Overcoming Complexity: API Gateway Challenges in Microservices
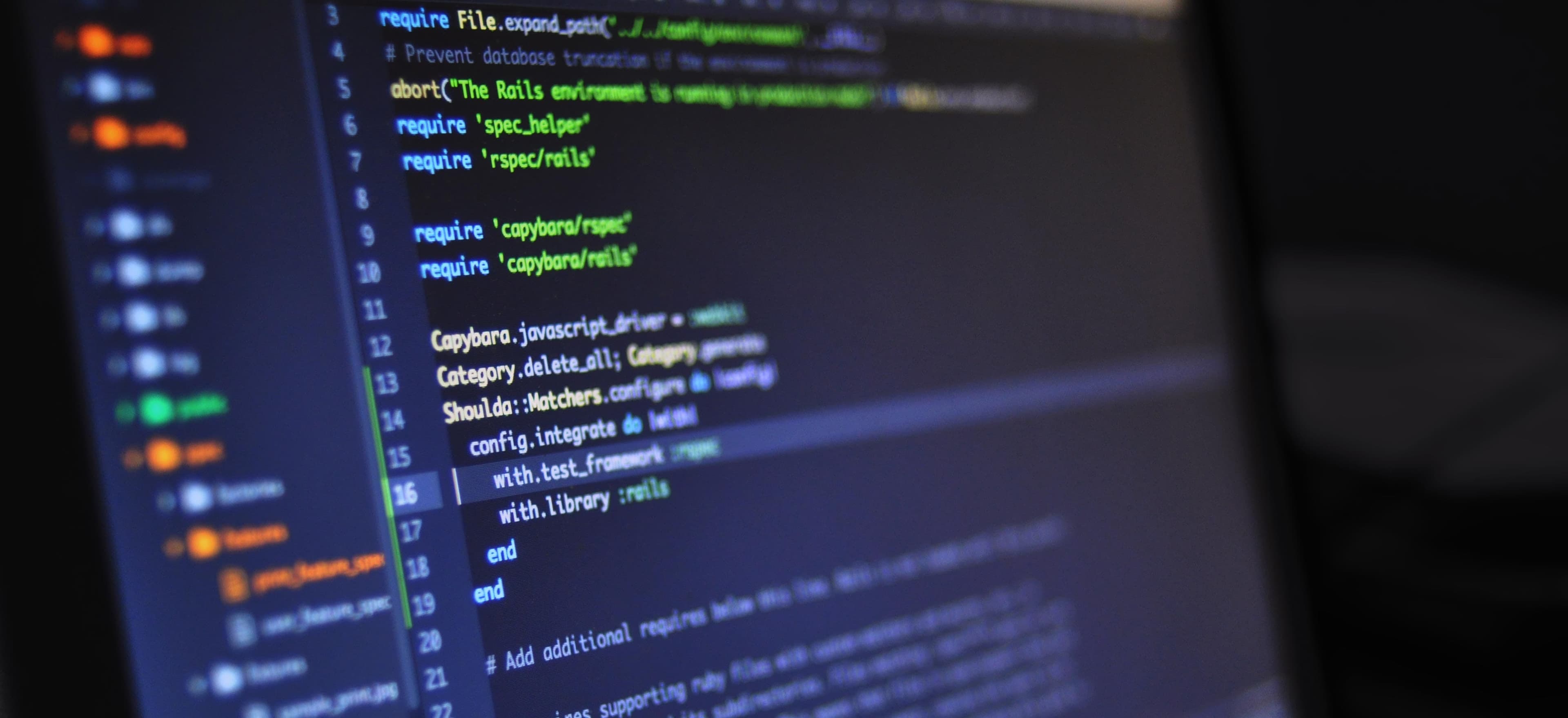
- Published on
Overcoming Complexity: API Gateway Challenges in Microservices
In the microservices architecture, one of the key components that enable efficient communication between services is the API Gateway. Although it serves a crucial role, the implementation and management of an API gateway come with their own set of challenges. In this blog post, we will explore these challenges, provide valuable insights, and offer practical solutions to help you navigate through them.
What is an API Gateway?
An API Gateway acts as a single entry point for an application with multiple microservices. It abstracts the underlying architecture, reducing complexity for clients while also handling cross-cutting concerns such as security, rate limiting, load balancing, caching, and logging. In essence, the API Gateway helps manage API requests and responses, directing them to the appropriate microservice in the background.
Challenges of API Gateway in Microservices
While the advantages of using an API Gateway are clear, implementing it effectively can be daunting. Here are some common challenges one might face:
1. Single Point of Failure
One of the most significant challenges of using an API Gateway is that it becomes a single point of failure in the system. If the gateway goes down, clients are unable to access any services. This makes high availability and load balancing crucial.
