Unlocking Primary Annotations: Usage Challenges in Spring
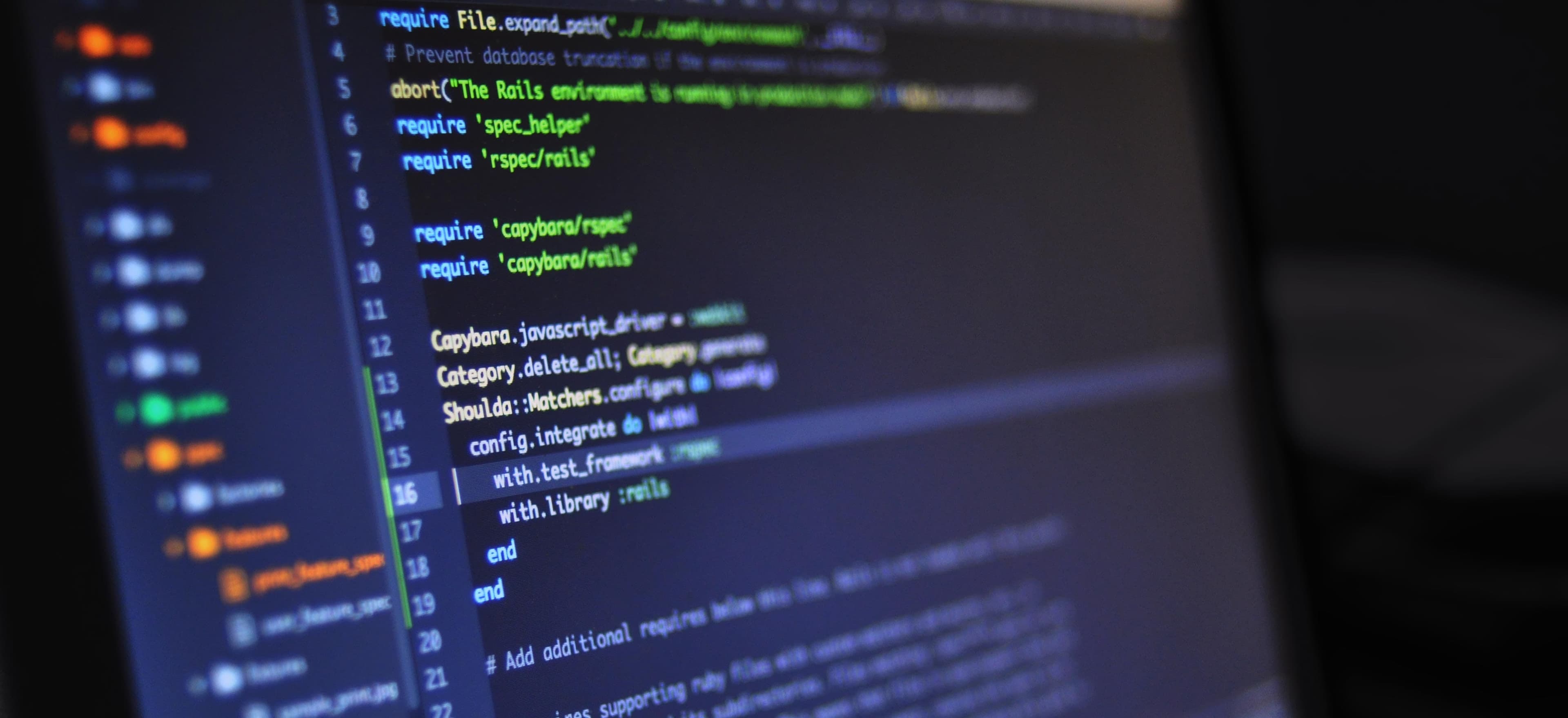
- Published on
Unlocking Primary Annotations: Usage Challenges in Spring
Spring Framework is known for its powerful features and annotations that simplify Java application development. However, with great power comes great responsibility, and understanding how to effectively use annotations, particularly primary annotations, can be a bit challenging. In this blog post, we’ll dive into the intricacies of using primary annotations in Spring, their implications, and some common pitfalls to avoid.
What Are Primary Annotations in Spring?
