Struggling to Create Realistic Mock Data? Here's How!
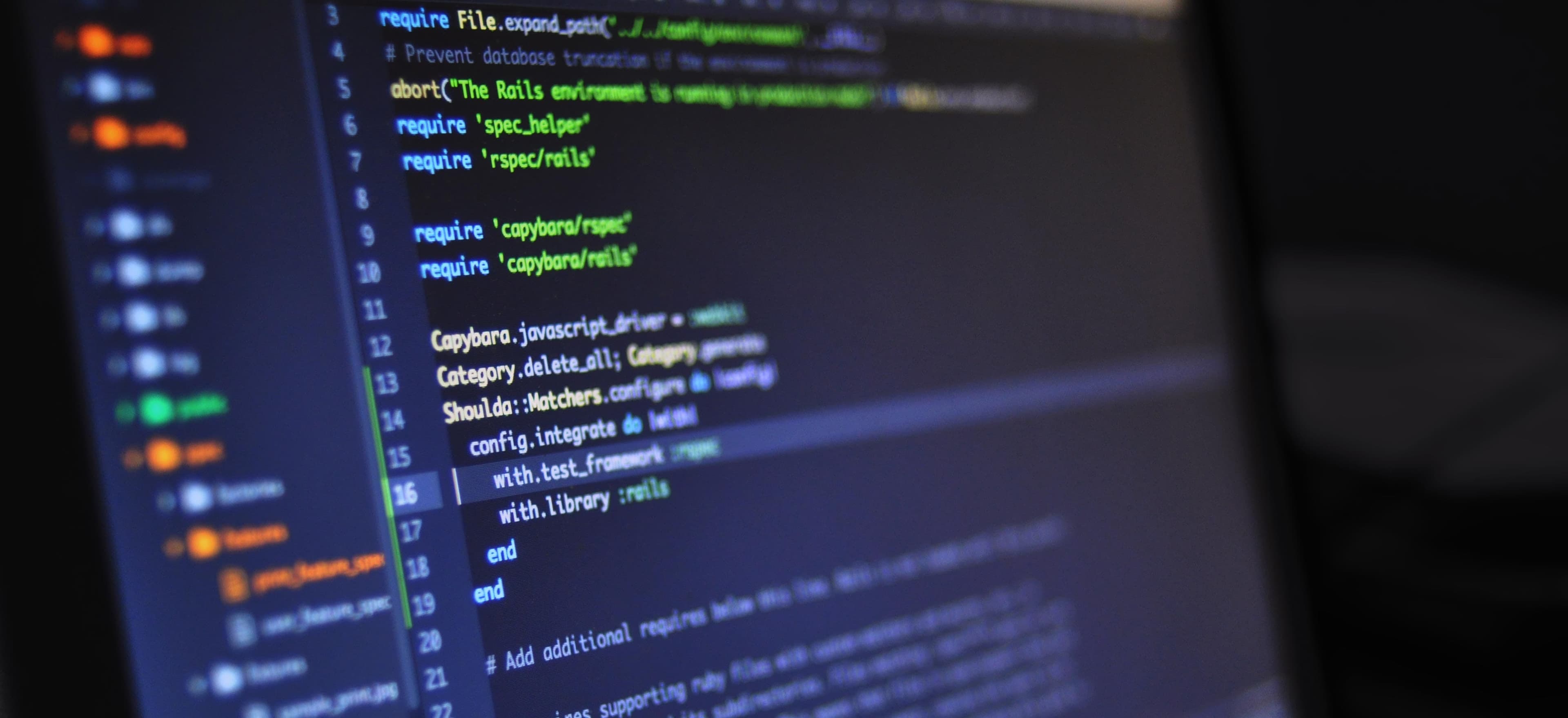
- Published on
Struggling to Create Realistic Mock Data? Here's How!
When developing applications or conducting tests, having realistic mock data is crucial. Not all of us are data scientists, so you might find yourself wondering: "How do I generate realistic mock data that can be used effectively in my projects?" You are not alone in this quest!
In this post, we will dive deep into techniques, libraries, and best practices for creating realistic mock data, particularly in Java. By the end of this article, you should be able to effectively generate mock data for your applications, ensuring a smooth and efficient development and testing process.
