Troubleshooting Common Selenium UI Test Failures
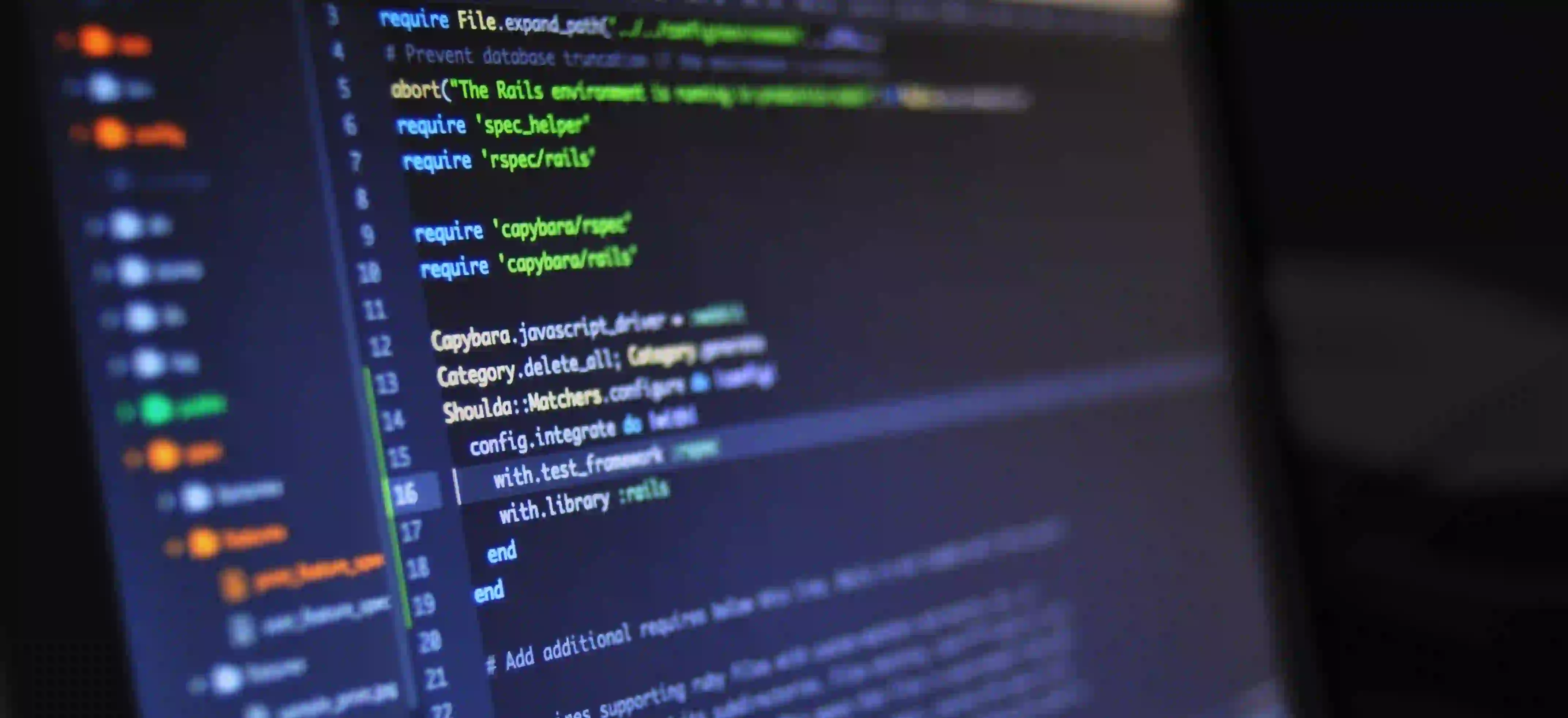
Troubleshooting Common Selenium UI Test Failures
Selenium is a powerful tool for automating web applications for testing purposes, but like any technology, it is not free from problems. Even the most seasoned developers encounter issues while using Selenium for UI testing. This blog post will cover common failures experienced during UI tests with Selenium and provide troubleshooting tips that will help you address these challenges effectively.
