Choosing the Right Framework for Mocking Static Methods
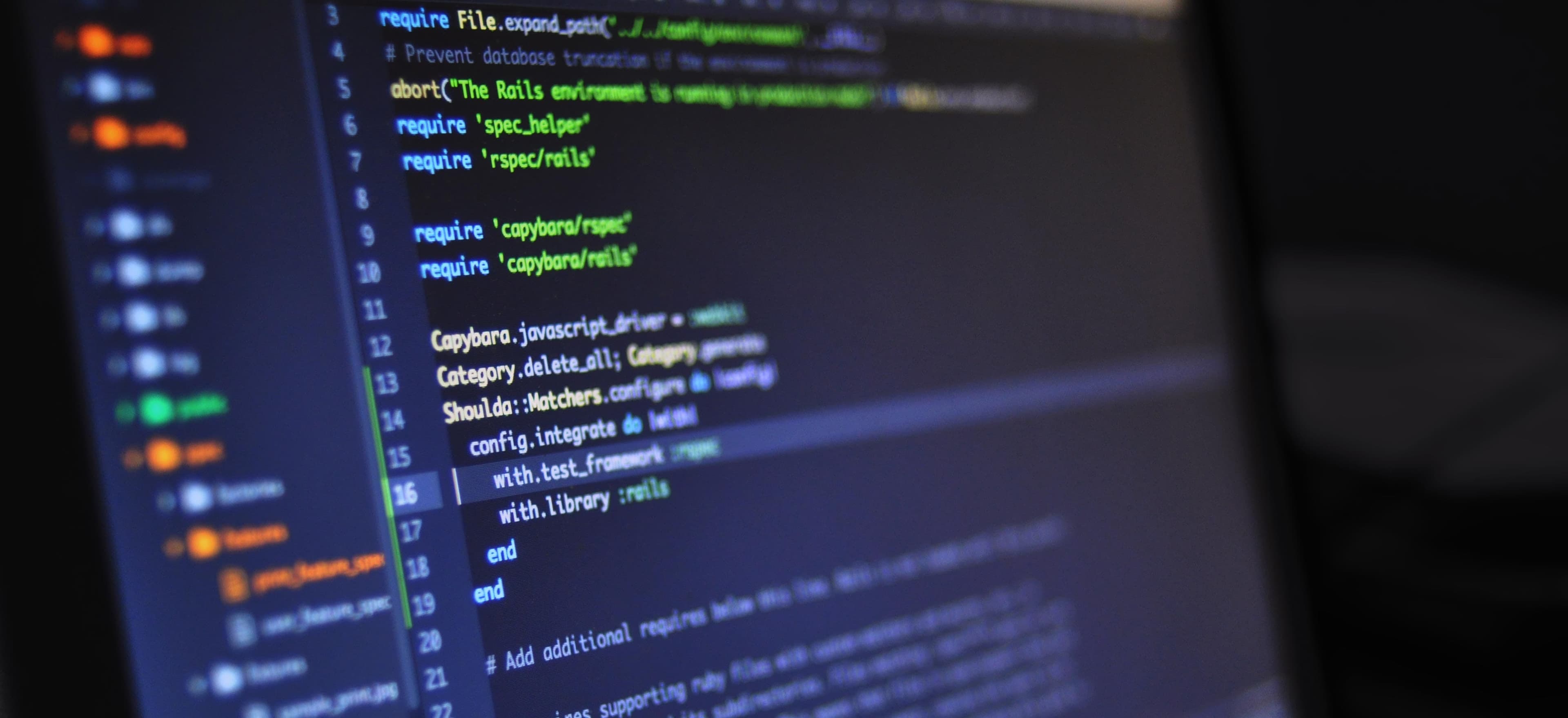
- Published on
Choosing the Right Framework for Mocking Static Methods
Static methods are often a point of contention in software design and testing. Their inherent nature can introduce challenges when it comes to unit testing. However, with the right frameworks and strategies, we can effectively handle them. In this article, we will delve into various options for mocking static methods in Java, discussing their pros and cons, and guiding you through code examples to illustrate their usage.
Understanding the Challenge
Static methods belong to the class rather than an instance of the class, making them difficult to mock. Traditional mocking frameworks like Mockito were initially built for mocking instance methods, which can lead to limitations.
Why Mock Static Methods?
Mocking static methods can help in various scenarios, particularly when:
- Third-party APIs: When interacting with external services that are not under your control.
- Legacy Code: When dealing with older libraries or code you can't modify.
- Isolation: Ensuring that tests run independently from other areas of the code.
Common Frameworks for Mocking Static Methods
When it comes to mocking static methods in Java, several frameworks are available. Below, we will look at the most popular options:
1. PowerMock
PowerMock is a powerful framework that extends the functionality of existing mock frameworks like Mockito or EasyMock. It allows you to mock static methods and even constructors.
