Mastering ISO 8601 Durations in Java: Common Pitfalls
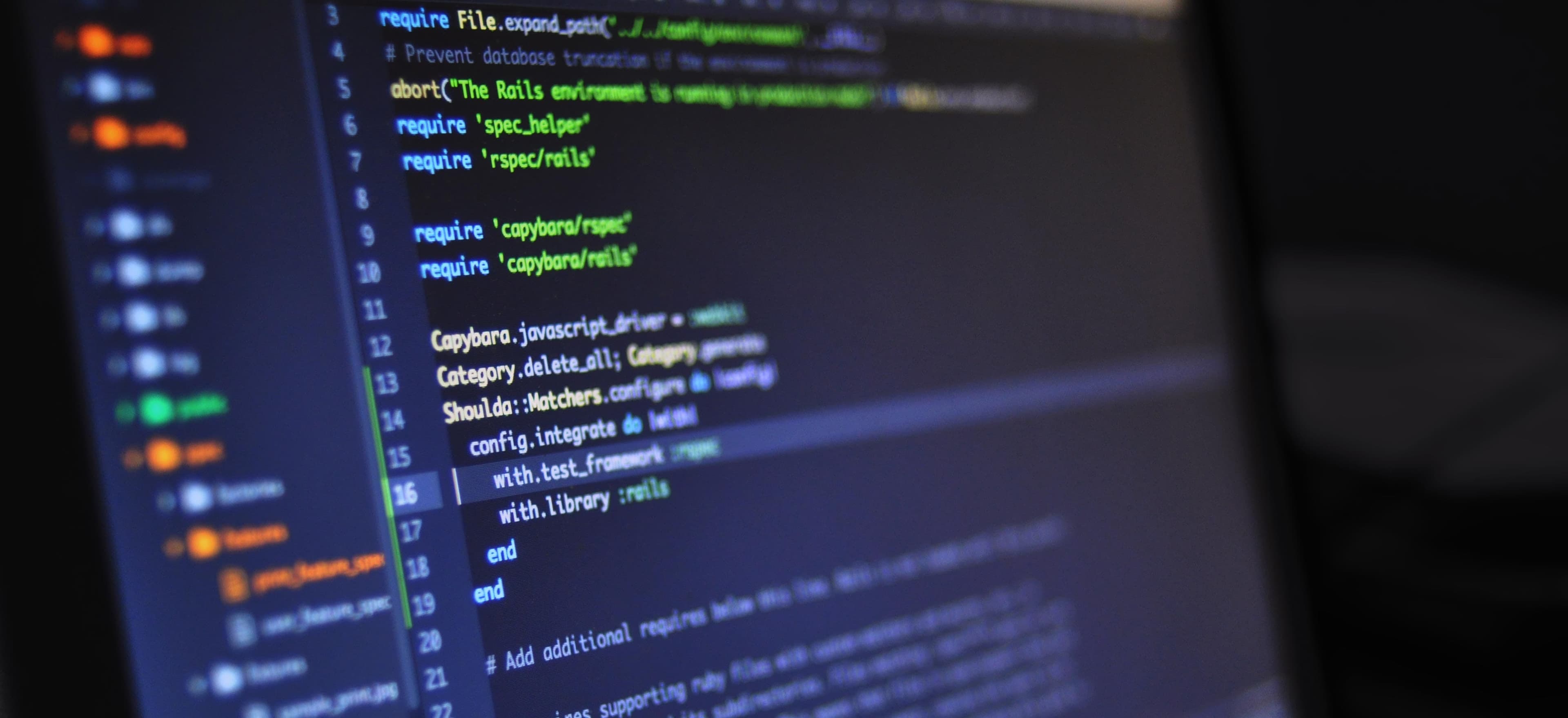
- Published on
Mastering ISO 8601 Durations in Java: Common Pitfalls
In an increasingly connected world, working with date and time is essential. ISO 8601 has become a universally accepted format for representing dates and durations. Java provides robust tools for handling these formats, but mastering them is often fraught with common pitfalls. In this blog post, we'll explore ISO 8601 durations, identify the frequent mistakes that developers make, and offer guidance to sidestep these issues.
What is ISO 8601?
ISO 8601 is an international standard for date and time notation. Specifically, it defines a consistent format for representing dates, times, and durations. Durations are represented as a time span, using a format such as:
P[n]Y[n]M[n]DT[n]H[n]M[n]S
Where:
- P indicates the period
- Y stands for years
- M stands for months
- D stands for days
- T is the time designator
- H for hours
- M for minutes
- S for seconds
For example, a duration of "P2Y3DT4H5M6S" translates to "2 years, 3 days, 4 hours, 5 minutes, and 6 seconds."
Understanding this structure is crucial for successful implementation in Java.
Getting Started with Java's Date-Time API
Java 8 introduced a new Date-Time API located in the java.time
package. This API includes classes such as Duration
and Period
for manipulating durations and periods respectively.
