Troubleshooting JVM Monitoring: Common Pitfalls and Fixes
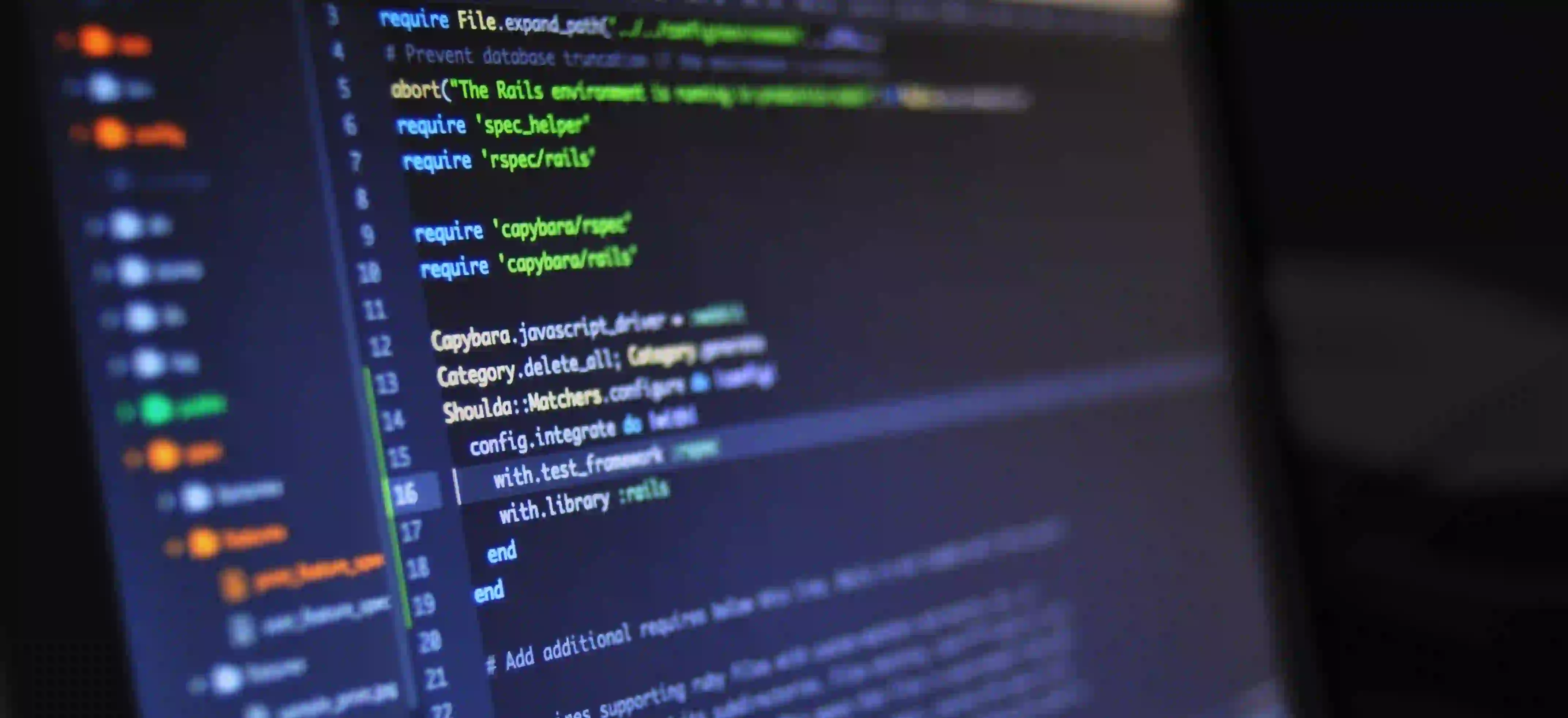
Troubleshooting JVM Monitoring: Common Pitfalls and Fixes
Java Virtual Machine (JVM) monitoring is a crucial aspect of maintaining performance and stability in Java applications. As Java developers, we often face challenges when it comes to diagnosing issues at the JVM level. Whether you're grappling with memory leaks, performance bottlenecks, or unexpected crashes, effective monitoring is essential. This post delves into common pitfalls in JVM monitoring, exploring effective strategies and how to resolve them.
Understanding JVM Monitoring
Before diving into troubleshooting, it's vital to understand what JVM monitoring entails. At its core, JVM monitoring involves tracking the state and performance of your Java applications by observing metrics such as:
- Memory usage
- Garbage collection (GC) behavior
- Thread activity
- CPU utilization
Monitoring these metrics allows developers to identify performance issues and optimize applications accordingly.
Key JVM Monitoring Tools
- Java Management Extensions (JMX)
- VisualVM
- Java Mission Control (JMC)
- Prometheus and Grafana
- New Relic
Each tool comes with its own strengths and weaknesses, and the choice depends on specific needs.
