Troubleshooting Common Issues in Angular-Node-Mongo Apps
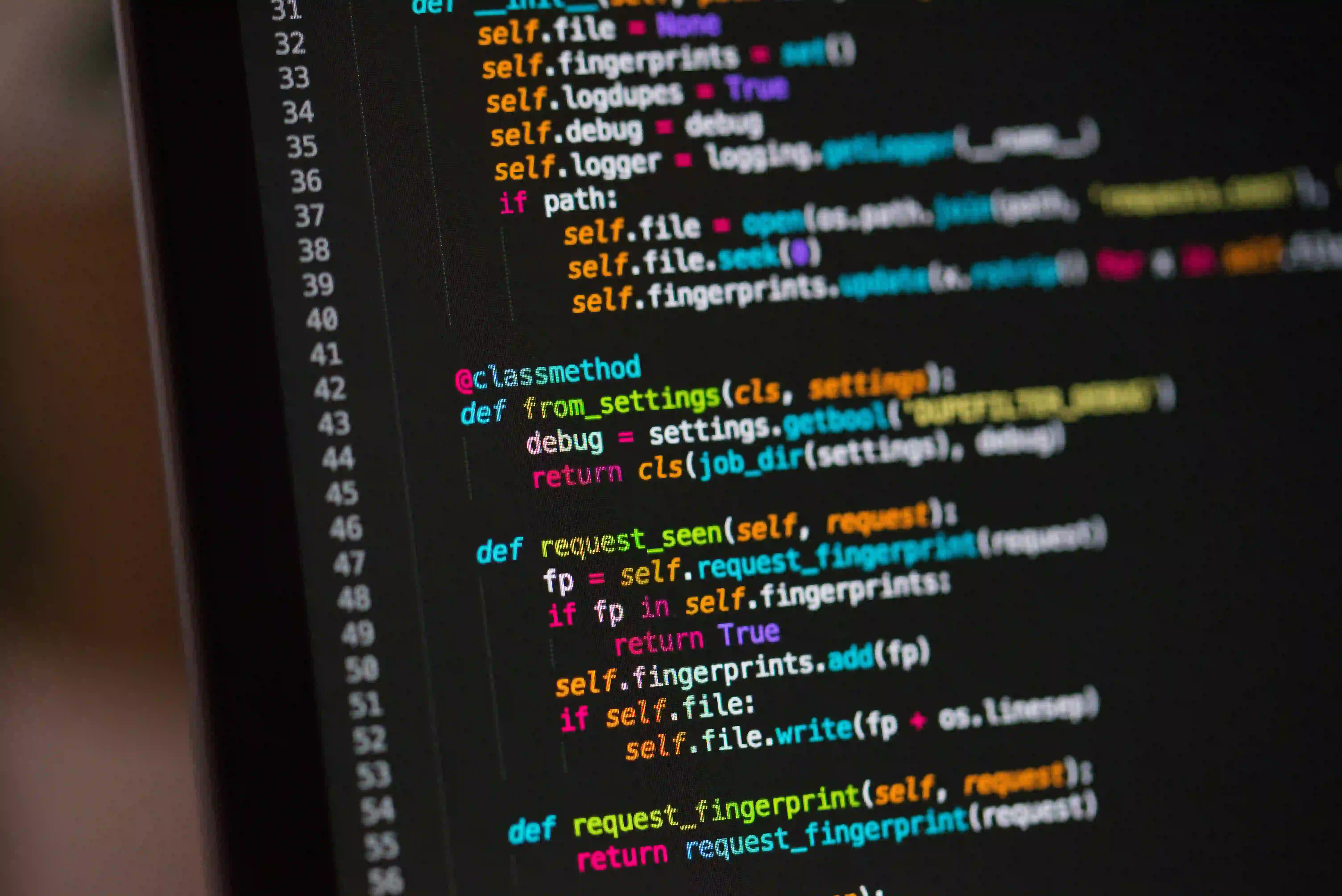
Troubleshooting Common Issues in Angular-Node-Mongo Apps
Building full-stack applications using Angular, Node.js, and MongoDB is a common approach many developers use today. However, just like any other technology stack, challenges abound. This blog post addresses common issues you may encounter and provides guidance on how to troubleshoot them effectively.
