Top 5 Mistakes in Securing User Authentication for Apps
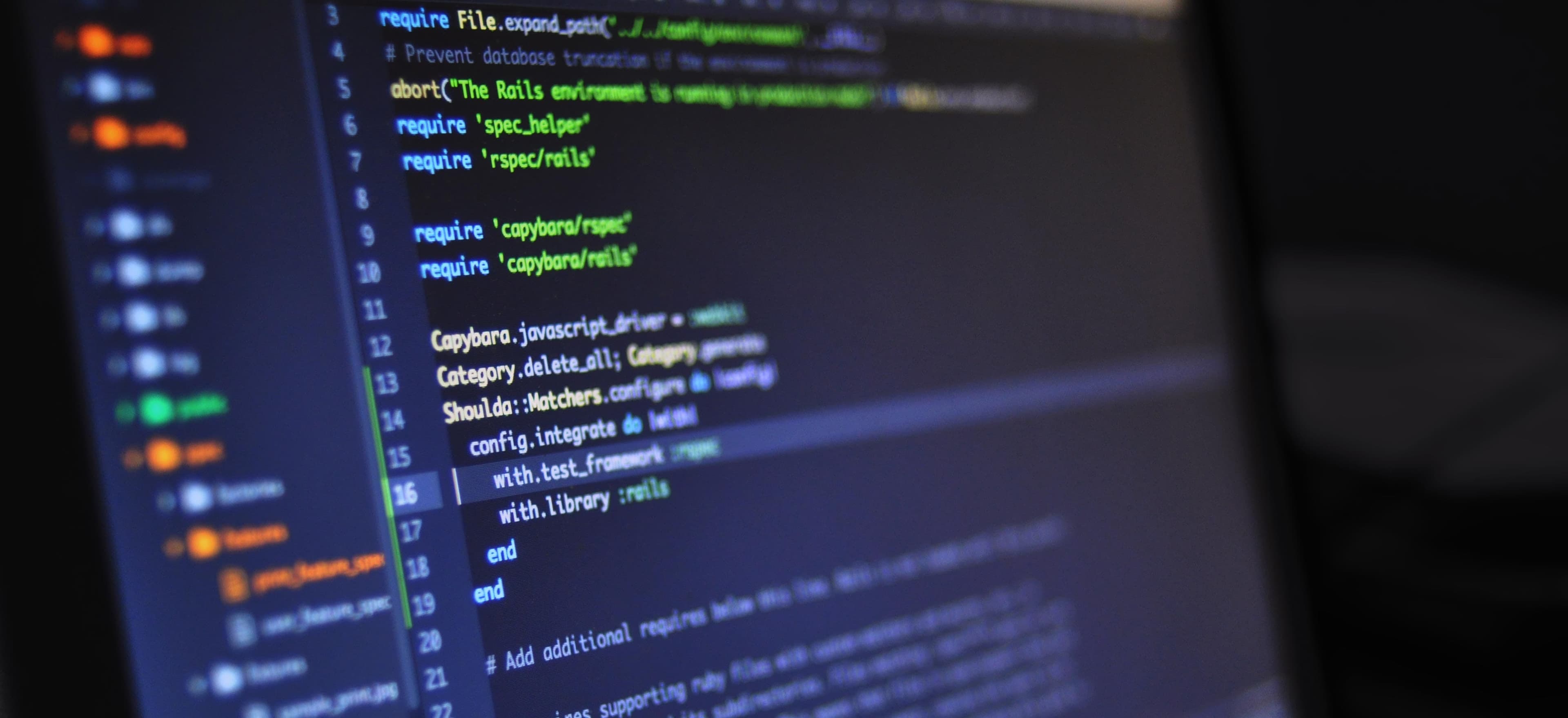
- Published on
Top 5 Mistakes in Securing User Authentication for Apps
In today’s digital landscape, securing user authentication is paramount. As cyber threats evolve, so must our approaches to software security—especially in applications where user identity verification is critical. In this blog post, we will explore the top five mistakes developers often make regarding user authentication and how to prevent them.
1. Weak Password Policies
Mistake:
Many applications allow users to create weak passwords or avoid strong password enforcement. This creates significant vulnerabilities, as attackers can easily guess or brute force these passwords.
Why It Matters:
Weak passwords are often the first line of defense that hackers target. A strong password policy is essential in minimizing the risk of unauthorized access.
Solution:
Implement a strong password policy that requires a mix of uppercase letters, lowercase letters, numbers, and special characters. Consider the following Java code snippet that validates password complexity:
import java.util.regex.Pattern;
public class PasswordValidator {
private static final String PASSWORD_PATTERN =
"^(?=.*[0-9])(?=.*[a-z])(?=.*[A-Z])(?=.*[@#$%^&+=])(?=\\S+$).{8,}$";
public static boolean isValidPassword(String password) {
return Pattern.compile(PASSWORD_PATTERN).matcher(password).matches();
}
}
Commentary:
This regex pattern ensures that passwords contain at least one numeric digit, one lowercase letter, one uppercase letter, and one special character, all while being at least eight characters long. Such rules help reinforce user accounts against unauthorized access.