In Spring, annotations are used to define application behavior and manage dependencies. Primary annotations refer to those specifically meant to indicate a primary bean for dependency injection when multiple candidates are available.
The most commonly used primary annotation in Spring is @Primary
. When you mark a bean with this annotation, it tells the Spring container that this bean should be given preference when multiple beans of the same type exist.
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Primary;
@Configuration
public class AppConfig {
@Bean
public MyBean myBeanOne() {
return new MyBean("Bean One");
}
@Bean
@Primary
public MyBean myBeanTwo() {
return new MyBean("Bean Two");
}
}
The Importance of Annotations
Annotations play an essential role in Java, especially in frameworks like Spring. They provide metadata that helps in the configuration of components in a clear and concise manner. This is particularly important for managing Dependency Injection (DI), where objects need to be injected into other objects.
Common Usage Challenges with @Primary
While the @Primary
annotation simplifies certain aspects of dependency management, it can introduce challenges if not used judiciously. Below are some common issues developers face:
1. Ambiguous Dependency Resolution
When multiple beans of the same type exist, Spring needs a way to determine which one to inject. If you do not mark one of the beans as @Primary
, the application may throw an error indicating ambiguity in the dependency.
@Autowired
private MyBean myBean; // This will cause an error if not marked with @Primary
To avoid this, ensure that you honor the @Primary
annotation. However, using @Primary
indiscriminately can create opaque dependencies that are hard to trace.
2. Testing Issues
Testing is often affected by how beans are configured. For example, if you have a primary bean used in your production code, it may complicate unit tests where distinct behavior is desired. Using mocks can resolve this, but it leads to increased complexity in test setups.
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = TestConfig.class)
public class MyServiceTest {
@MockBean
private MyBean myBean; // This can lead to inconsistencies
}
3. Overriding @Primary Annotations
In some scenarios, it may be tempting to override a primary bean in a child context or through profiles. This can lead to confusion regarding which bean is currently active, especially in larger applications. Maintaining clarity in bean definitions is crucial.
Best Practices for Using @Primary
Despite the challenges, following best practices can make @Primary
a powerful tool:
1. Use Wisely
Only mark beans as @Primary
when it’s clear that they are intended to be the default. Avoid marking multiple beans as primary unless absolutely necessary.
2. Clearly Document
When using @Primary
, make sure to document your intent within the code. Comments can help other developers understand why this choice was made, preventing confusion later on.
3. Design for Testing
When designing your classes, keep testing in mind. You can use interfaces and avoid concrete classes as much as possible. This will allow you to replace dependencies easily during tests.
public interface MyBean {
String getName();
}
@Primary
@Bean
public MyBean myBeanTwo() {
return new MyBeanImpl("Bean Two");
}
Alternatives to @Primary
While @Primary
is useful, it’s not the only tool in your toolbox. Alternatives exist that can also resolve dependency ambiguity.
1. @Qualifier Annotation
The @Qualifier
annotation can be used in conjunction with @Autowired
to specify exactly which bean to inject. For instance, if you have a bean without the @Primary
annotation, you can specify it directly.
@Autowired
@Qualifier("myBeanOne")
private MyBean myBean;
2. Profiles
Using profiles can allow you to create separate configurations for development, testing, and production. This way, you can have primary beans for each environment as needed.
@Profile("dev")
@Bean
public MyBean devBean() {
return new MyBean("Development Bean");
}
A Final Look
Mastering the use of primary annotations in Spring can significantly enhance your application architecture. While @Primary
offers a straightforward way to manage dependency resolution, it requires careful consideration and documentation to avoid common pitfalls.
Being aware of the challenges—ambiguous dependency resolution, testing complexities, and potential overriding of primary annotations—enables developers to think critically about their design choices. Furthermore, leveraging alternatives like @Qualifier
and profiles can provide more flexibility and clarity to your Spring applications.
To deepen your understanding of dependency injection in Spring, consider checking out the Spring Documentation and Spring Guides on Dependency Injection. They offer valuable insights and extend your knowledge even further.
By balancing the use of @Primary
against potential complications, developers can unlock the full potential of Spring frameworks in their applications. Happy coding!