Common Pitfalls in JVM Monitoring
1. Overlooking Memory Leaks
Why It’s a Pitfall
Memory leaks occur when objects are no longer needed but are still referenced, preventing the garbage collector from reclaiming memory. This can lead to OutOfMemoryErrors.
Fix
Utilize profiling tools to analyze memory usage. VisualVM, for example, allows you to monitor heap dumps and analyze memory consumption over time.
Code Example: Taking a Heap Dump with JVisualVM
// Use the following command to start Java VisualVM
// Ensure that JVisualVM is installed with the JDK
jvisualvm
To take a heap dump from VisualVM, simply right-click your application instance, select 'Heap Dump', and analyze the results. Look for long-lived objects that should have been collected.
2. Ignoring Garbage Collection Metrics
Why It’s a Pitfall
Garbage collection is a crucial mechanism for managing memory in JVM. Many developers overlook the GC logs, leading to increased pause times and application slowdowns.
Fix
Enable GC logging to analyze its performance. By doing so, you can determine if GC is running too frequently or excessively, causing application lag.
Code Example: Enabling GC Logging
java -Xlog:gc*:file=gc.log:time -jar your_application.jar
This command creates a GC log file (gc.log
) that records every GC activity, including time taken and memory reclaimed. Analyzing this log is essential to understanding how often and how effectively GC operates.
3. Misconfigured JVM Options
Why It’s a Pitfall
Incorrect JVM configurations can lead to performance degradation. For instance, insufficient heap size can lead to frequent GC cycles, while oversized heap sizes may lead to long GC times.
Fix
Review and adjust the JVM parameters based on the application load. The following parameters control heap size:
-Xms
: Sets the initial heap size.-Xmx
: Sets the maximum heap size.
Code Example: Setting Heap Sizes
java -Xms512m -Xmx4g -jar your_application.jar
In this command, the JVM starts with 512 MB of memory and can grow up to 4 GB as needed, optimizing memory use without overwhelming the system.
4. Failing to Monitor Thread Behavior
Why It’s a Pitfall
Threads within a Java application can become blocked or deadlocked, adversely affecting performance. Not monitoring thread states can lead to undetected issues.
Fix
Use tools like VisualVM or JMC to analyze thread states in real-time. Look for threads that are in the BLOCKED or WAITING state, as they might indicate underlying issues.
Code Example: Analyzing Thread States
// Call this method to examine thread state at runtime
Thread.getAllStackTraces().forEach((thread, stackTrace) -> {
System.out.println(thread.getName() + ": " + thread.getState());
});
This snippet provides a snapshot of the current thread states. Regularly monitoring thread activity helps pinpoint where bottlenecks may occur.
5. Ignoring Application Logs
Why It’s a Pitfall
Application logs provide invaluable insights into application behavior, yet many overlook them during monitoring.
Fix
Integrate logging libraries such as SLF4J or Log4j. Ensure that logs are detailed, capturing both errors and warnings, which will illuminate issues not visible through metrics alone.
Code Example: Basic Logging with SLF4J
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class Example {
private static final Logger logger = LoggerFactory.getLogger(Example.class);
public void performAction() {
logger.info("Action started");
// Perform action
logger.error("An error occurred");
}
}
With this implementation, logs will help trace the application flow and highlight errors as they occur. A well-structured logging strategy is essential for effective troubleshooting.
Best Practices for JVM Monitoring
- Establish Baselines: Know how your application performs under normal conditions. Document key metrics for future comparisons.
- Automate Monitoring: Use automated tools to continuously gather performance metrics and alert on significant deviations.
- Regularly Review Configuration: Reassess your JVM settings based on changing application loads and usage patterns.
- Combine Metrics with Logs: Leverage both metrics and logs for a comprehensive view of application health.
- Keep Learning: Stay updated with JVM improvements and updates that may impact performance.
In Conclusion, Here is What Matters
Monitoring the JVM is not just about keeping an eye on metrics; it requires a holistic approach that encompasses a variety of tools, techniques, and practices. Understanding these common pitfalls and how to resolve them lays the groundwork for creating stable, high-performing Java applications.
By implementing the practices discussed, and taking advantage of monitoring tools and techniques, you will be well-equipped to troubleshoot JVM issues efficiently. The next time you face unexpected behavior in your application, you can dive into the world of JVM monitoring with confidence.
Further Reading
- Java Documentation on JMX
- Oracle's Java Garbage Collection Tuning Guide
- Using VisualVM for Memory Profiling
With ongoing diligence in JVM monitoring, you can ensure your applications run smoothly at their peak performance. Happy coding!