To parse and format ISO 8601 durations, you can use the following code snippet:
import java.time.Duration;
import java.time.Period;
import java.time.format.DateTimeParseException;
public class Iso8601Examples {
public static void main(String[] args) {
String isoDuration = "PT20H15M30S"; // 20 hours, 15 minutes, 30 seconds
String isoPeriod = "P2Y3DT4H5M6S"; // 2 years, 3 days, 4 hours, 5 minutes, 6 seconds
try {
Duration duration = Duration.parse(isoDuration);
Period period = Period.parse(isoPeriod);
System.out.println("Parsed Duration: " + duration);
System.out.println("Parsed Period: " + period);
} catch (DateTimeParseException e) {
System.err.println("Invalid format: " + e.getMessage());
}
}
}
Explanation of the Code
- Imports: The required classes are imported for handling
Duration
,Period
, and parsing exceptions. - Parsing:
Duration.parse
andPeriod.parse
are used to convert ISO strings directly into Java objects. This method is strict and will throw aDateTimeParseException
if the format doesn't match. - Error Handling: A try-catch block ensures that any invalid input is appropriately handled, informing the user of the error.
This example provides a foundational understanding of parsing durations and periods. However, pitfalls can arise when calculating or comparing these objects.
Common Pitfalls with ISO 8601 Durations in Java
1. Misusing Duration and Period
Duration
is designed to represent time-based amounts, while Period
represents date-based amounts. This distinction matters. Attempting to use a Duration
when a Period
is needed will yield misleading results.
Example of the Mistake
import java.time.Duration;
import java.time.Period;
public class TypeMistake {
public static void main(String[] args) {
// Assuming you want to represent a period of six months
Duration durationMistake = Duration.ofMonths(6); // Mistake, durations cannot represent months
// Correct usage
Period periodCorrect = Period.ofMonths(6);
System.out.println("Duration Mistake: " + durationMistake); // Gives an error
System.out.println("Period Correct: " + periodCorrect);
}
}
2. Ignoring Negative Durations
In certain business applications, negative durations can trigger unexpected behaviors. For instance, subtracting a longer duration from a shorter one might yield an illogical outcome if not handled correctly.
Key Consideration
Always check if a duration is negative and handle it accordingly, potentially using validations:
import java.time.Duration;
public class NegativeDurationCheck {
public static void main(String[] args) {
Duration duration1 = Duration.ofHours(4);
Duration duration2 = Duration.ofHours(5);
Duration result = duration1.minus(duration2);
if (result.isNegative()) {
System.err.println("The result of the duration is negative!");
} else {
System.out.println("Resulting Duration: " + result);
}
}
}
3. Mixing Time Units Inappropriately
Developers often misinterpret the relationship between different time units, leading to incorrect calculations. Remember that Duration
operates in terms of seconds and nanoseconds, while Period
deals with days, months, and years.
Example of Mixing Units
Consider an attempt to add months and seconds together without careful consideration of their measurement conversion.
Instead, always convert to a common unit when necessary:
import java.time.Duration;
import java.time.Period;
public class MixingUnits {
public static void main(String[] args) {
Period period = Period.ofDays(10);
Duration duration = Duration.ofHours(24); // Duration represents 1 day
// This is a mistake: you can't add these directly.
// Duration total = period.addTo(duration); // Would cause errors
// Correct: Convert Period to Duration
long totalHours = duration.toHours() + period.toTotalMonths() * 30 * 24; // Simple conversion (not accurate)
System.out.println("Total Hours (Approximated): " + totalHours);
}
}
4. Not Considering Time Zones
When calculating specific durations with respect to time zones, also account for the potential variability due to Daylight Saving Time. Using ZonedDateTime
will help alleviate these issues.
Example of Time Zone Consideration
import java.time.ZonedDateTime;
import java.time.Duration;
public class TimeZoneConsideration {
public static void main(String[] args) {
ZonedDateTime startDate = ZonedDateTime.now();
ZonedDateTime endDate = startDate.plus(Duration.ofHours(5)); // Adding 5 hours
System.out.println("Start Date: " + startDate);
System.out.println("End Date: " + endDate);
// Check if there was a time zone change (e.g., Daylight saving)
if (!startDate.getZone().equals(endDate.getZone())) {
System.out.println("The time zones differ.");
}
}
}
Key Takeaways
Understanding ISO 8601 durations in Java requires more than just parsing strings. Missteps can lead to confusion and errors in your applications. By recognizing and avoiding these common pitfalls—such as misusing Duration
and Period
, ignoring negative durations, and mixing time units—you can master date and time manipulations effectively.
For more in-depth information, consider exploring the Java Date and Time documentation.
Mastering these elements will not only improve your Java programming skills but also enhance the performance and reliability of your applications. The world of date and time is complex, but with careful attention, you can navigate it successfully.
Checkout our other articles