventing memory leaks.
Tuning the JVM for Optimal Performance
Setting Heap Sizes
To ensure your application runs smoothly, it's essential to tune the heap sizes according to the requirements:
java -Xms1g -Xmx4g -jar your-application.jar
Adjust Xms
and Xmx
values based on your application’s usage patterns.
Garbage Collector Selection
Different garbage collectors treat heap instantiation differently. The G1 garbage collector is often recommended for applications demanding low pause times:
java -XX:+UseG1GC -jar your-application.jar
In Conclusion, Here is What Matters
Debugging heap instantiation issues within the JVM is a multifaceted process requiring a mix of monitoring, analysis, and in-depth code reviews. Setting up the right JVM options, using diagnostic tools, and applying best coding practices are vital.
For further reading on heap memory management and garbage collection specifics, you can refer to the Java Performance Tuning Guide and the Java Memory Management documentation.
Remember, any preventive measures and optimizations can save you from runtime headaches, paving the way for a more efficient and robust Java application. Happy coding!