Table of Contents
- Introduction
- Common Issues
- Issue 1: API Requests Not Responding
- Issue 2: CORS Errors
- Issue 3: MongoDB Connection Problems
- Issue 4: Angular Routing Errors
- Best Practices for Troubleshooting
- Conclusion
Setting the Stage
The MERN stack (MongoDB, Express, React, Node.js) and MEAN stack (MongoDB, Express, Angular, Node.js) are popular in modern web application development. Both allow developers to build powerful client-server applications efficiently. However, issues may arise that can halt development. Here, we will cover frequent challenges faced, focusing on resolution techniques.
Common Issues
Issue 1: API Requests Not Responding
When your Angular application makes a request to the Node.js server and gets no response or an error, it can be very frustrating. This issue often stems from several factors.
Possible Causes:
- The backend server is not running.
- Incorrect endpoint URL.
- The API service is experiencing downtime.
Troubleshooting Steps:
- Check Server Status: Ensure your Node.js server is running. You can check this by accessing the default route in your browser or using a tool like Postman.
node server.js
Ensure that your server's terminal indicates it is listening on the correct port.
- Verify Endpoint URL: Make sure the URL you are hitting in your API request matches the route defined in your server.
// Example of an Angular service
getData(): Observable<Data[]> {
return this.http.get<Data[]>('http://localhost:3000/api/data');
}
If this URL is incorrect, your request will not reach the server.
Issue 2: CORS Errors
Cross-Origin Resource Sharing (CORS) issues can arise when the front end and back end of your application are hosted on different domains or ports.
Common Symptoms:
- Browser console errors indicate that the request has been blocked due to CORS policy.
Troubleshooting Steps:
- Enable CORS in Node.js Server: Use the
cors
package in your Node.js application to allow requests from different origins.
const cors = require('cors');
app.use(cors());
This will allow your API to respond to requests from your Angular application.
- Whitelist Specific Origins: For enhanced security, you can explicitly define which origins can access your API.
app.use(cors({ origin: 'http://localhost:4200' }));
Issue 3: MongoDB Connection Problems
Often, connection issues with MongoDB can arise from network problems or incorrect URL configurations.
Possible Causes:
- Incorrect MongoDB connection string.
- MongoDB server not running.
Troubleshooting Steps:
- Check Connection String: Verify your connection string in your Node.js application.
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/myDatabase', { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('MongoDB connected'))
.catch(err => console.log(err));
Any syntax errors or an incorrect URL can prevent MongoDB from connecting.
- Check MongoDB Status: Use a command line to check if your MongoDB database is up.
sudo service mongod status
This command will let you know if the MongoDB service is active.
Issue 4: Angular Routing Errors
Angular’s router can sometimes lead to unexpected behavior, such as 404 errors when navigating between views.
Common Symptoms:
- The application displays a blank page instead of the expected view.
Troubleshooting Steps:
- Check Routes Configuration: Double-check the Angular routing setup.
const routes: Routes = [
{ path: 'home', component: HomeComponent },
{ path: 'about', component: AboutComponent },
{ path: '', redirectTo: '/home', pathMatch: 'full' },
];
Using the appropriate path names is crucial for smooth navigation.
- RouterModule Configuration: Make sure
RouterModule
imports are correctly added inAppModule
.
@NgModule({
imports: [RouterModule.forRoot(routes)],
})
export class AppModule {}
Best Practices for Troubleshooting
- Use Logging Liberally: Add logging to both the backend and frontend to monitor the flow of data and control.
- Isolate the Source of Problems: Use Postman to simulate API requests independently from Angular. It helps ensure that your Node.js backend is functioning correctly.
- Stay Updated: Use the latest versions of your libraries and frameworks. This minimizes bugs that may have already been fixed in newer releases.
Useful Links:
Closing the Chapter
Troubleshooting is an integral part of software development, especially in full-stack applications like Angular-Node-Mongo setups. By systematically addressing common issues and employing effective debugging techniques, you can rapidly resolve most challenges you face. Keeping logs, isolating problems, and validating configuration settings will lead to smoother workflows and increased productivity. Always remember, while issues are inevitable in development, they are also opportunities for learning and honing your skills.
By following the structured approach outlined in this article, you should feel more equipped to tackle issues in your applications as they arise. Happy coding!