Solving ClassLoader Conflicts in Java Dynamic Loading
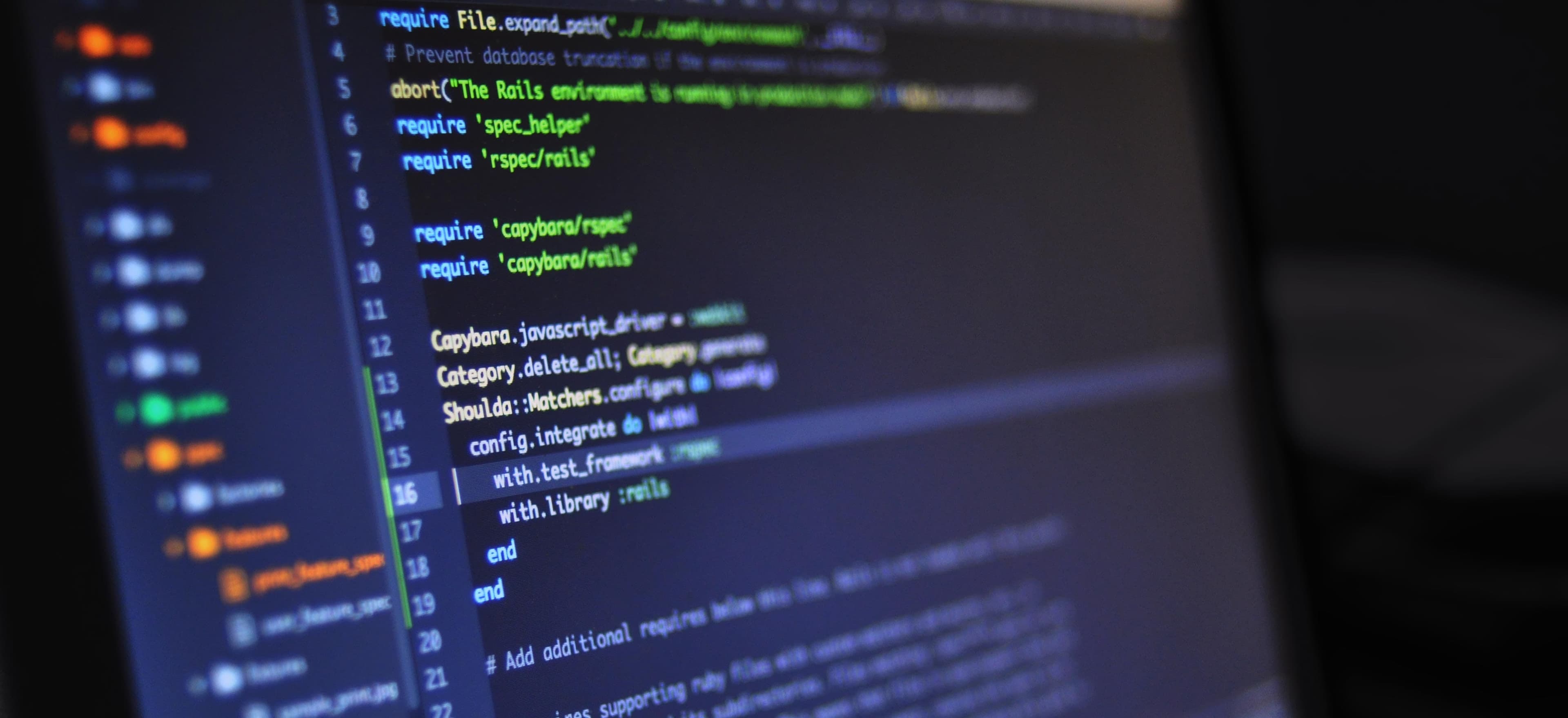
- Published on
Solving ClassLoader Conflicts in Java Dynamic Loading
Java's class loading mechanism is one of its most powerful features, allowing developers to dynamically load, link, and instantiate classes at runtime. However, with this power comes complexity, and ClassLoader conflicts can frequently occur. This blog post will discuss the nature of ClassLoader conflicts, how they arise, and most importantly, provide solutions to effectively manage and resolve these issues.
Understanding ClassLoaders in Java
Java uses a hierarchy of ClassLoaders, which are responsible for loading classes into memory. The Java Runtime Environment (JRE) has a built-in class loader architecture that includes:
- Bootstrap ClassLoader: The parent of all ClassLoaders, responsible for loading core Java classes from the Java Runtime Environment.
