Balancing Java's Complexity: High-Level vs Low-Level Dilemma
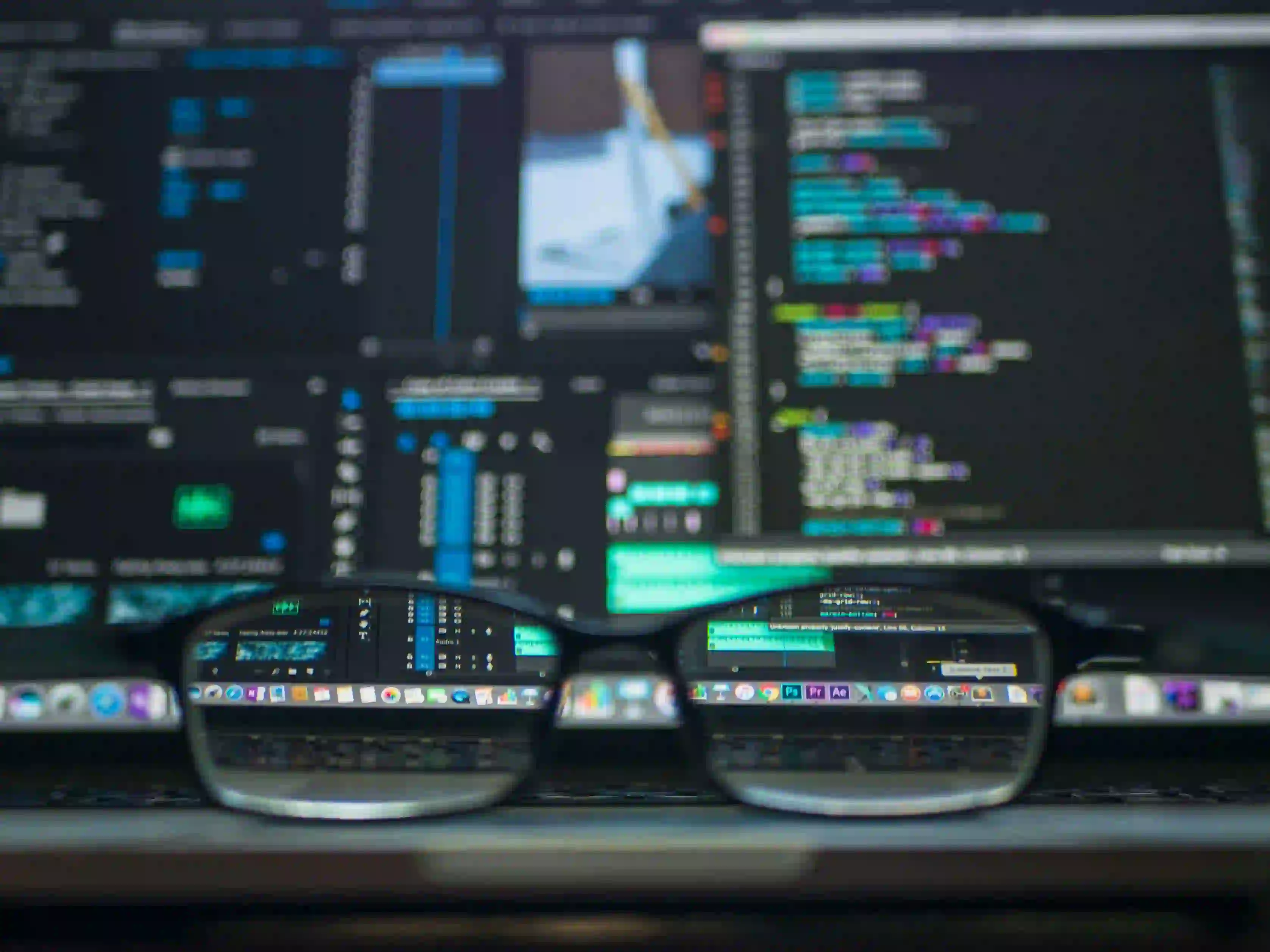
Balancing Java's Complexity: High-Level vs Low-Level Dilemma
Java is often praised for its portability, scalability, and robust memory management. However, it exists within a broad spectrum of complexity, oscillating between high-level abstractions and low-level mechanisms. This blog post will explore this high-level and low-level dilemma in Java, breaking down when to use each approach and how to balance them effectively.
Understanding High-Level and Low-Level Programming
High-Level Programming
High-level programming languages, like Java, offer abstractions that allow developers to write code efficiently without concerning themselves with the underlying hardware. High-level features include:
- Garbage Collection: Java automatically manages memory, reducing the risk of memory leaks.
- Object-Oriented Programming (OOP): Java employs principles such as inheritance, encapsulation, and polymorphism.