Table of Contents
- Understanding Selenium UI Testing
- Common Selenium UI Test Failures
- Practical Troubleshooting Tips
- Conclusion
Understanding Selenium UI Testing
Selenium is an open-source framework that allows developers and testers to write tests in various programming languages such as Java, Python, Ruby, and JavaScript. It interacts with web browsers just like a real user would, performing actions like clicking buttons, entering text, and navigating through pages.
When conducting UI tests, developers often use Selenium to ensure that the user interface behaves as expected. Despite Selenium's capabilities, various challenges may arise, leading to failures in the test execution process. This requires a keen understanding of the underlying issues.
Common Selenium UI Test Failures
Element Not Found
One of the most prevalent errors encountered with Selenium is when the script cannot locate an element on the web page, resulting in a NoSuchElementException
. This may occur for several reasons:
- Element is not yet loaded: If the element takes time to appear, Selenium may run the command before it is ready.
- Wrong Locator Strategy: The locator you are using (XPath, CSS Selector, etc.) may not accurately point to the element.
Example
WebElement loginButton = driver.findElement(By.id("login-button"));
loginButton.click();
Commentary: Ensure the ID is correct and the button exists on the page when the script is executed. You can implement an explicit wait to handle the timing issues.
Element Not Interactable
Sometimes, Selenium finds the element, but it is not clickable. This happens because the element may be obscured by another element, not visible on the screen, or not enabled yet.
- Obscured Elements: Use of modals, overlays, or CSS effects could prevent interaction.
Example
WebElement continueButton = driver.findElement(By.cssSelector(".continue"));
((JavascriptExecutor) driver).executeScript("arguments[0].click();", continueButton);
Commentary: Using JavaScript to perform the click circumvents issues stemming from overlays.
Stale Element Reference
A StaleElementReferenceException occurs when the web element that your Selenium test is trying to interact with is no longer present in the DOM (Document Object Model). This often happens during page reloads or dynamic content changes.
- Dynamic Content: For single-page applications, elements may change frequently.
Example
WebElement dynamicElement = driver.findElement(By.id("dynamic"));
String elementText = dynamicElement.getText();
Commentary: If the dynamic element updates after it is located, you must re-fetch the element after the page refresh or update.
Timeout Errors
Selenium allows you to set implicit and explicit waits. If a page takes longer than expected to load, you could run into TimeoutException
.
- Server-side Delays: Sometimes network latency or server response times affect element availability.
Example
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("delayed-element")));
Commentary: An explicit wait allows the script to pause until the condition is met. This can effectively handle elements that take time to be displayed.
Practical Troubleshooting Tips
-
Use Explicit Waits: Rely on explicit waits for elements that take time to appear. This can significantly reduce failure rates caused by timing issues.
-
Check Locator Strategies: Always validate your XPath or CSS Selector. Tools like Chrome's DevTools can facilitate this by enabling you to experiment with selectors.
-
Debugging: Use breakpoints or logging to identify the exact point of failure. Debugging tools can also allow you to step through the test line-by-line.
-
Element Visibility: Verify that the element is interactable (i.e., it is not hidden behind an overlay). You may need to scroll into view or ensure it is not obstructed.
-
Re-fetch Elements: If the page refreshes or changes dynamically, be proactive in re-fetching elements as needed. This helps avoid stale references.
-
Utilize Page Object Model: Implement the Page Object Model (POM) design pattern. This approach encapsulates the logic of the page interactions and provides a cleaner framework for managing tests, which can lead to fewer errors.
For more information about best practices when using Selenium, you can check out the Selenium official documentation.
Closing Remarks
Selenium is a robust tool for UI testing, but it's essential to understand and troubleshoot the common failures that can arise during test execution. By mastering the techniques discussed here, you can improve your Selenium tests and ensure reliable execution. Embracing best practices such as explicit waits, validating locators, and utilizing the Page Object Model can drastically enhance your testing efficiency.
With ongoing updates and improvements in web technologies, staying informed on troubleshooting techniques and adapting to changes will empower you to effectively manage Selenium UI tests. Happy testing!