2. Lack of Two-Factor Authentication (2FA)
Mistake:
Failing to implement two-factor authentication (2FA) creates a significant vulnerability. Relying solely on passwords means that any user credential compromise can lead to account takeover.
Why It Matters:
2FA adds an extra layer of security by requiring additional verification beyond just a password. This significantly reduces the chances of unauthorized access even if user credentials are compromised.
Solution:
Integrate 2FA using one-time codes or authentication apps. Here’s a sample code snippet to demonstrate how you might manage the generation and verification of a one-time code:
import java.util.concurrent.ThreadLocalRandom;
public class TwoFactorAuth {
public static int generateCode() {
return ThreadLocalRandom.current().nextInt(100000, 999999);
}
public static boolean verifyCode(int providedCode, int actualCode) {
return providedCode == actualCode;
}
}
Commentary:
In this code, we generate a random six-digit code for 2FA and verify it. Encourage users to use applications like Google Authenticator, which can enhance their authentication processes.
3. Storing Passwords Insecurely
Mistake:
Storing user passwords in plain text or using weak hashing algorithms like MD5 or SHA-1 is a severe error. If a database breaches occur, passwords can be easily retrieved.
Why It Matters:
These practices expose users to grave risks, as compromised passwords can lead to account hacking and personal data theft.
Solution:
Always hash passwords using strong hashing algorithms like bcrypt, Argon2, or PBKDF2, which include salting and iteration techniques. Here’s an example of using bcrypt in Java:
import org.mindrot.jbcrypt.BCrypt;
public class PasswordUtil {
public static String hashPassword(String password) {
return BCrypt.hashpw(password, BCrypt.gensalt());
}
public static boolean checkPassword(String password, String hashed) {
return BCrypt.checkpw(password, hashed);
}
}
Commentary:
Using bcrypt enhances security by making it difficult for hackers to reverse-engineer hashed passwords. The gensalt()
function generates a random salt for the password hash, adding another layer of security.
4. Not Implementing Account Lockout Mechanisms
Mistake:
A lack of account lockout mechanisms can lead to repeated attempts at guessing passwords, thereby increasing vulnerability.
Why It Matters:
Without restrictions, an attacker could brute-force an account indefinitely, gaining access eventually.
Solution:
Implement an account lockout policy after a specific number of failed login attempts. Below is an example of how you can manage account lockouts:
import java.util.HashMap;
import java.util.Map;
public class AccountLockout {
private static final int MAX_ATTEMPTS = 5;
private Map<String, Integer> loginAttempts = new HashMap<>();
public boolean login(String username, String password) {
if (isAccountLocked(username)) {
return false; // Account is locked
}
// Authentication logic here
boolean success = authenticate(username, password);
if (!success) {
loginAttempts.put(username, loginAttempts.getOrDefault(username, 0) + 1);
if (loginAttempts.get(username) >= MAX_ATTEMPTS) {
lockAccount(username);
}
}
return success;
}
private boolean isAccountLocked(String username) {
// Check if account is locked
return false; // Implement actual locking logic here
}
private void lockAccount(String username) {
// Logic to lock the account
}
}
Commentary:
This example tracks login attempts and locks the account after five failed attempts. Lockouts can drastically reduce brute-force attacks on user accounts.
5. Lack of Security Testing and Monitoring
Mistake:
Many developers avoid ongoing security testing, believing that once the app is launched, security is sufficient. However, the reality is quite different.
Why It Matters:
Applications are continually exposed to new vulnerabilities, and failure to monitor and test for these can lead to serious compromises.
Solution:
Incorporate regular security audits, penetration testing, and logging features to monitor suspicious activities. Consider using tools like OWASP ZAP or Snyk to help in ongoing security assessments.
Best Practices:
- Use session management frameworks: Secure session handling is critical. Implement secure cookies and session timeouts.
- Log security events: Monitor and log authentication attempts, especially failed login attempts.
- Educate users on security: Encourage users to adopt security best practices, such as recognizing phishing attempts and safeguarding their credentials.
Final Considerations
Securing user authentication is not just about implementing features; it is a continuous process involving vigilance, education, and adaptation to evolving threats. By avoiding these five common mistakes, developers can significantly improve the security posture of their applications.
For more in-depth knowledge on application security, check out OWASP's Top Ten project. Prioritizing user authentication ultimately leads to trust, user retention, and a secure application environment—elements essential for any successful digital product.
With this guide, you should be better prepared to identify and rectify authentication vulnerabilities in your applications. Stay updated on security practices and crucial code implementations to keep your software—and your users—secure!