Setting Up PowerMock
To get started with PowerMock, include the following dependencies in your pom.xml
:
<dependency>
<groupId>org.powermock</groupId>
<artifactId>powermock-module-junit4</artifactId>
<version>2.0.9</version> <!-- or latest -->
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.powermock</groupId>
<artifactId>powermock-api-mockito2</artifactId>
<version>2.0.9</version> <!-- or latest -->
<scope>test</scope>
</dependency>
Code Example with PowerMock
Here’s how to mock a static method using PowerMock:
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.MockedStatic;
import org.mockito.Mockito;
import org.powermock.api.mockito.PowerMockito;
import org.powermock.modules.junit4.PowerMockRunner;
@RunWith(PowerMockRunner.class)
public class StaticMethodTest {
@Test
public void testStaticMethod() {
// Arrange
MockedStatic<StaticClass> mockedStatic = PowerMockito.mockStatic(StaticClass.class);
mockedStatic.when(StaticClass::staticMethod).thenReturn("Mocked Result");
// Act
String result = StaticClass.staticMethod();
// Assert
assertEquals("Mocked Result", result);
// Verify
mockedStatic.verify(StaticClass::staticMethod);
mockedStatic.close(); // Important to close the static mock
}
}
Why Use PowerMock?
PowerMock is particularly beneficial when you are dealing with legacy code or third-party libraries that you cannot modify. It comes with a heavier footprint compared to other mock frameworks but gives you flexibility when needed.
2. Mockito with Inline Mocking
Starting from Mockito 3.4.0, support for mocking static methods has been added directly. This is accomplished through an inline mocking extension, allowing developers to mock static methods without needing PowerMock.
Setting Up Mockito
To use Mockito's inline mocking capability, add the necessary dependency:
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-inline</artifactId>
<version>4.0.0</version> <!-- or latest -->
<scope>test</scope>
</dependency>
Code Example with Mockito
Here’s how to mock a static method using Mockito:
import org.junit.jupiter.api.Test;
import org.mockito.MockedStatic;
import org.mockito.Mockito;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class StaticMethodMockingTest {
@Test
public void testStaticMethod() {
try (MockedStatic<StaticClass> mockedStatic = Mockito.mockStatic(StaticClass.class)) {
// Arrange
mockedStatic.when(StaticClass::staticMethod).thenReturn("Mocked Result");
// Act
String result = StaticClass.staticMethod();
// Assert
assertEquals("Mocked Result", result);
// Verify
mockedStatic.verify(StaticClass::staticMethod);
}
}
}
Why Use Mockito for Static Methods?
Mockito's inline mocking is simpler for those who already use Mockito and want to avoid additional dependencies. It integrates smoothly and maintains the familiarity of Mockito’s API.
3. JMockit
JMockit is another advanced framework that can mock static methods along with private and final methods. It is powerful but comes with its complexity.
Setting Up JMockit
You can include JMockit with the following dependency:
<dependency>
<groupId>org.jmockit</groupId>
<artifactId>jmockit</artifactId>
<version>1.51</version> <!-- or latest -->
<scope>test</scope>
</dependency>
Code Example with JMockit
Here's an example of using JMockit to mock a static method:
import mockit.Expectations;
import mockit.Mocked;
import org.junit.Test;
public class JMockitStaticMethodTest {
@Mocked
StaticClass staticClass;
@Test
public void testStaticMethod() {
new Expectations() {{
StaticClass.staticMethod(); result = "Mocked Result";
}};
// Act
String result = StaticClass.staticMethod();
// Assert
assertEquals("Mocked Result", result);
}
}
Why Use JMockit?
JMockit is powerful in scenarios where extensive mocking is required, but its learning curve can be steeper. It is ideal for more advanced users and those looking to test applications with complex interactions.
Choosing the Right Framework
The choice of mocking framework largely depends on your project’s requirements and your team’s familiarity with the tools. Here is a quick comparison to guide your decision:
| Framework | Pros | Cons | |-------------------|----------------------------------------------------|------------------------------------------| | PowerMock | Handles static methods and constructors, powerful | Heavier footprint, additional learning curve | | Mockito with Inline Mocking | Lightweight, integrates well with existing code | Limited to version 3.4.0 or later | | JMockit | Extensive mocking capabilities, robust | Steeper learning curve |
Closing the Chapter
Mocking static methods can be a daunting task, but with the right tools, it becomes manageable. PowerMock, Mockito's inline mocking, and JMockit offer distinct advantages depending on your specific requirements.
As best practices, always strive to minimize the usage of static methods where possible. Favor dependency injection to allow for better testability in your code. However, when you do encounter static methods in your projects, choose a mocking framework that aligns with your team’s skills and project demands.
For further reading, you can explore the official documentation of each framework:
By understanding the strengths and weaknesses of these mocking frameworks, you can make informed decisions that enhance the maintainability and testability of your Java applications. Happy coding!