- Rich Libraries: Java comes with vast libraries and frameworks enabling rapid development.
Advantages of High-Level Programming:
- Reduced Development Time: Developers can focus more on the logic rather than the implementation details.
- Increased Readability: Code is easier to understand and maintain.
Disadvantages of High-Level Programming:
- Performance Overhead: Abstractions can lead to inefficiencies, impacting application performance.
- Debugging Complexity: Higher abstractions can sometimes obscure the underlying errors.
Low-Level Programming
Low-level programming typically refers to assembly or machine code but can also encompass certain aspects of Java, such as direct memory management using Unsafe
or handling threads and sockets manually.
Advantages of Low-Level Programming:
- Enhanced Performance: Fine-tuning performance-critical applications becomes more feasible.
- Greater Control: Developers can optimize specific components of their applications.
Disadvantages of Low-Level Programming:
- Longer Development Time: It requires more attention to detail, extending the development cycle.
- Increased Risk of Bugs: More control means more responsibility. Bugs become harder to trace and fix.
The Balancing Act
In a practical sense, developers often need to balance high-level and low-level programming. Here, we can analyze a few real-world scenarios and utilize some code snippets to illustrate critical points.
Scenario 1: Developing a CRUD Application
When you are building a web application like a CRUD (Create, Read, Update, Delete) application, high-level frameworks such as Spring Framework or Java EE are highly beneficial.
@RestController
@RequestMapping("/api/users")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/{id}")
public ResponseEntity<User> getUserById(@PathVariable Long id) {
User user = userService.findById(id);
return ResponseEntity.ok(user);
}
}
This code snippet shows how Spring handles HTTP requests. The @RestController
annotation indicates that this class contains methods that will handle web requests. Notice how we are abstracted away from lower-level HTTP handling, allowing us to focus purely on business logic.
Takeaway:
Using a high-level framework like Spring increases productivity, making development faster and easier to maintain.
Scenario 2: Performance-Critical Applications
If you are working on a real-time application where performance is paramount (like a game engine or high-frequency trading system), you may need to interact with lower-level Java capabilities.
public class UnsafeDemo {
private static final sun.misc.Unsafe unsafe = getUnsafe();
private static sun.misc.Unsafe getUnsafe() {
try {
Field field = sun.misc.Unsafe.class.getDeclaredField("theUnsafe");
field.setAccessible(true);
return (sun.misc.Unsafe) field.get(null);
} catch (Exception e) {
throw new RuntimeException("Unable to access Unsafe");
}
}
public void exampleMemoryAccess() {
long address = unsafe.allocateMemory(4L);
unsafe.putInt(address, 12345);
int value = unsafe.getInt(address);
unsafe.freeMemory(address);
System.out.println("The value is: " + value);
}
}
In this snippet, we use the Unsafe
class to perform low-level memory manipulation. While this offers high performance and control, it also comes at the cost of safety and maintainability.
Takeaway:
Use low-level approaches sparingly, focusing on specific bottlenecks in performance-critical applications.
The Middle Ground: Best Practices
When to Choose High-Level or Low-Level
- Use high-level abstractions when prototyping, developing business applications, or when your team is less experienced.
- Opt for low-level optimizations when dealing with performance constraints that demand precise resource management.
Maintainability and Readability
Balance is also essential for maintainability and readability. Here are a few best practices:
- Use Interfaces: By defining interfaces, you can lean towards high-level programming while allowing for low-level implementations to optimize performance as necessary.
public interface UserRepository {
User findUserById(Long id);
void saveUser(User user);
}
-
Encapsulation: Always aim to encapsulate low-level code behind high-level interfaces. This encapsulation minimizes the complexity presented to other developers.
-
Documentation: Make sure that both high-level and low-level code is well-documented, particularly the low-level operations that can complicate understanding.
Leveraging Libraries and Frameworks
Utilize well-established libraries and frameworks that often abstract low-level processes under the hood. For instance, Apache Commons provides useful utilities while managing complexities internally.
Monitoring and Profiling
Regardless of the level of abstraction chosen, profiling and monitoring are essential. Tools such as Java Mission Control and VisualVM can help identify bottlenecks in your application.
Final Considerations
Java's versatility enables a balanced approach between high-level and low-level programming. Recognize the strengths and weaknesses of both approaches, and adapt your strategy based on application requirements.
In an era where performance, maintainability, and rapid development are essential, mastering the art of balancing high-level abstractions with low-level code will empower you to make smarter decisions that enhance both the codebase and the user experience.
By embracing both high-level frameworks for productivity and low-level techniques for performance, you can elevate your Java applications to new heights while ensuring clarity and maintainability.
If you're looking for more resources on Java development, consider checking out the Java Tutorials provided by Oracle or engaging with the vibrant community on Stack Overflow. Keep learning and coding!