Why Do You Need Realistic Mock Data?
Realistic mock data is essential for several reasons:
- Testing: It allows you to evaluate how your application performs under various scenarios, including edge cases.
- Development: It provides a rich context for UI development, ensuring that features can be tested in a closer-to-real environment.
- Demonstrations: It enables you to showcase your application's functionality without needing access to production data.
Generating Mock Data: Key Techniques
Creating realistic mock data comes down to various techniques. These include hard-coded data generation, using libraries, and database seeding.
1. Hard-Coded Data Generation
Though tedious, hard-coding mock data is straightforward. Here's a simple example in Java:
public class UserMockData {
public String getUserData() {
return "John Doe, johndoe@example.com, 29";
}
}
While simple, hard-coded data can quickly become infeasible—it's limited, less flexible, and not very maintainable. For small projects, it might suffice, but let's explore more dynamic options.
2. Using Libraries
Several libraries can significantly simplify mock data generation in Java. Two popular libraries are:
- Java Faker: A library for generating fake data.
- Mockaroo: An online tool that allows users to generate mock data in various formats.
Example with Java Faker
Using the Java Faker library is an easy way to generate more robust mock data.
- Include the Dependency
If you're using Maven, include the following in your pom.xml
:
<dependency>
<groupId>com.github.javafaker</groupId>
<artifactId>javafaker</artifactId>
<version>1.0.2</version>
</dependency>
- Generate Data
Here's a basic example of how to generate user data using Java Faker:
import com.github.javafaker.Faker;
public class UserMockData {
public static void main(String[] args) {
Faker faker = new Faker();
String name = faker.name().fullName();
String email = faker.internet().emailAddress();
int age = faker.number().numberBetween(18, 60);
System.out.println("Name: " + name);
System.out.println("Email: " + email);
System.out.println("Age: " + age);
}
}
Why Use Java Faker?
Using a library like Java Faker allows for:
- Variety and randomness
- Different locales, which makes the data culturally diverse
- Quick setup with minimal effort
3. Database Seeding
If your application uses a database, consider seeding it with mock data. This approach allows for contextually rich datasets directly reflecting your application's structure.
Example of Database Seeding in Spring Boot
Suppose you are using Spring Boot with JPA. You can create entities and populate the database on startup:
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import org.springframework.data.jpa.repository.JpaRepository;
@Entity
public class User {
@Id
@GeneratedValue
private Long id;
private String name;
private String email;
private int age;
// standard getters and setters
}
public interface UserRepository extends JpaRepository<User, Long> {
}
import org.springframework.beans.factory.annotation.Autowired;
import javax.annotation.PostConstruct;
public class UserDataSeeder {
@Autowired
private UserRepository userRepository;
@PostConstruct
public void init() {
// Simulate data population
for (int i = 0; i < 10; i++) {
User user = new User();
user.setName("User " + (i + 1));
user.setEmail("user" + i + "@example.com");
user.setAge(20 + (i % 10));
userRepository.save(user);
}
}
}
Why Database Seeding?
Seeding a database with mock data allows you to test your application's interaction with the data layer. This approach helps ensure that your application behaves as expected in realistic scenarios.
Best Practices for Mock Data Generation
Here are some best practices to consider:
- Data Diversity: Ensure that your mock data covers a range of cases, including edge cases. The more diverse your data, the more realistic your testing.
- Structure Replication: Be precise in mimicking the fields and relationships in your actual data models.
- Reproducibility: Keep your mock data generation simple and repeatable so you can regenerate it as needed.
- Documentation: Document how your mock data was generated. This step can aid later development or testing phases.
Lessons Learned
In this article, we've explored how to create realistic mock data using different techniques and libraries in Java. From simple hard-coded examples to robust libraries and database seeding, each method has its benefits and use cases.
The journey to generate effective mock data is manageable when equipped with the right tools and understanding. By applying the techniques outlined here, you can enhance your application development and testing process significantly.
For additional reading and resources, check out these links:
With a solid foundation in mock data generation, you are now ready to tackle real-world challenges head-on!
Checkout our other articles