Solution: Redundancy and Load Balancing
Implement redundancy by deploying multiple instances of the API Gateway across different locations. You can use a load balancer to distribute incoming traffic among these instances, ensuring that if one fails, others can take over.
public class LoadBalancer {
private List<String> servers;
public LoadBalancer() {
this.servers = Arrays.asList("Server1:8080", "Server2:8080");
}
public String getServer() {
Random rand = new Random();
return servers.get(rand.nextInt(servers.size()));
}
}
// Usage:
LoadBalancer lb = new LoadBalancer();
String selectedServer = lb.getServer();
2. Increased Latency
Adding an API Gateway introduces an additional network hop, potentially increasing latency. This effect can be magnified with multiple service calls, common in microservices.
Solution: Optimizing API Calls
You can reduce latency by using techniques like request aggregation and response transformation. Instead of making multiple API calls, aggregate them at the gateway level.
@RequestMapping("/aggregate")
public ResponseEntity<ComplexResponse> aggregateRequests() {
Future<ResponseEntity<ServiceResponse1>> service1Response = callService1Async();
Future<ResponseEntity<ServiceResponse2>> service2Response = callService2Async();
return ResponseEntity.ok(aggregateResponses(service1Response.get(), service2Response.get()));
}
3. Security Concerns
The API Gateway must deal with various security concerns, including authentication and authorization. With multiple services behind the gateway, it can be a challenge to ensure each service has the correct access control.
Solution: Centralized Security Measures
Implement centralized security mechanisms like OAuth2 or API keys. Ensure that all services behind the API Gateway rely on these mechanisms for authentication.
@RestController
public class AuthController {
@PostMapping("/authenticate")
public ResponseEntity<String> authenticate(@RequestBody AuthRequest authRequest) {
String token = authenticationService.authenticate(authRequest);
return ResponseEntity.ok(token);
}
}
4. Complexity in Configuration Management
Managing configurations for multiple microservices can be challenging. A misconfiguration in the API Gateway can lead to service failures.
Solution: Consistent Configuration Management
Utilize centralized configuration management tools like Spring Cloud Config or HashiCorp Vault. These tools allow you to manage settings and make them consistent across different environments.
5. Monitoring and Logging Overhead
Every microservices architecture needs observability. When introducing an API Gateway, you need to be able to log and monitor traffic effectively, which can add complexity.
Solution: Distributed Tracing and Centralized Logging
Incorporate distributed tracing solutions like Jaeger or Zipkin to track the flow of requests across the services. Centralized logging tools like ELK (Elasticsearch, Logstash, and Kibana) can help in analyzing and visualizing logs.
@GetMapping("/trace/{id}")
public TraceResponse getTrace(@PathVariable String id) {
return tracingService.getTraceById(id);
}
Best Practices for API Gateway Management
After addressing the challenges, let's discuss some best practices for managing your API Gateway effectively:
1. API Versioning
Versioning of APIs is essential to ensure that changes do not break existing clients. Use meaningful version identifiers in your endpoints.
@GetMapping("/v1/users")
public List<User> getUsersV1() {
return userService.getAllUsers();
}
2. Rate Limiting
To prevent abuse and ensure fair usage, implement rate limiting at the gateway level.
@RequestMapping("/users")
@RateLimit(limit = 100, duration = 60)
public List<User> getUsers() {
return userService.getAllUsers();
}
3. Documentation
Maintaining clear documentation of your API Gateway and its services is imperative. Tools like Swagger/OpenAPI can facilitate clear, interactive documentation.
4. Continuous Integration/Continuous Deployment
Use CI/CD pipelines to automate the deployment of your API Gateway. This ensures that any changes are consistently tested and deployed.
When to Use an API Gateway
An API Gateway is most beneficial in scenarios where:
- Your application has multiple microservices.
- You require centralized control over traffic.
- Security is a concern, and centralized authentication is necessary.
- You want to aggregate responses and reduce the number of client calls.
Closing Remarks
Navigating the complexities of API Gateways in microservices architecture requires a keen understanding of the challenges involved as well as an eye for best practices. By implementing strategies like redundancy, centralized security, and effective monitoring, you can ensure that your API Gateway serves as a robust facilitator of communication between microservices while minimizing bottlenecks and vulnerabilities.
For further reading, you can check out the Microservices Architecture or explore API Gateway Explained for a deeper dive into the topic.
With careful planning and implementation, you can overcome the complexities of an API Gateway and reap the benefits of a microservices architecture, creating scalable, maintainable, and resilient systems.
Checkout our other articles