- Extension ClassLoader: Loads classes from the JDK extension directory (jre/lib/ext).
- Application ClassLoader: The default ClassLoader to load classes from the application's classpath.
These ClassLoaders work together in a parent-child delegation model. When a ClassLoader is asked to load a class, it first delegates the request to its parent. This mechanism is crucial to avoid loading multiple versions of the same class.
What are ClassLoader Conflicts?
ClassLoader conflicts arise when the same class is loaded by different ClassLoaders, leading to class version issues. This is often the case in complex applications, especially those using:
- Modular architectures (e.g., OSGi)
- Java EE applications (e.g., using different web applications within the same server)
- Dynamic Class Loading (e.g., using reflection)
Common Symptoms of ClassLoader Conflicts
- ClassCastException: You may receive a
ClassCastException
when trying to cast an object to a specific class because the runtime identifies it as a different class. - NoClassDefFoundError: This happens when your code tries to reference a class that was found but could not be initialized.
- LinkageError: This error may occur due to classes being incompatible, even though they were supposedly the same.
Example of ClassLoader Conflicts
Let's consider an example. Suppose you have a library loaded by two different ClassLoaders.
Code Snippet
// Class A in Library A
public class A {
public void display() {
System.out.println("Class A");
}
}
// Main class for loading dynamically
public class Main {
public static void main(String[] args) throws Exception {
URLClassLoader loader1 = new URLClassLoader(new URL[] {new File("path/to/libraryA").toURI().toURL()});
URLClassLoader loader2 = new URLClassLoader(new URL[] {new File("path/to/libraryA").toURI().toURL()});
Class<?> classA1 = loader1.loadClass("A");
Class<?> classA2 = loader2.loadClass("A");
// This will throw ClassCastException
Object objA = classA1.newInstance();
A a = (A) objA; // Attempting to cast to class loaded by a different ClassLoader
}
}
In the above code, the class A
is being loaded by two different class loaders, leading to a ClassCastException
when casting.
Solutions to ClassLoader Conflicts
1. Use a Shared ClassLoader
One of the most effective strategies is to use a shared ClassLoader. This means ensuring that your classes or libraries are loaded by the same ClassLoader whenever possible. By doing this, you prevent duplicate class loading.
// Use a common parent ClassLoader
ClassLoader sharedLoader = Main.class.getClassLoader();
Class<?> classA1 = sharedLoader.loadClass("A");
Class<?> classA2 = sharedLoader.loadClass("A"); // Same ClassLoader
2. Isolate Dependencies
In modern applications, especially microservices architectures, it can be beneficial to isolate dependencies. Tools like Maven and Gradle allow you to manage dependencies effectively.
- Use the
<scope>
tag in Maven to limit visibility to classes needed for your build. - In Gradle, use implementation and compileOnly configurations.
These tools help control which classes are included in each ClassLoader’s namespace.
3. ClassLoader Hierarchy
Understanding and utilizing ClassLoader hierarchy can mitigate conflicts. When more than one ClassLoader is in use, ensure that your custom ClassLoader overrides the default behavior elegantly.
public class CustomClassLoader extends ClassLoader {
@Override
protected Class<?> findClass(String name) throws ClassNotFoundException {
// Custom logic to find and define class
}
}
4. Java EE Best Practices
In Java EE environments, conflicts frequently arise from deploying multiple versions of the same library across different applications.
- Use shared libraries: Place shared libraries in a common location, often referred to as
lib
. - Avoid app server-provided libraries: Be mindful of JARs that may already exist in the server's library and ensure you're not unintentionally overriding them.
5. ClassLoader Leak Prevention
ClassLoader leaks can inadvertently keep classes from being garbage collected. To prevent leaks, unregister any classes or listeners on application shutdown and clean up custom ClassLoader references.
@Override
public void contextDestroyed(ServletContextEvent sce) {
// Cleanup logic
this.customClassLoader = null;
}
The Last Word
ClassLoader conflicts in Java dynamic loading pose challenges that can be resolved with thoughtful design and code organization. By understanding how ClassLoaders function and implementing best practices, developers can minimize conflicts, leading to cleaner, more maintainable code.
For further reading on ClassLoader mechanisms and conflicts, consider checking out Oracle's Java ClassLoader documentation and the Java Tutorials.
By applying these principles, you can enhance your Java applications' reliability and maintainability, ensuring a smoother operational experience.
Checkout our other articles